How to clear localStorage when browser/tab is closing
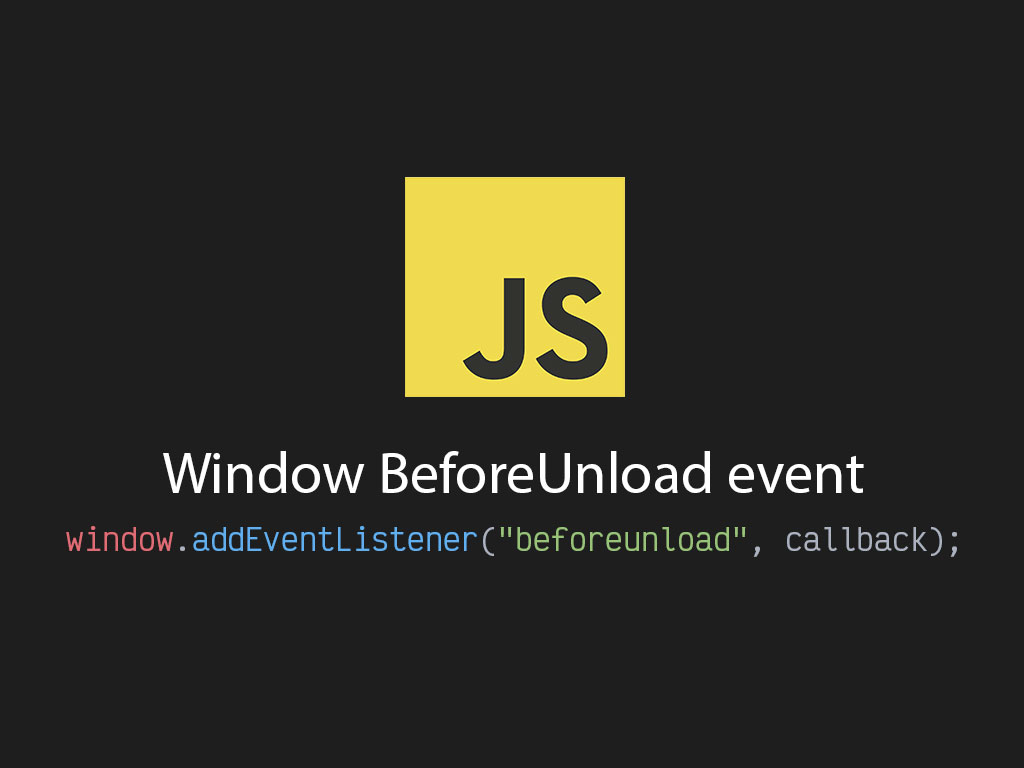
Every now and then I store simple data onto the window.localStorage
, and I want to clear it right after.
The answer to solve this problem is to use window.onbeforeunload
event listener.
Here’s a simple example. Let’s say that we have a sign in form.
The user clicks sign in.
const signIn = () => localStorage.setItem('isAuth', 'true');
Great! Now you can reference the browser local storage to check if the user is authenticated.
localStorage.getItem('isAuth') === 'true' // true
Now let’s add a curve ball! Everytime the user comes back to the site, I want him/her to sign in again.
To do that, I typically write this:
window.onload = () => {
// Clear localStorage
if (localStorage.getItem('isAuth') === 'true') {
localStorage.removeItem('isAuth');
// Show them the sign in form
}
};
The method above works. But it may cause some weird issues.
It may flicker the authenticated view for a second. Or now you may have extra boilerplate code to check when the webpage loads.
There’s a cleaner way!
Use window.onbeforeunload
There is a JavaScript event called beforeunload
. This event gets triggered when a window or browser tab is about to close.
In the code above, I’m going to switch from window.onload
to window.onbeforeunload
.
window.onbeforeunload = () => {
localStorage.removeItem('isAuth');
}
This event is supported in all major browsers. Even IE 4, if you want to go old school.
You may also use the window.addEventListener()
method.
window.addEventListener("beforeunload", () => localStorage.removeItem('isAuth'));
It’s really neat to know that there’s an event listener for a closing tab or window.
But for the use-case above, theirs a better and simpler solution; and that is window.sessionStorage
.
Best solution: sessionStorage
window.sessionStorage
is similar to window.localStorage
.
// Save data to sessionStorage
sessionStorage.setItem('isAuth', 'true');
// Get saved data from sessionStorage
let data = sessionStorage.getItem('isAuth');
// Remove saved data from sessionStorage
sessionStorage.removeItem('isAuth');
// Remove all saved data from sessionStorage
sessionStorage.clear();
The only difference is that, window.sessionStorage
automatically clears the data after closing window or tab.
So you don’t need to add an event listener!
This feature is supported in all major browsers and IE8+.
Hey, you made this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!