How to find Webflow API rate limit with JavaScript
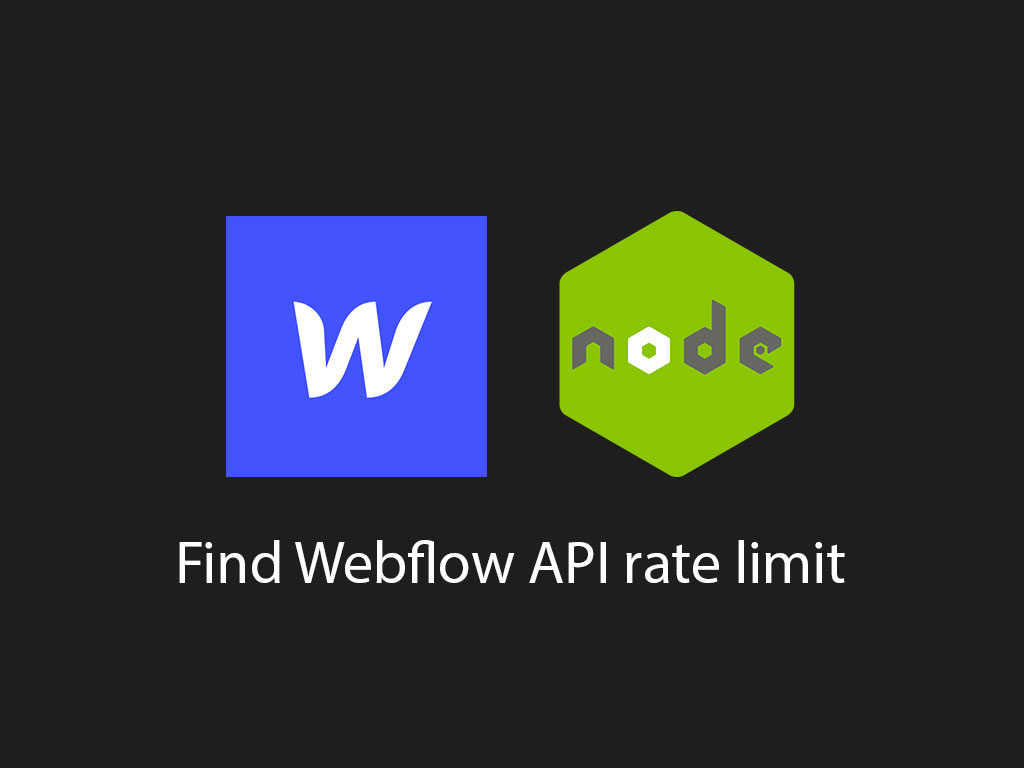
Are you trying to figure out how what your Webflow API rate limit is per minute?
The default for everyone is 60 per minute. But you can find out with this simple Node.JS script.
First, before you start making any API requests to Webflow, you’ll need to get a site up started for as little as $12/month. Webflow makes it really easy build websites without coding.
Disclaimer: The three Webflow links above are affiliate links which provide a small commission to me at no cost to you. These links track your purchase and credit it to this website. Affiliate links are a primary way that I make money from this blog and Webflow is the best visual website builder for new bloggers or ecommerce platforms.
Let’s dive into finding out how to check what your Webflow API rate limit is.
Get Webflow site/project API key
The first thing you’ll need to find is your Webflow website API key, and you’re going to store in a JavaScript variable as such.
const WEBFLOW_API_KEY = '<replace_with_webflow_api_token>';
Once you’ve created your Webflow project, hover over the vertical ellipsis, and click settings.
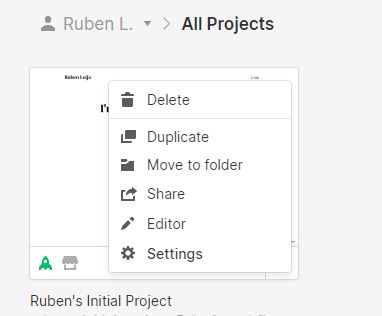
Once you’re in the projects settings, head over to the integration tab.
Scroll down to the section that says API Access and click on the button Generate new API token.
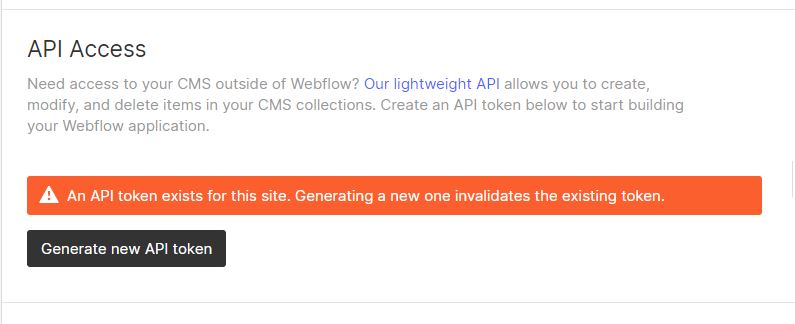
If you forget your API token in the future, you’ll need to recreate a new one.
Great! Now that you have the token lets get into the coding section.
Find out Webflow site/project API rate limit
I’ll be using a module called isomorphic-fetch to make my request to Webflow API.
You may use which ever HTTP request module you like.
const fetch = require('isomorphic-fetch');
const WEBFLOW_API_KEY = '<replace_with_webflow_api_token>';
const WEBFLOW_BASE_URL = 'https://api.webflow.com/info';
async function fetchWebflowSiteInfo() {
try {
const res = await fetch(WEBFLOW_BASE_URL, {
headers: {
Authorization: `Bearer ${WEBFLOW_API_KEY}`,
'accept-version': '1.0.0',
}
});
return await res.json();
} catch(e) {
console.log(e);
}
}
fetchWebflowSiteInfo()
.then(snapshot => console.log(snapshot));
Here’s a sample output:
{
"_id": "55818d58616600637b9a5786",
"createdOn": "2016-10-03T23:12:00.755Z",
"grantType": "authorization_code",
"lastUsed": "2016-10-10T21:41:12.736Z",
"sites": [ ],
"orgs": [
"551ad253f0a9c0686f71ed08"
],
"users": [
"545bbecb7bdd6769632504a7"
],
"rateLimit": 60,
"status": "confirmed",
"application": {
"_id": "55131cd036c09f7d07883dfc",
"description": "OAuth Testing Application",
"homepage": "https://webflow.com",
"name": "Test App",
"owner": "545bbecb7bdd6769632504a7",
"ownerType": "Person"
}
}
In the response object, you should be able to see the value for the rate limit
{
"rateLimit": 60,
}
How to increase your Webflow API rate limit
It seems that their is no way to increase the site’s Webflow API rate limit manually. You’ll need to get in contact with a Webflow staff member and request it to up the limit.
I like to tweet about Node.js and post helpful code snippets. Follow me there if you would like some too!