How to get the current price of Bitcoin with Next.js and Kraken API
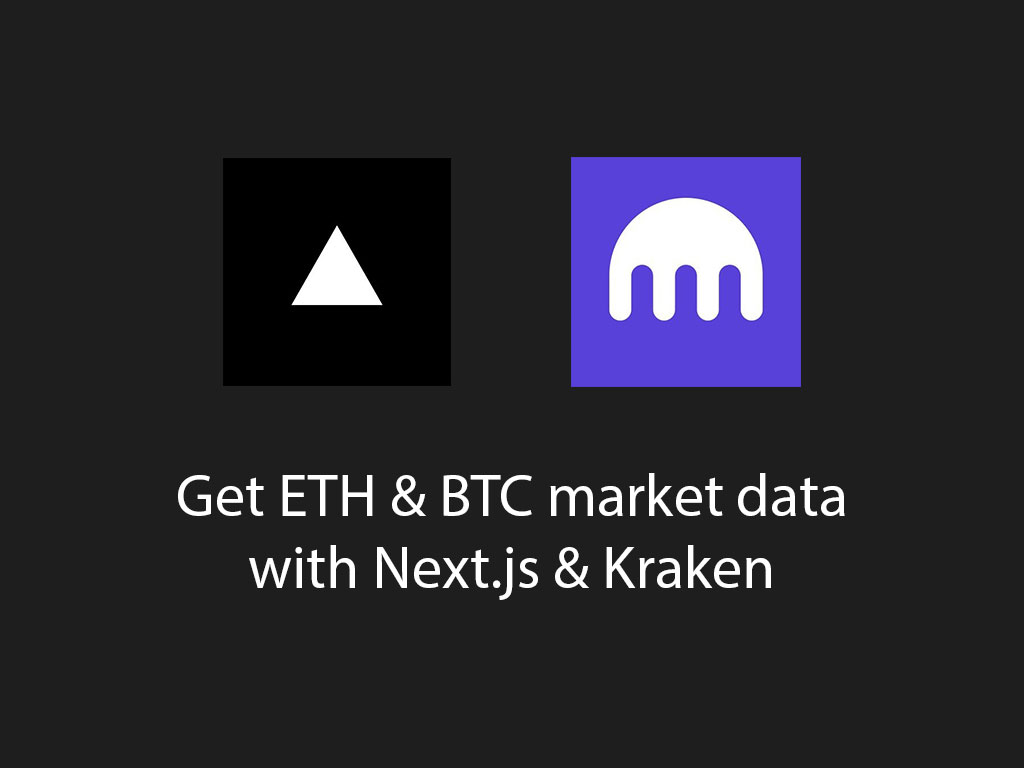
I’m a big fan of cryptocurrency, especially Bitcoin and Ethereum. It got me wondering, “is there an open API for the market data of cryptocurrencies?”
The answer there is! I found out that the cryptocurrency trading service that I use, Kraken, provides an API that gives you access to public market data about a cryptocurrency such as Ethereum and Bitcoin. And it has other neat private API’s for funding and trading within your Kraken account.
But in today’s article will focus on creating a Next.js application that fetches public data from Kraken API and render the data on the page.
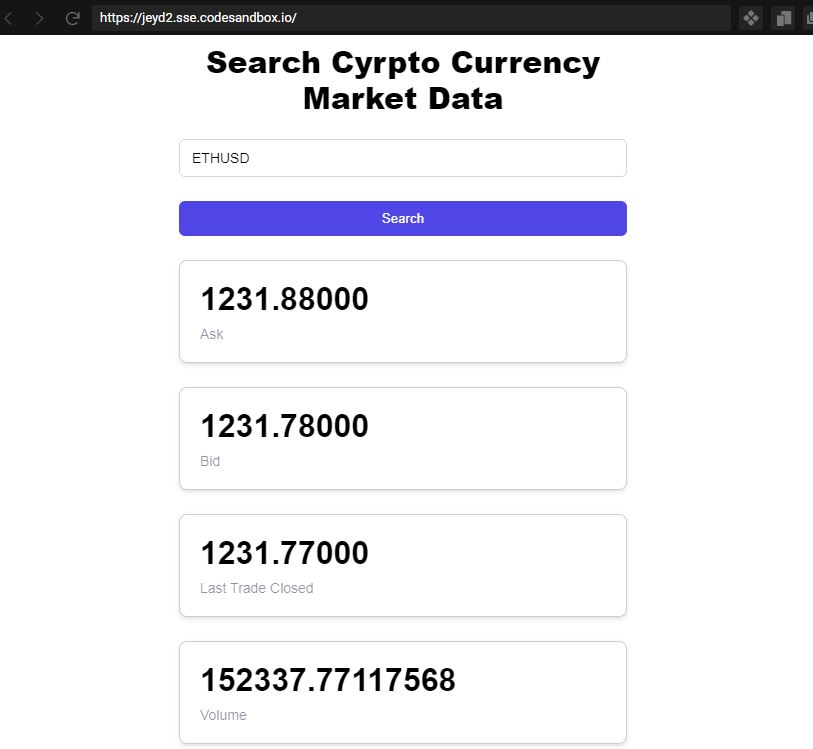
The full source code can be found by clicking here.
First, if you’re looking to get started with trading cryptocurrency, and you’re looking for a service that is secure, and has great customer service, you might want to look into Kraken. Kraken has been rated as one top 5 trading exchange platforms. They’re constantly improving their security to make sure you have a safe and reliable user experience. On top of the that, they are one of the trading services that provides a wide variety of cryptocurrency and supports a wide variety of fiat currency.
Get started with Kraken.
Disclaimer: This article contains affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. This is merely a service I fully recommend when trading cryptocurrency.
Let’s get started!
Step 1: Create a Kraken account (Optional)
Create an account with Kraken, if you want to do more than just getting public market data from a cryptocurrency.
Then you’ll have to create a Kraken account so you can generate an API key and a private key.
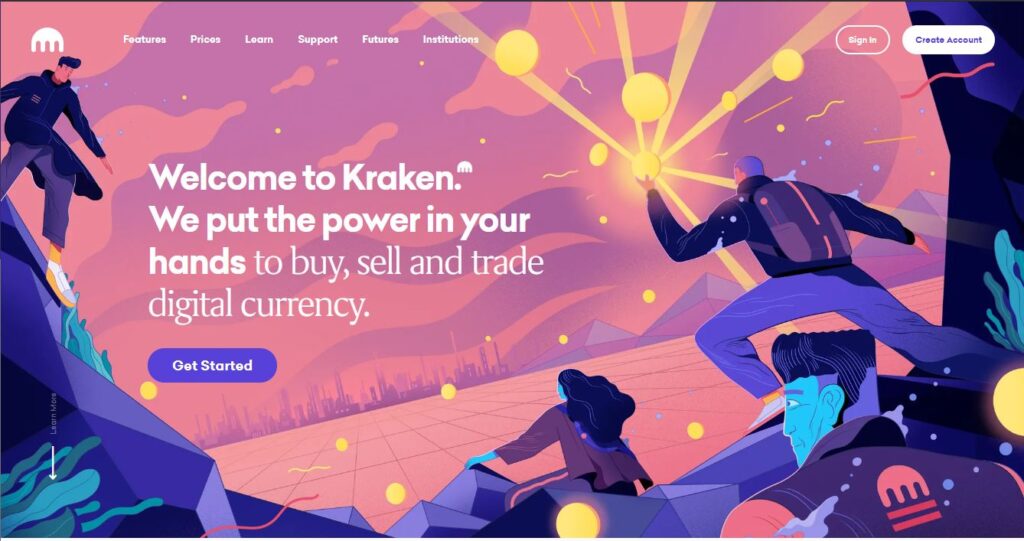
Here’s a list of features you can do with Kraken API:
- Get ticker information (public)
- Get recent trades (public)
- Get account user data (private)
- Trade cryptocurrency (private)
- Fund your account (private)
Click here to get started with cryptocurrency trading with Kraken.
Step 2: Install dependencies and setup package.json file
Installing dependencies is always the easiest part of a project. Copy the command below and enter it in your terminal.
npm i -S next react react-dom isomorphic-fetch
Your package.json file should have been updated.
We’ve installed the following libraries to our project:
next
– Our React frameworkreact
– The UI libraryreact-dom
– Attaching React to the DOMisomorphic-fetch
– Will help you make requests to Kraken API.
Here’s what the package.json file should look like:
{
"name": "nextjs-sandbox",
"version": "1.0.0",
"scripts": {
"dev": "next",
"build": "next build",
"start": "next start"
},
"dependencies": {
"isomorphic-fetch": "3.0.0",
"next": "latest",
"react": "^16.7.0",
"react-dom": "^16.7.0"
}
}
Step 3: Import libraries and setup Kraken labels
In our Next.js project, I’m going to do all the code within the index.js file inside the pages directory.
import { useState } from "react";
import Head from "next/head";
import fetch from "isomorphic-fetch";
const KRAKEN_API_URL = "https://api.kraken.com/0/public/Ticker";
const KRAKEN_DATA_LABELS = Object.freeze({
a: "Ask",
b: "Bid",
c: "Last Trade Closed",
v: "Volume",
l: "Low",
h: "High",
o: "Today Opening Price"
});
The first 3 lines of the code snippet above is just importing some utilities that will be used in the project.
The important part of the code above is the KRAKEN_API_URL
variable, and the KRAKEN_DATA_LABELS
JavaScript object.
KRAKEN_API_URL
holds the Kraken endpoint to fetch the cryptocurrency ticker market data.
https://api.kraken.com/0/public/Ticker
KRAKEN_DATA_LABELS
is an object that holds human readable labels. The Kraken API returns a collection of letters, but each letter has a meaning. It’s just not human friendly.
a = ask
b = bid
c = last trade closed
v = volume
p = volume weighted average price
t = number of trades
l = low
h = high
o = today's opening price
Step 4: Create custom React hook to find ticker market data
In the index.js file, I will create a custom React hook called useFindTicker()
.
const useFindTicker = () => {
// Holds value for search form
const [symbol, setSymbol] = useState("");
// Holds data value of cryptocurrency market data
const [data, setData] = useState(null);
// Holds error messages from KRAKEN API
const [error, setError] = useState(null);
return {
setSymbol,
data,
error,
find: async () => {
try {
// No need to make a request if symbol value is empty.
if (symbol.trim().length < 1) {
console.log("Symbol is empty");
return;
}
// Fetch ticker data from Kraken API
const res = await fetch(`${KRAKEN_API_URL}?pair=${symbol.trim()}`);
const { error, result } = await res.json();
// IF any errors, set error to state
if (error.length > 0) {
setError(error);
return;
}
// Set ticker data to state
setData(
Object.keys(result).reduce(
(pv, cv) => ({
...result[cv]
}),
{}
)
);
return;
} catch (e) {
console.error(e);
}
}
};
};
The purpose of this custom Reach hook is to store the symbol value from the search form, and the data or error messages being returned from the Kraken API.
Let’s do a quick breakdown of the code.
// Holds value for search form
const [symbol, setSymbol] = useState("");
// Holds data value of cryptocurrency market data
const [data, setData] = useState(null);
// Holds error messages from KRAKEN API
const [error, setError] = useState(null)
symbol
– Is the React state variable for the Cryptocurrency symbol.
For example if I search ETHUSD which is the symbol for Kraken to search for Ethereum with USD currency values.
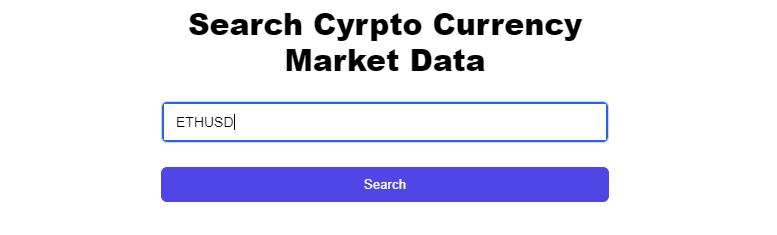
The value ETHUSD will be stored in the variable symbol
.
The data
React state variable holds any result returned from the Kraken API.
The error
React state variable holds any error messages returned from the Kraken API.
The next important piece of code is the find()
function.
find: async () => {
try {
// No need to make a request if symbol value is empty.
if (symbol.trim().length < 1) {
console.log("Symbol is empty");
return;
}
// Fetch ticker data from Kraken API
const res = await fetch(`${KRAKEN_API_URL}?pair=${symbol.trim()}`);
const { error, result } = await res.json();
// IF any errors, set error to state
if (error.length > 0) {
setError(error);
return;
}
// Set ticker data to state
setData(
Object.keys(result).reduce(
(pv, cv) => ({
...result[cv]
}),
{}
)
);
return;
} catch (e) {
console.error(e);
}
}
The find()
function does the following:
- Makes sure that the React state variable
symbol
is not empty. If it is empty, it does not proceed. - Makes a request to Kraken ticker endpoint
- Checks if any errors came back. If so, it updates the
error
React state variable and ends the process there. - If endpoint returns the result, update the React state variable,
data
.
Step 5: Create React component to render ticker market data
The final part is to create the React search form and the cards to show the results.
Creating the Cryptocurrency React form
export default () => {
const { find, setSymbol, data, error } = useFindTicker();
// On submit handler
const handleSubmit = () => find();
// On input change handler
const handleChange = (e) => setSymbol(e.target.value);
return (
<>
<Head>
<link rel="stylesheet" href="/styles.css" />
</Head>
<div className="container">
<h2 className="headline">Search Cyrpto Currency Market Data</h2>
<div className="rounded-form">
<input type="text" placeholder="ETHUSD" onChange={handleChange} />
</div>
<div className="action-box">
<button onClick={handleSubmit}>Search</button>
</div>
</div>
</>
);
};
In the initial part of the React component, I’m using the custom React hook that was created, useFindTicker()
and I’m destructuring it to access the find, setSymbol
, data
, and error
variable.
I’ve also created a handleSubmit()
handler function that uses the find()
from the custom hook that was created.
The handleChange()
handler function uses setSymbol
to save each character entered in the search field to the React state variable symbol
.
The rest is fairly simple. I’m just creating a JSX/HTML to render the form.
I’ve also attached the handleSubmit()
function to the search button and the handleChange()
function to the input element
.
Display data or error message
The final step is to output the data from the Kraken API endpoint.
export default () => {
// ... useFindTicker() and handler functions
return (
<>
{/* ... search form code */}
{(error && <h1 className="headline">{error}</h1>) ||
(data && (
<div className="container result">
{Object.keys(data).map((dataKey, i) => (
<div key={`${dataKey}-${i}`} className="card">
<h3 className="card-value">
{Array.isArray(data[dataKey])
? data[dataKey][0]
: data[dataKey]}
</h3>
<p className="card-label">{KRAKEN_DATA_LABELS[dataKey]}</p>
</div>
))}
</div>
))}
</>
);
};
Step 6: Run it
That’s pretty much it. Go ahead and run your program.
npm run dev
Source code
If you would like access to the source code. Click here.
First, if you’re looking to get started with trading cryptocurrency, and you’re looking for a service that is secure, and has great customer service, you might want to look into Kraken. Kraken has been rated as one top 5 trading exchange platforms. They’re constantly improving their security to make sure you have a safe and reliable user experience. On top of the that, they are one of the trading services that provides a wide variety of cryptocurrency and supports a wide variety of fiat currency.
Get started with Kraken.
Disclaimer: This article contains affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. This is merely a service I fully recommend when trading cryptocurrency.
I like to tweet about Next.js and post helpful code snippets. Follow me there if you would like some too!