JavaScript: Define a variable in IF statement
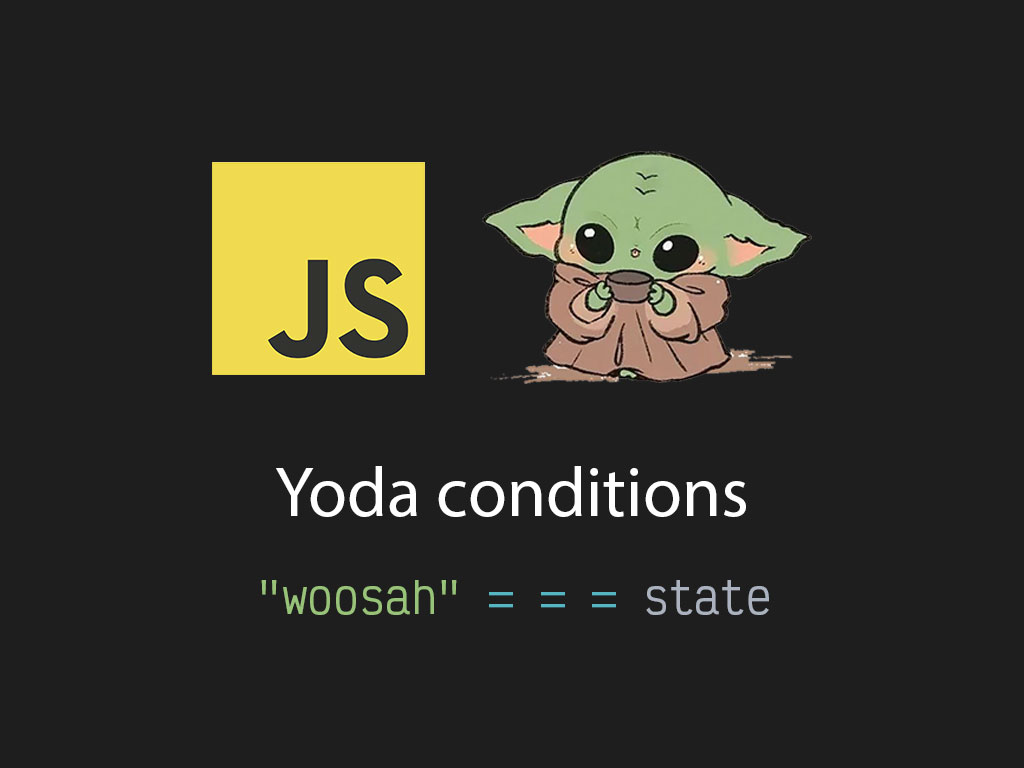
Have you ever tried to write an IF statement to check a primitive/constant value against a JavaScript variable, but accidentally re-defined the variable?
let state = "woosah";
if (state = "not-woosah") {
console.log("not relaxed!");
} else {
console.log("relaxed :)");
}
Accidents happen, what I really meant to write was:
state === "not-woosah"
This statement has 3 equals symbols (===
) which means something completely different.
When I came across this problem for the first time, I asked myself..
Can you define variables inside an IF statement?
The answer is yes you can. Let’s look at the code example above again:
let state = "woosah";
if (state = "not-woosah") {
console.log("not relaxed!"); // This outputs!
} else {
console.log("relaxed :)");
}
What I did there, was re-define a JavaScript variable inside the IF statement.
But you can create a new variable right inside the IF statement parenthesis.
if (state = "woosah") {
console.log(state); // Prints out "woosah"
}
So my next question, which I’m sure your’s is too, should anyone be doing this?
Should you define a variable inside IF statement?
Honestly, there’s no right or wrong answer to this question. JavaScript allows it, so you can make your decision from there.
I haven’t come across a use-case where this makes sense for me to do.
And personally, I think this is prone to bugs. It may also make it difficult to be catch when debugging, and your peers may be speed reading your code and not catch that variable definition.
They may mistake it for an equals check.
To avoid this, there’s a writing style I use called Yoda conditions.
A guide to Yoda conditions
Yoda conditions is a programming style when writing expressions. It’s also known as Yoda notation.
Let’s look at the code example above. I’ve also corrected the statement.
let state = "woosah";
if (state === "not-woosah") {
console.log("not relaxed!");
} else {
console.log("relaxed :)"); // This prints; relaxed
}
In the code example above, I’m checking to see if the variable, state
, equals the string "not-woosah"
.
If that statement is true, than it will print, “Not relaxed!”. Otherwise it will print, “relaxed :)”.
Like I mentioned above, accidents happen, and you may accidentally forget to type the additional equals symbols (===
).
To avoid this error, I use the Yoda condition programming style.
"not-woosah" === state
All I did above, was switch the constant, "not-woosah"
, to the left-hand side.
So just in-case you’re typing really fast, and you write something like this:
"woosah" = state
Instead of
"woosah" === state
Your console should yell at you with this error message:
Uncaught SyntaxError: Invalid left-hand side in assignment
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!