How to permanently remove a DOM element with JavaScript
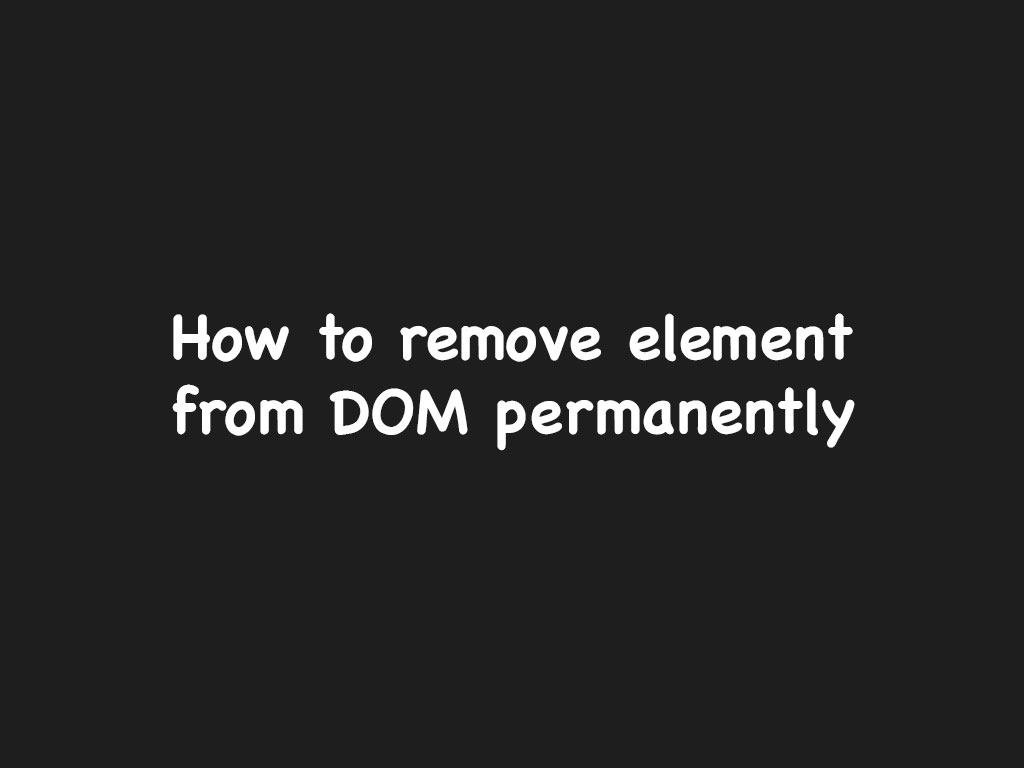
99% of the time, to hide/remove elements from a user, you can use display:none;
. But I recently came across a use case where I needed to remove the element from the DOM permanently. Is there a solution to remove an element from the DOM with JavaScript?
The answer is yes! You can use node.removeChild()
JavaScript utility function to remove elements from the DOM permanently.
Let’s go over how to use node.removeChild
with a code example.
The solution
Let’s say you have a list of cats.
<ul>
<li id="1">Whiskey</li>
<li id="2">Carmella</li>
<li id="3">Fluffy</li>
</ul>
Let’s say I want to remove Carmella out of the list. Here’s how this is done in JavaScript:
// Step 1: Select element you desire to remove
const carmella = document.querySelector('#2');
// Step 2: Check if she exist in the list
if (carmella) {
// Remove from element permanently from the DOM
// Step 3: Reference back to the parent element and execute removeChild()
// Step 4: Pass in element you desire to remove
carmella.parentElement.removeChild(carmella);
}
By using node.removeChild()
you will permanently remove an element from the DOM. Super simple!
Hey, you’ve made it this far! If you found this article helpful, I would appreciate it if you liked or retweet the tweet below!
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!