How to add onMouseEnter or onMouseOver in ReactJS
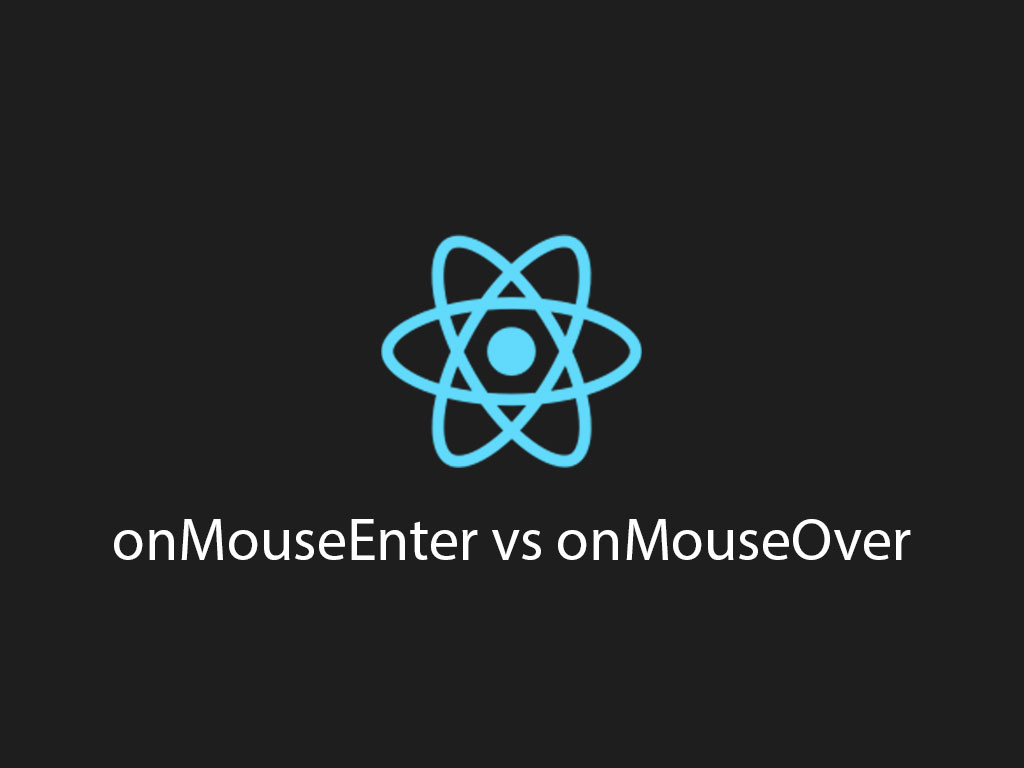
You need an event when a user’s mouse hovers over an HTML element or React component.
So you run into onMouseOver
and onMouseEnter
. They both behave the same, so which one is the right one for you?
First, if you’re looking to become a strong and elite React developer within just 11 modules, you might want to look into Wes Bos, Advanced React course for just $97.00 (30% off). Wouldn’t it be nice to learn how to create end-to-end applications in React to get a higher paying job? Wes Bos, Advanced React course will make you an elite React developer and will teach you the skillset for you to have the confidence to apply for React positions.
Click here to become a strong and elite React developer: Advanced React course.
Disclaimer: The three React course links are affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the course I fully recommend when it comes to becoming a React expert.
The difference between mouseover and mousenter
The difference between mouseenter
and mouseover
is when child elements are put inside the targeted element.
Both of these events behave the same way. When user enters an element they both get triggered.
<style>
#my_div {
height: 120px;
width: 120px;
background-color: #333;
}
</style>
<div id="my_div"></div>
<script>
const divEl = document.querySelector('#my_div');
divEl.addEventListener('mouseenter', () => console.log('Event: mouseenter'));
divEl.addEventListener('mouseover', () => console.log('Event: mouseover'));
</script>
The output should look like this when you hover over the box.
Event: mouseover
Event: mouseenter
Event: mouseover
Event: mouseenter
I’m going to update HTML template and update the styles.
<style>
#my_div {
height: 120px;
width: 120px;
background-color: #333;
display: flex;
align-items: center;
justify-content: center;
}
#my_div > div {
height: 50px;
width: 50px;
background-color: #ccc;
}
</style>
<div id="my_div">
<div></div>
</div>
You should have a smaller box in the center of the #my_div
element.
When you hover all the way to the middle, and hover out of the all the boxes, you should see the following output.
Event: mouseover
Event: mouseenter
Event: mouseover
Event: mouseover
mouseover
gets triggered multiple times. That’s because it gets triggered when the mouse hovers over the selected element OR it’s child elements.
Okay, let’s implement this in a React component now.
React onMouseEnter and onMouseOver examples
The goal in this example is to make .innerBox
appear and disappear when triggering one of these events.
Both will have the same CSS.
.container,
.wrapper {
display: flex;
justify-content: center;
align-items: center;
}
.container {
width: 200px;
height: 200px;
background-color: #ccc;
margin: 60px auto 0;
display: flex;
}
.wrapper {
height: 150px;
width: 150px;
background-color: #666;
}
.innerBox {
width: 80px;
height: 80px;
padding: 8px;
background-color: #222;
display: none;
}
.container.show .innerBox {
display: block;
}
React onMouseEnter example
To add a mouseenter
event listener in React, you must embed our event handler function to onMouseEnter
.
class App extends React.Component {
state = {
showBox: false
};
handleBoxToggle = () => this.setState({ showBox: !this.state.showBox });
render() {
return (
<div
onMouseEnter={this.handleBoxToggle}
className={`container${this.state.showBox ? " show" : ""}`}
>
<div className="wrapper">
<div className="innerBox" />
</div>
</div>
);
}
}
As soon as you hover over the .container
element, it will make .innerBox
appear.
React onMouseOver example
To add a mouseover
event, swap out onMouseEnter
for onMouseOver
.
<div
onMouseOver={this.handleBoxToggle}
className={`container${this.state.showBox ? " show" : ""}`}
>
<div className="wrapper">
<div className="innerBox" />
</div>
</div>
When you hover over .container
, .innerBox
will appear.
But .innerBox
will disappear when you hover the mouse over .wrapper
. Because .wrapper
is an inner child element of .container
, and it will trigger the toggle method.
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!