How to use Array.map() to render data in React
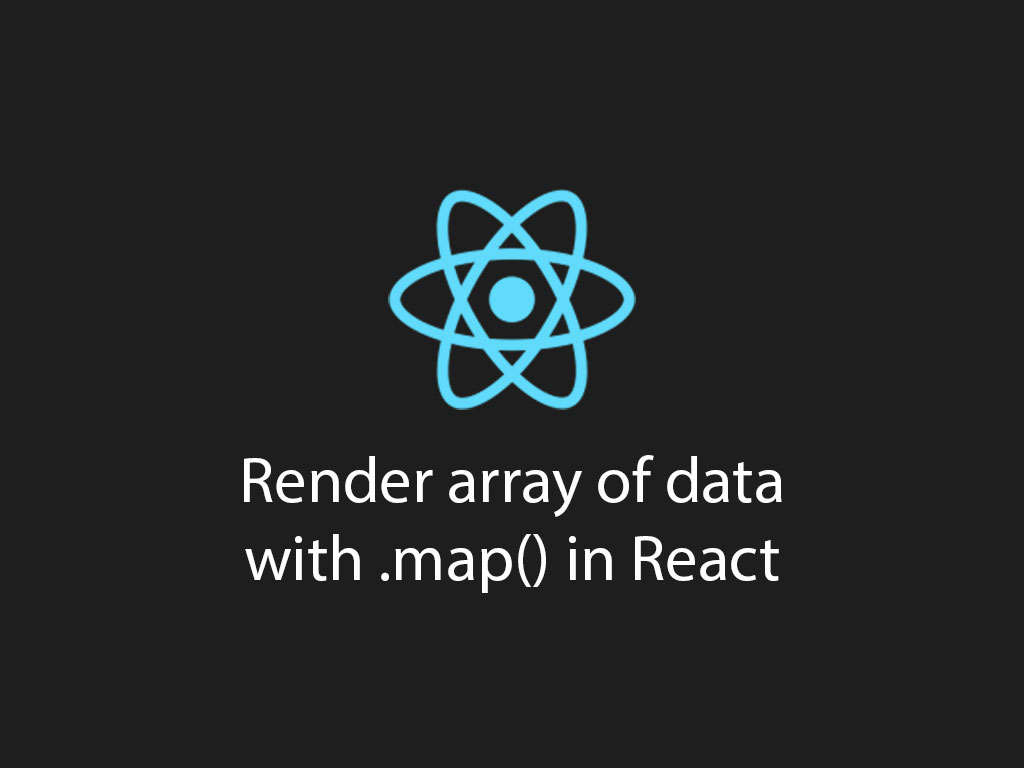
Let’s say you have an array of objects with user information. How do you render that data in React?
const people = [
{ id: 1, name: "Johnny", gender: "male", age: 30 },
{ id: 2, name: "Jenny", gender: "female", age: 28 },
{ id: 3, name: "Sam", gender: "male", age: 13 },
{ id: 4, name: "Dean", gender: "male", age: 8 }
];
The answer is, you use Array.map()
in your component and return JSX elements inside the Array.map()
callback function to render the UI.
Here’s an example how to use Array.map()
in React.
<ul>
{people.map(person => {
return (
<li key={person.id}>
{person.name} - {person.age} years old
</li>
)
})}
</ul>
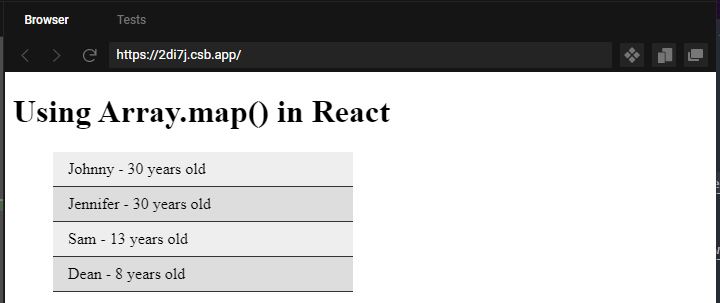
Let’s breakdown this code.
In the JSX section you’re going to start by adding curly braces ({}
).
{people.map(callback)}
The curly braces ({}
) are a special syntax to let the JSX parser know that it needs to interpret the part as JavaScript.
In the example above, I want to execute an Array.map()
to render the people data.
Array.map()
is going to loop through the array of data, and give you access of each item in the array in a callback function.
In the callback function you’re going to need to return JSX elements to render the UI you desire.
{people.map(person => {
return (
<li key={person.id}>
{person.name} - {person.age} years old
</li>
)
})}
In the example above, I’m returning a li
HTML element that displays to the browser the name of the person and their age.
A couple things to note in the code example above.
Any time you render HTML/JSX elements in Array.map()
you must pass the key prop.
<li key={person.id}></li>
If you don’t, it may cause some weird side effects. And make sure that each value is unique.
The next thing to note, is that I’m using the curly braces ({}
) inside the loop. to display the person data.
<li key={person.id}>
{person.name} - {person.age} years old
</li>
The rule of thumb is, you can use the curly braces as many times as you want but it must be nested within JSX elements.
Here’s some examples of class components and functional components using Array.map()
to render an array of data to the browser.
How to use map() with a React class component
class App extends React.Component {
people = [
{ id: 1, name: "Johnny", gender: "male", age: 30 },
{ id: 2, name: "Jenny", gender: "female", age: 28 },
{ id: 3, name: "Sam", gender: "male", age: 13 },
{ id: 4, name: "Dean", gender: "male", age: 8 }
];
render() {
return (
<ul>
{this.people.map(person => {
return (
<li key={person.id}>
{person.name} - {person.age} years old
</li>
)
})}
</ul>
);
}
}
How to use map() with a React functional component
function App() {
return (
<ul>
{people.map(person => {
return (
<li key={person.id}>
{person.name} - {person.age} years old
</li>
)
})}
</ul>
);
}
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!