How to solve TypeScript possibly undefined value
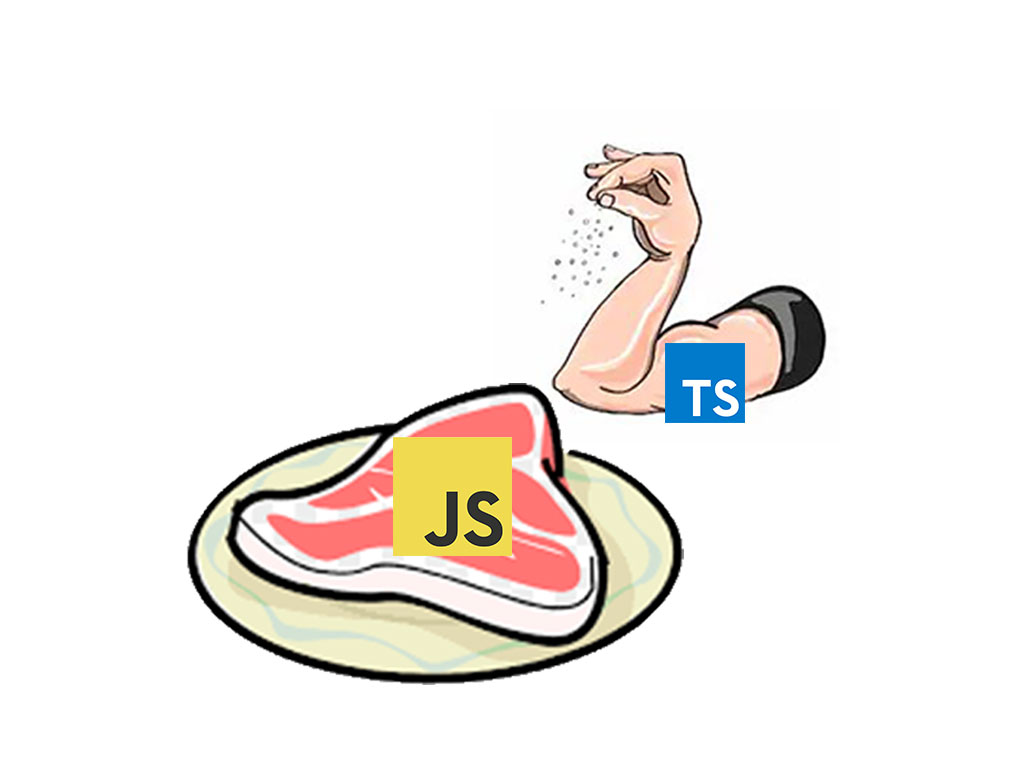
Have you ever tried to access a property in an object, but you get a TypeScript error message such as:
TS2532: Object is possibly 'undefined'.
Or maybe, you want to pass a variable in a function
function validateToken(token: string) {
return token;
}
const token = 'kjadj' as string | undefined;
validateToken(token);
and you end up getting a TypeScript error message like this:
TS2322: Type 'string | undefined' is not assignable to type 'string'. Type 'undefined' is not assignable to type 'string'.
This happens because TypeScript expects a specific value type but you’re providing an incorrect value type. If you like to learn more about JavaScript value types, feel free to check out this article, “JavaScript data types: Intro“.
In this article, I’m going to show you 5 different methods to handle this situation.
Method #1: Use IF conditions
if (token) {
validateToken(token);
}
The example above checks if the variable, token
, is a falsy or truthy value.
This works because we’ve originally said that token
maybe be a string value or undefined.
I highly recommend you to be a little bit more specific on your check. Use the typeof
keyword and make sure its the right JavaScript value type.
if (typeof token === 'string') {
validateToken(token);
}
Method #2: Use the OR logical operator
Another method is to use OR operator (||
).
validateToken(token || 'default-token');
If token
doesn’t meet validateToken()
argument requirement, than pass a fallback value.
In the example above, if the value of token
is undefined, then the string "default-token"
will be used as the fallback.
Method #3: Use the keyword as
The keyword as
, can be used to let TypeScript know, that you know the value is going to be whatever value type it expects.
Here’s an example:
validateToken(token as string)
In the example above, I’m passing token
, and letting TypeScript know that even though the variable may be undefined; at this point of the app, it will be a string. So it has nothing to worry about.
Method #4: Use !
non-null assertion operator
After a variable is being used, you may add the exclamation mark (!
) after the variable.
validateToken(token!);
This similar to method #3. Sometimes the TypeScript compiler isn’t able to determine what type of value it may at a certain point.
By adding the exclamation mark (!
) at the end, you let the TypeScript compiler that there is no way this variable will be undefined or null.
Method #5: Use ??
nullish coalescing operator
validateToken(token ?? 'default-token');
This method is very similar to method #2, OR logical operator (||
). The difference is that the OR logical operator checks for falsy values.
Falsy values are false
, undefined
, null
, 0
, NaN
, and a empty string.
Nullish coalescing operator (??
) only checks for undefined or null.
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about TypeScript and post helpful code snippets. Follow me there if you would like some too!