3 easy methods to include JavaScript in your HTML file
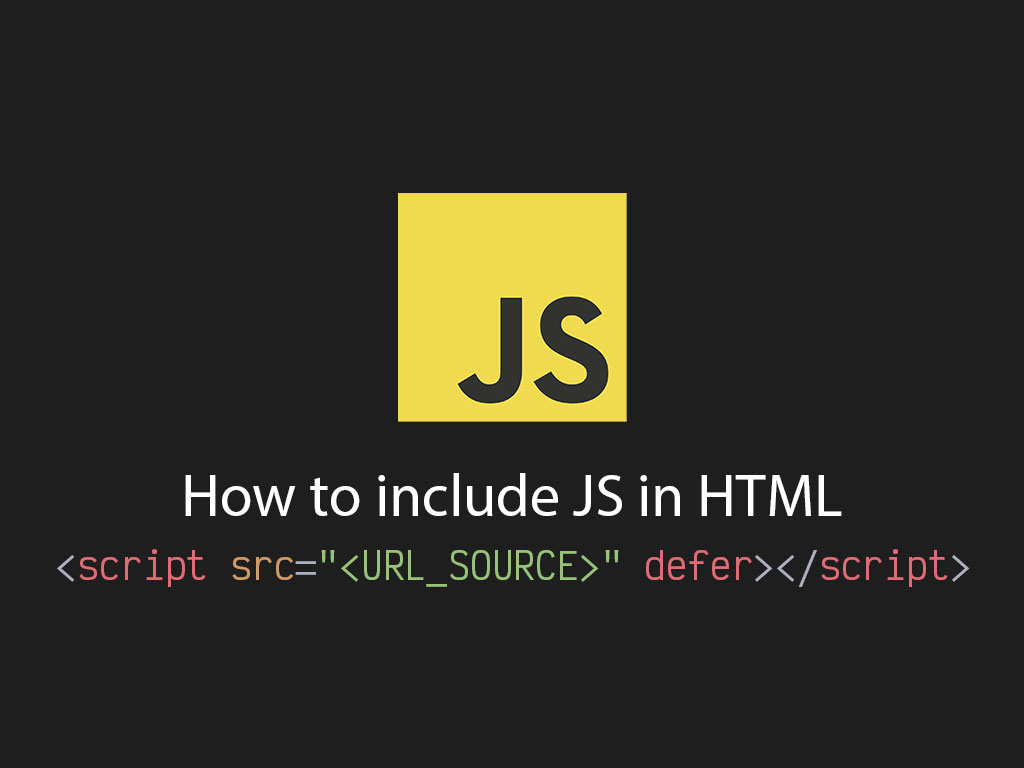
Are you stuck trying to add JavaScript to your HTML file?
Here’s a cheat sheet that shows you 3 easy methods to include JavaScript in your HTML file.
Add JavaScript within HTML script tags
This is one of the easiest ways to just implement JavaScript in your HTML.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>JavaScript inside script tag</title>
</head>
<body>
<script>
document.body.append("Hello World");
</script>
</body>
</html>
This method makes it easy to start writing JavaScript in your HTML file, and it also makes it easier to maintain instead of managing 100’s of files.
But this method comes at a cost worth knowing.
Problem: Blocking nature of script tags
In HTML, every line code is synchronous and gets executed 1 line at a time.
This could mean that script tags may delay the rendering of the UI in the page. Slow speed times could mean a bad user experience for your users.
Here are a few methods to prevent delaying rendering times while using the script tag.
Add script tag at the bottom
One method to avoid delaying the rendering of the UI is by adding the script tag at the bottom before the closing </body>
tag.
<body>
<h3>hi</h3>
<!-- JavaScript last -->
<script>
document.body.append("Hello World");
</script>
</body>
Since your script tag is at the bottom of the page, it tells the users browser that this block should be the last thing to execute.
Use defer property in script tag
Another method to avoid delaying the rendering of the UI is by adding the defer attribute
in the script tag.
<head>
<script defer>
document.body.append('Hello there!');
</script>
</head>
<body>
<h3>hi</h3>
</body>
The defer
attribute lets the browser know not to run this script tag until after the has loaded.
Add JavaScript inline
This method is considered to be very 90’s style. It’s a practice that is NOT recommended by modern code standards.
But it does work, so here’s an example:
<input type="button" value="Click" onclick="alert('This is inline!')" />
Again, avoid this method at all cost.
Include external JavaScript file
I highly recommend from all 3 options.
<head>
<script src="<URL_TO_FILE>" defer></script>
</head>
<body>
<h1>hi there</h1>
</body>
The script tag accepts an attribute called src
.
The src
attribute specifies the URL of an external script file.
This is helpful when you want to run the same JavaScript code in multiple pages. It can help to NOT create duplicate code.
Another pro to this method is that it can be cached. When a user comes to the site for the first time, it will get served the normal load times. The browser will then store that asset in its cache system.
When the same user visits the site again, that JavaScript file will get served instantly to the user. Faster load times, better user experience.
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!