How to define a single or multiple return type for a function in TypeScript
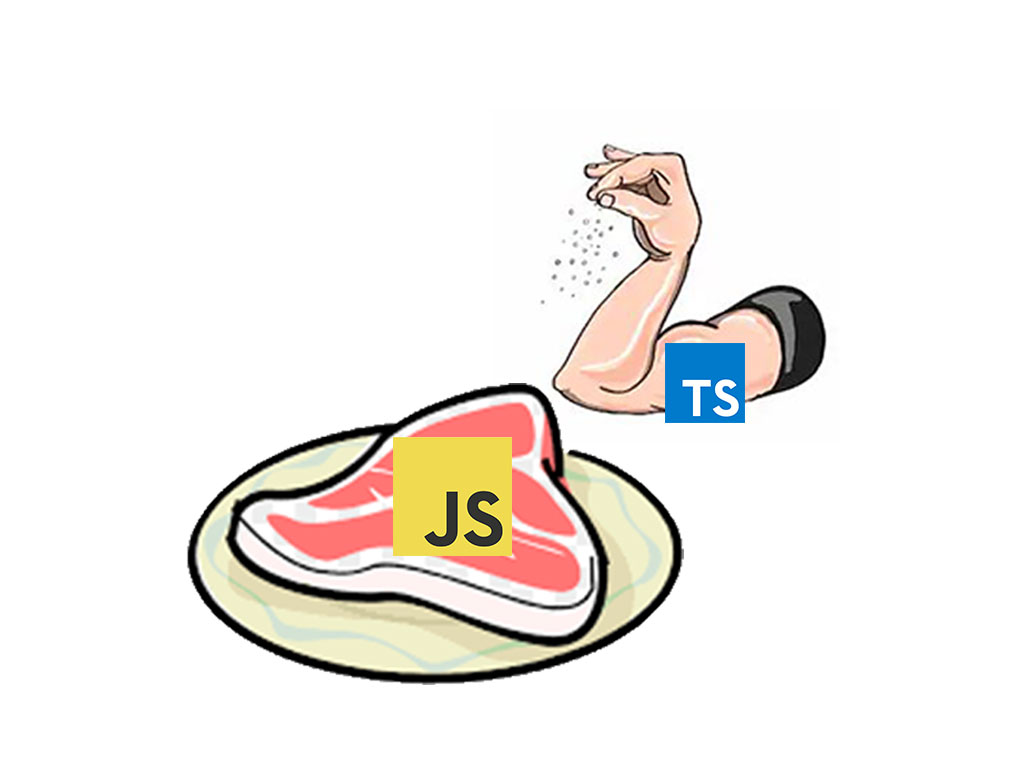
Sometimes, reading through the TypeScript documentation may be a bit difficult.
And all you want to do is know how to type your functions.
In this article I discuss how to type your regular functions, arrow functions, and how to define multiple data types for a function.
Defining return type of a function
Returning the type value from a function is pretty simple.
All you need to do is add a :
between the closing parenthesis of the signature method ,and the opening curly bracket.
After the colon, write the data type the function will return. This may either be a string, number, boolean, void, or and many more.
interface PersonProps {
name: string;
age: number;
isCool: boolean;
}
// Return type string
function setName(str: string): string {
return str;
}
// Return type number
function setAge(num: number): number {
return num;
}
// Return type boolean
function isCool(): boolean {
return true;
}
// Return type of an object
function person(): PersonProps {
return {
name: setName('John Doe'),
age: setAge(31),
isCool: isCool(),
}
}
// Return type void
function greet(): void {
console.log('Hi there!');
}
Defining return type for arrow function
The same rule applies to arrow function.
// Return type string
const setName = (name: string): string => name;
// Return type number
const setAge = (num: number): number => num;
// Return type boolean
const isCool = (): boolean => true;
// Return type void
const greet = (): void => console.log('Hi there!');
Return multiple data types
To allow a function to return multiple data types, you simply add a pipe after a data type.
interface PersonProps {
id: number;
name: string;
likes: {
dogs: boolean;
cats: boolean;
}
}
// This function may return a PersonProp object type or null
function getPersonById(id: number): PersonProps | null {
const person = {
id: 1,
name: 'John Doe',
likes: {
dogs: true,
cats: false,
}
};
if (id !== person.id) {
return null;
}
return person;
};
const person1: PersonProps | null = getPersonById(); // null
// { id: 1, name: 'John Doe', likes: { dogs: true, cats: false, } }
const person2: PersonProps | null = getPersonById(1);
In the example above, PersonProps | null
, I added a pipe after PersonProps
Related articles:
I like to tweet about TypeScript and post helpful code snippets. Follow me there if you would like some too!