How to access getInitialProps with a class or function component in NextJS
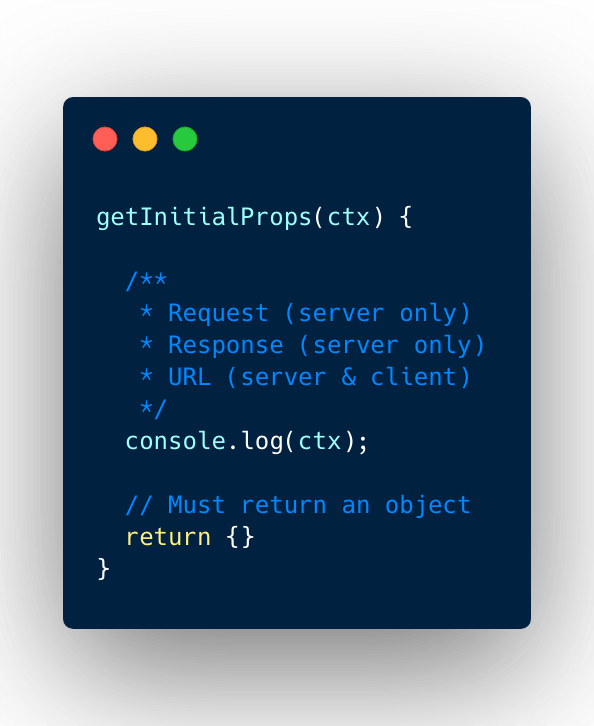
In today’s article we’re going to cover how to fetch data on the server side, and passing it down to a React component using NextJS.
To accomplish this task, will need to use a static method that NextJS provides called, getInitialProps()
.
What is getInitialProps()?
That’s a great question.
This methods is like another lifecycle added to a React component, function or class type.
It allows the engineer to do business logic work like, doing some computation, data transformation, and data fetching before the component logic even comes into execution.
This lifecycle, can happen both on client side & server side of the application.
/**
* CTX object
* - req (server-side only)
* - res (server-side only)
* - pathname (server & client side)
* - query (server & client side)
*/
getInitialProps(ctx) {
return {};
}
The nice things about getInitialProps()
is that it passes down an object, called ctx
(context), metadata of the application.
Such as the request, response, pathname, and query object.
The request & response object are only for server-side. The pathname and query object is available for both.
It also requires you to return an object, preferably with data.
But you may return an empty object.
How to use getInitialProps() in a React class component?
If you’re using a React class style component, you may access getInitialProps()
as a static method.
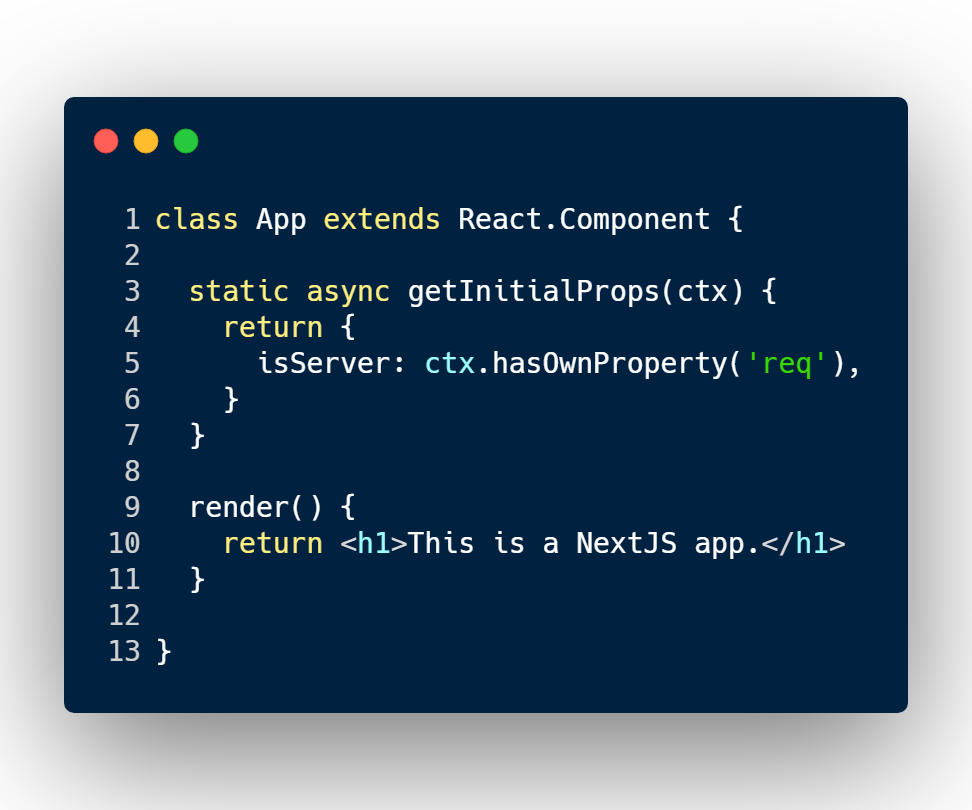
In the example above, I’m also making getInitialProps()
an asynchronous method. This way it, doesn’t block the render of the React component.
How to use getInitialProps() in a React functional/stateless component?
If your trying to do a functional/stateless React component style, you still have access to getInitialProps()
.
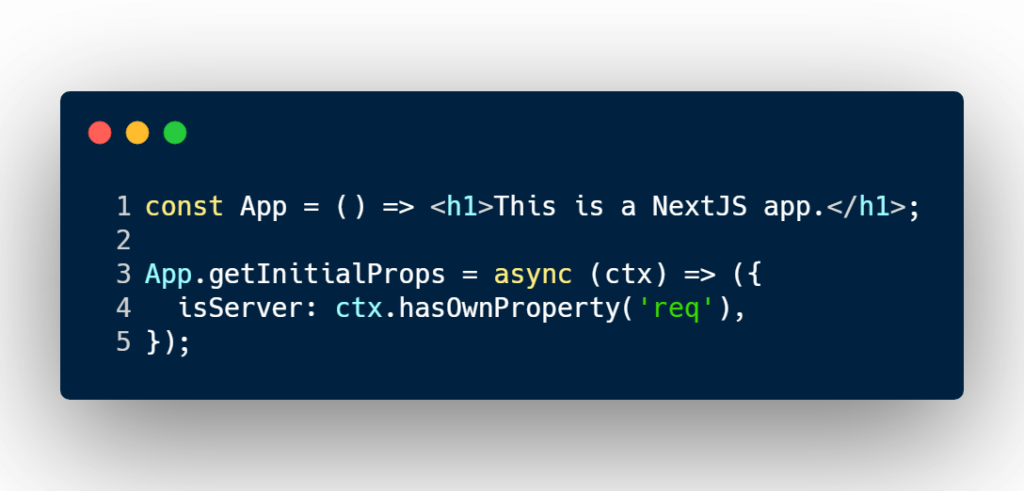
The first step is to define your React component.
On line 3, I’m adding the property getInitialProps
to the App
variable.
You can still make it a asynchronous function by adding the keyword async.
Conclusion
getInitialProps()
is a great lifecycle to do server/client work before a component gets render.
And you still have access to it whether it’s a class or function React component.
I like to tweet about NextJS and post helpful code snippets. Follow me there if you would like some too!