A complete guide how to segment a URL with JavaScript
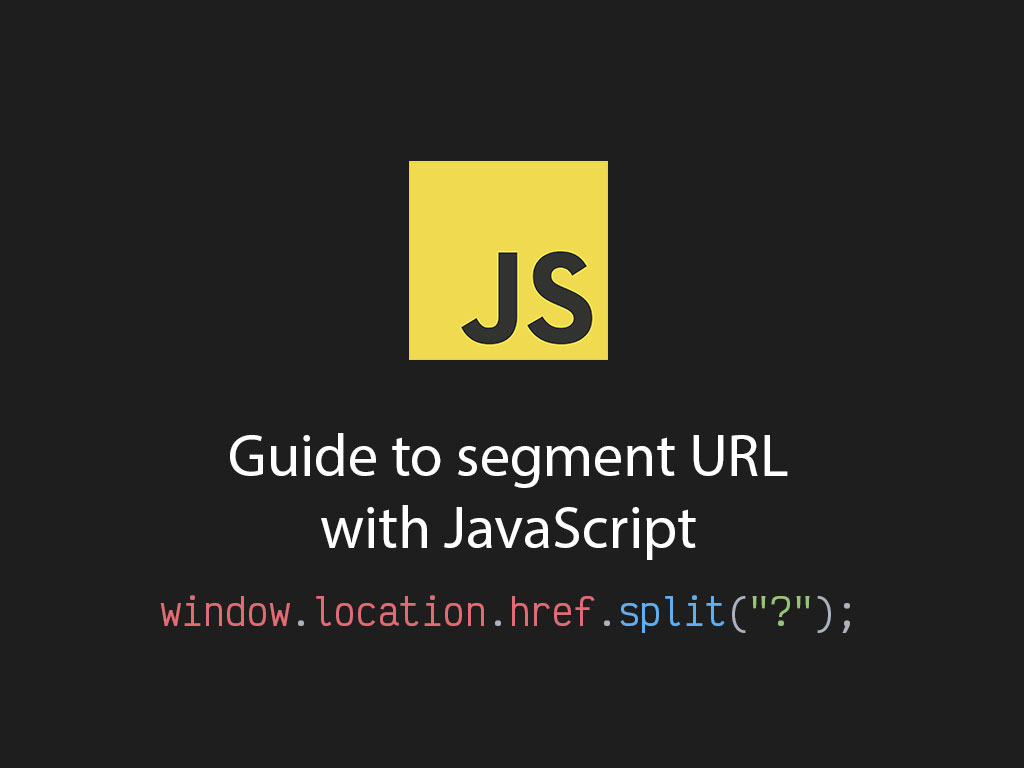
Every now and then, I find myself needing to segment a URL to reformat or just pluck some data out of the URL.
I’m hoping this article solves a number of those URL segment questions for you, as it does for me.
Let’s dive into how I solve these problems.
Get the last part of a URL
const slug = window.location.href.substring(
window.location.href.lastIndexOf("/") + 1
);
This method works well ONLY if you have a URL without a query string.
This method will return the last part of a URL path WITH the query string.
Get the last part of a URL without query string
If you want to get the last part of a URL without the query string, just use the property name window.location.pathname
instead of window.location.href
.
const slug = window.location.pathname.substring(
window.location.pathname.lastIndexOf("/") + 1
);
Get URL without query parameters in general
Sometimes, you just need the full URL with the protocol, but without query parameters. Here’s how that is done.
const fullUrlWithoutParams =
window.location.protocol +
"//" +
window.location.host +
window.location.pathname;
In the window.location
object, you can pick out the protocol (https or http) and concatenate it with the host (www.domain.com) and the pathname.
If you want to do this in ES6 format, you can use the interpolation technique.
const { protocol, host, pathname } = window.location;
const fullUrlWithoutParams = `${protocol}//${host}${pathname}`;
How to get URL query parameter
If IE support is not your concern, you can use the new URL()
object that comes with JavaScript out of the box.
Let’s say you have this URL: https://domain.com/?foo=woosah
And you want to get the parameter value of foo
, here’s how to do it with the new URL()
object in JavaScript.
const url = new URL(window.location.href);
Once you’ve created a new instance, you’ll have access to the searchParams
methods.
Here’s the full code.
const url = new URL(window.location.href)
const paramValue = url.searchParams.get('foo')
console.log(paramValue); // woosah
If you want something that is better supported for IE, you can google the thousands of functions to parse a query string, or you can use an NPM module such as query-string.
They may make it easy to
const queryString = require('query-string');
const parsed = queryString.parse(location.search);
console.log(parsed); // { foo: woosah }
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!