How to run multiple NPM scripts in parallel
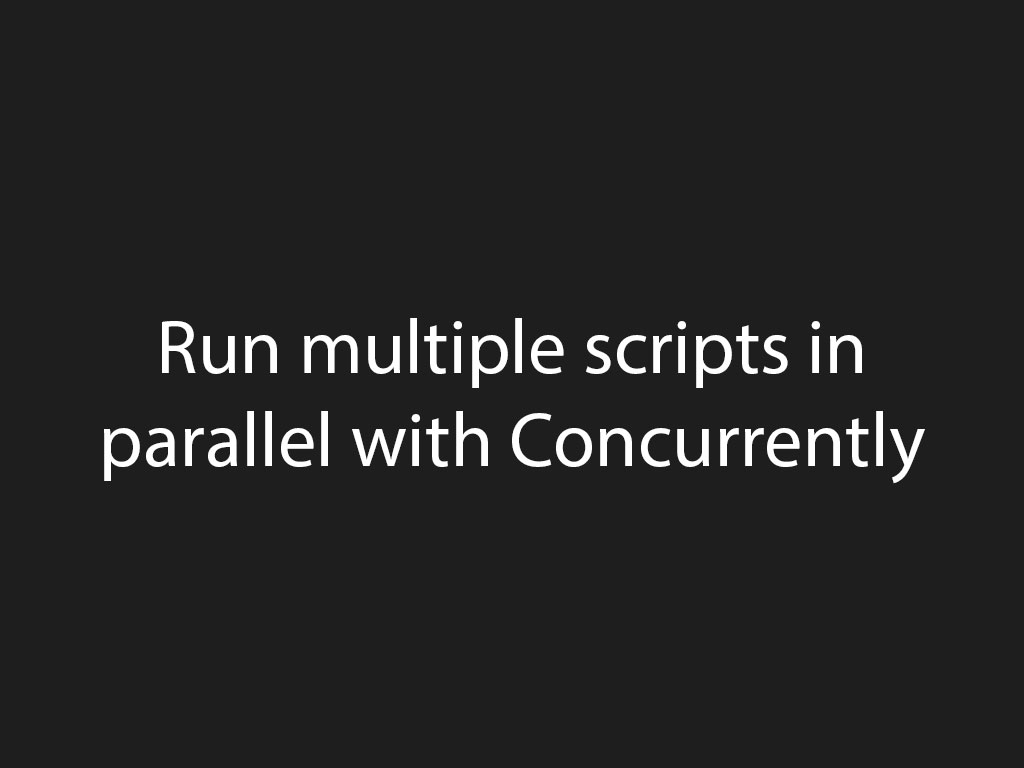
For a while I was running multiple NPM scripts by opening multiple terminal tabs. That got really annoying and asked myself, “How can I run these scripts in parallel?”
I then found a NPM module called Concurrently. Concurrently allowed me to run multiple commands in parallel!
Let’s do a step-by-step process to get you running commands in parallel.
Step 1: Install the concurrently NPM module
The first step is to install concurrently
into your project, and save it as a dev dependency in your package.json file.
npm i -D concurrently
Step 2: Create your package.json dev scripts
Let’s do a couple watch scripts for SASS/CSS and TypeScript. And will use concurrently
do run them in parallel.
{
"name": "test-app",
"scripts": {
"dev": "concurrently npm:watch:*",
"watch:css": "sass --watch styles/main.scss:public/css/main.css",
"watch:js": "tsc *.ts --watch"
},
"devDependencies": {
"concurrently": "^5.3.0",
"sass": "^1.32.0",
"typescript": "^4.1.3"
}
}
As you can see in the code above I have two package.json scripts, watch:css & watch:js
, that watch all changes for SASS code and TypeScript.
I wrote another script called dev
.
The dev script
uses the concurrently module to run all the watch scripts.
The colon(:
) in the script is equivalent to npm run
. You can use an asterisk(*
) as a wildcard.
Step 3: Run your dev package.json script
When you run your concurrently command you should see in your terminal that all your watch scripts are running in parallel.
npm run dev
Hey, you’ve made it this far! If you found this article helpful, I would appreciate it if you liked or retweet the tweet below!
I like to tweet about code and post helpful code snippets. Follow me there if you would like some too!