An EASY starting guide to Enums in TypeScript
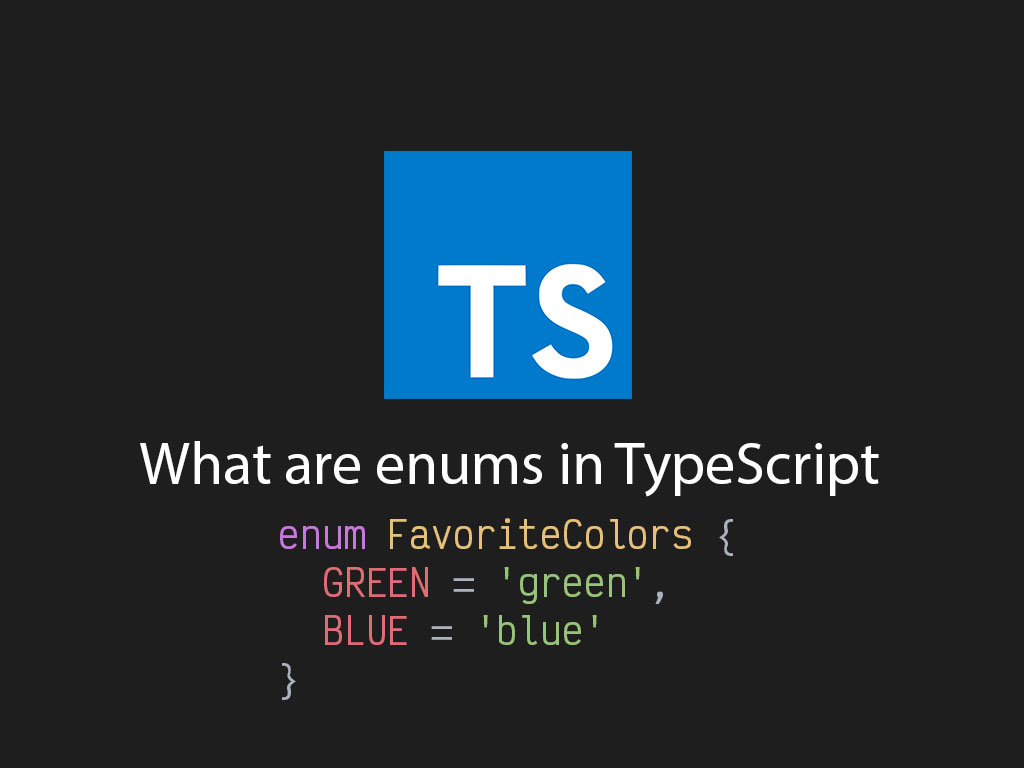
Lately, I’ve been seeing the keyword Enum in TypeScript code. I’ve never used Enums, what could that an Enum possibly mean?
Enums are really just a collection (object format) of constants.
Before I explain and show an example of an Enum in TypeScript, let’s go over a plain constant in JavaScript.
A constant in JavaScript
A constant in JavaScript are variables that do not change in value after they’ve been initiated.
const pizzaType = "pepperoni";
If you would like to learn more about JavaScript variables, you can visit this page, “JavaScript Variables“.
Now let’s define an Enum.
The definition of Enum
An enum
in TypeScript is a collection of constants. That’s it.
Here’s the syntax example:
enum Colors {
RED = 'red',
WHITE = 'white',
BLUE = 'blue'
}
TypeScript will grab the enum
that was created and allow you to access the properties like an object.
console.log(Colors.RED) // red
The correct way to define an Enum is by using camelcase
The standard that I’ve seen engineers write enums is by using camelcase syntax style.
enum FavoriteColors {}
And all the properties inside are uppercase.
enum FavoriteColors {
GREEN = 'green',
BLUE = 'blue'
}
This special keyword only exists in TypeScript and NOT in vanilla JavaScript.
Is there a vanilla JavaScript method to write an enum
style? Yes there is!
JavaScript does not have enums but it has Object.freeze()
Like I said above, vanilla JavaScript does not support enums. But you can imitate the style with Object.freeze()
.
const Pizzas = Object.freeze({
PEPPERONI: true,
CHEESE: true,
PINEAPPLE: false,
});
Object.freeze()
allows you to create an object that is immutable, which means no property values can be changed after the initiation.
console.log(Pizzas.PEPPERONI) // true
Const Enum vs Enum
Another hot topic is the debate of using enum
vs const enum
. What’s the difference? Which should you use?
Let’s look at a two examples of a normal enum
, and what the output looks like.
enum Colors {
RED = 'red',
WHITE = 'white',
BLUE = 'blue'
}
enum Colors2 {
RED,
WHITE,
BLUE
}
Here’s the output of the code above:
"use strict";
// Colors enum output
var Colors;
(function (Colors) {
Colors["RED"] = "red";
Colors["WHITE"] = "white";
Colors["BLUE"] = "blue";
})(Colors || (Colors = {}));
// Color2 enum output
var Colors2;
(function (Colors2) {
Colors2[Colors2["RED"] = 0] = "RED";
Colors2[Colors2["WHITE"] = 1] = "WHITE";
Colors2[Colors2["BLUE"] = 2] = "BLUE";
})(Colors2 || (Colors2 = {}));
One to note, is that the JS output of an enum
is some what unpredictable.
Now let’s look at the difference of a const enum
. We’re going to grab one of the enum
above, and just add the keyword const
in front of it.
const enum Colors {
RED = 'red',
WHITE = 'white',
BLUE = 'blue'
}
Here’s the JS output of a const enum
:
"use strict";
The result is that none of that code makes to the compiled code!
Now, the question is “Should use const enum or enum”?
TypeScript has noted, that const enums
should be avoided on their documentation. So try to use regular enum
as much as possible.
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about TypeScript and post helpful code snippets. Follow me there if you would like some too!