An Easy Comprehensive Guide To Bluehost Affiliate API
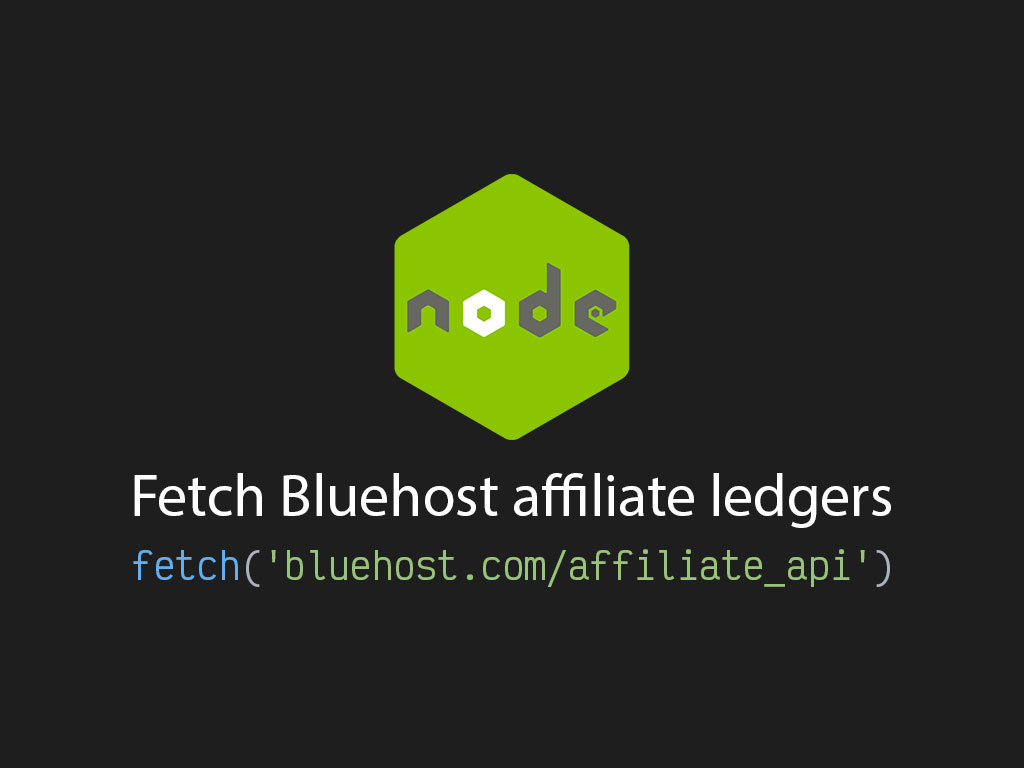
Are you looking to build a custom data visualization application with fancy and fun charts from your Bluehost affiliate stats?
I know I am. The problem I’m facing is that the Bluehost Affiliate API guide is missing information like what does an implementation of the code look like, and what will the response object look like.
This guide aims to give you a full scope of how to make calls, and what to expect from Bluehost Affiliate API.
Disclaimer: This article contains affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the tools I fully recommend when it comes to hosting a website.
Getting started
Before we get started making calls and what not, I want to preface that you will need some basic knowledge of JavaScript, since I will be using with my code examples.
If you’re not familiar with it, and would like to start learning, head on over to, “How to start JavaScript“.
Second, you’re going to need to make an affiliate account with Bluehost to be able to make calls.
Click here to create an affiliate account.
Once you’ve been accepted and have access to the dashboard, you can go to settings menu item and then click on the api sub menu item.
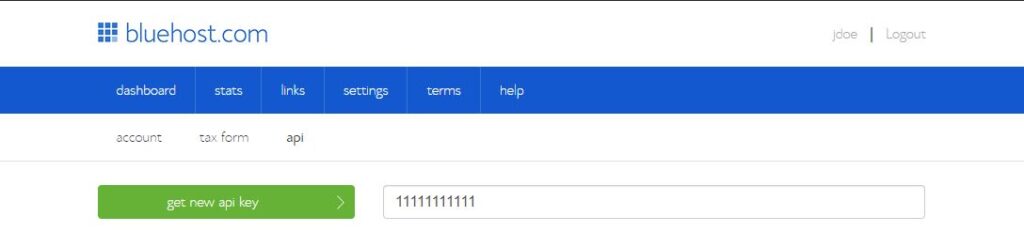
Protect your Bluehost API key
If the API key is going to be used locally you have nothing to worry about.
But if your project is going to a server or going to a Git service like Gitlab or Github, make sure to not track it with Git.
Save your API key in an environment variable file so they’re not flying everywhere.
Bluehost API response object
What makes this API simple and easy to use is that all the endpoints return the same response object.
{
"headers": [
"date_entry",
"domain",
"type",
"amount",
"term",
"package_cost",
"signup_ip",
"tracking_code",
"referer"
],
"rows": [
{
"tracking_code": "default",
"term": "term-lenght",
"signup_ip": "some.ip.address",
"domain": "customer.domain.com",
"amount": "commision-amount",
"package_cost": "price-of-the-package",
"type": "signup",
"date_entry": "date-customer-purchase",
"referer": "-"
}
]
}
The headers
property contains a list of properties and values that you will find in every entry.
[
"date_entry",
"domain",
"type",
"amount",
"term",
"package_cost",
"signup_ip",
"tracking_code",
"referer"
]
The rows
property is the array of all the entries that have been successfully submitted.
[
{
"tracking_code": "default",
"term": "term-lenght",
"signup_ip": "some.ip.address",
"domain": "customer.domain.com",
"amount": "commision-amount",
"package_cost": "price-of-the-package",
"type": "signup",
"date_entry": "date-customer-purchase",
"referer": "-"
}
// ... more items
]
Get all your affiliate sells for this current year
Here’s the URL you’re going to need to ping to get the current year affiliate sells from Bluehost:
https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_ytd/json/${generated_api_key}
This is how it looks like calling that API endpoint with JavaScript.
try {
const res = await fetch('https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_ytd/json/${generated_api_key}');
console.log(res);
} catch(e) {
console.log(e) // print error
}
Here are some other Bluehost API endpoint examples.
Get all your affiliate sells from last year
Here’s the URL you’re going to need to ping to get last years affiliate sells from Bluehost:
https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_lastyear/json/${generated_api_key}
This is how it looks like calling that API endpoint with JavaScript.
try {
const res = await fetch('https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_lastyear/json/${generated_api_key}');
console.log(res);
} catch(e) {
console.log(e) // print error
}
Get all your affiliate sells from current month
Here’s the URL you’re going to need to ping to get the current month affiliate sells from Bluehost:
https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_currentmonth/json/${generated_api_key}
This is how it looks like calling that API endpoint with JavaScript.
try {
const res = await fetch('https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_currentmonth/json/${generated_api_key}');
console.log(res);
} catch(e) {
console.log(e) // print error
}
Get all your affiliate sells from the last month
Here’s the URL you’re going to need to ping to get the previous month affiliate sells from Bluehost:
https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_prevmonth/json/${generated_api_key}
This is how it looks like calling that API endpoint with JavaScript.
try {
const res = await fetch('https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_prevmonth/json/${generated_api_key}');
console.log(res);
} catch(e) {
console.log(e) // print error
}
Get all your affiliate sells from the current quarter
Here’s the URL you’re going to need to ping to get the current quarter affiliate sells from Bluehost:
https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_currentquarter/json/${generated_api_key}
This is how it looks like calling that API endpoint with JavaScript.
try {
const res = await fetch('https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_currentquarter/json/${generated_api_key}');
console.log(res);
} catch(e) {
console.log(e) // print error
}
Get all your affiliate sells from the previous quarter
Here’s the URL you’re going to need to ping to get the previous quarter affiliate sells from Bluehost:
https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_prevquarter/json/${generated_api_key}
This is how it looks like calling that API endpoint with JavaScript.
try {
const res = await fetch('https://www.bluehost.com/cgi/affiliate_api/${username}/ledger_prevquarter/json/${generated_api_key}');
console.log(res);
} catch(e) {
console.log(e) // print error
}
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!