Learning the basics of a Svelte component
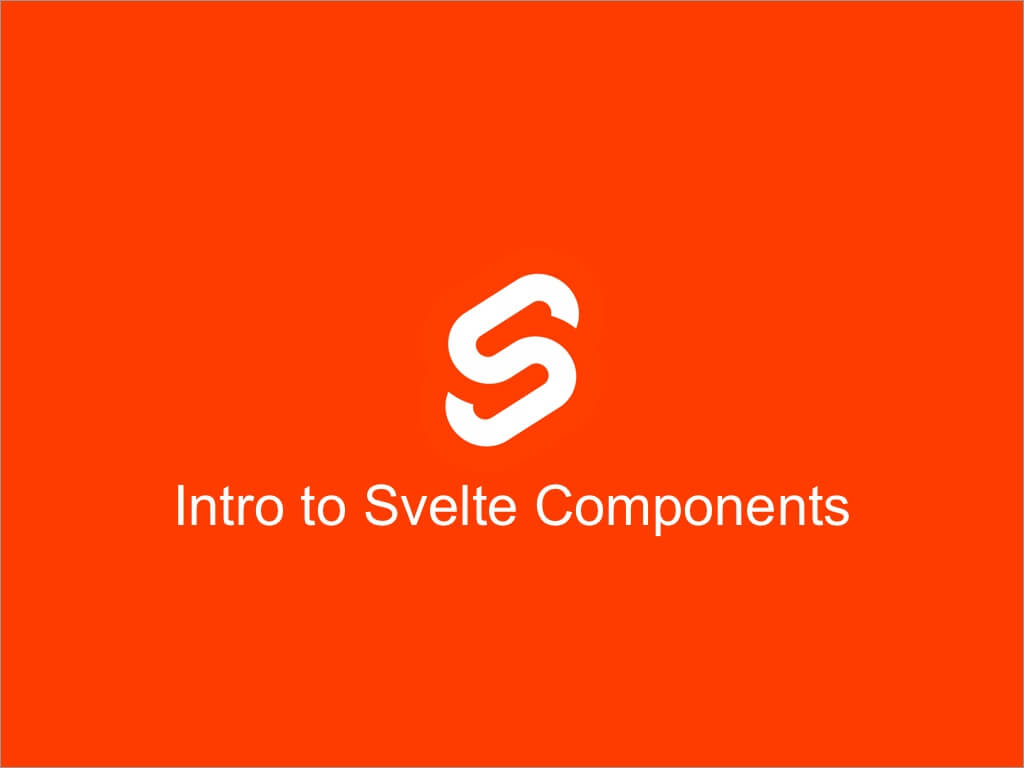
Creating a component in Svelte is extremely simple.
I thought creating a functional component in React couldn’t get any simpler.
import React from 'react';
const GreetMessage = () => (
<h1>Hello John, Ruben here!</h1>
);
export default GreetMessage;
Oh boy, was I wrong. Svelte came in and made creating components much simpler.
Creating the Svelte component
In your text editor, create a new file with the file extension name .svelte
.
You’re literally done!
Or you can use your terminal.
touch Greet.svelte
Exporting a Svelte component
In React it’s pretty simple to export a component. Let’s export the React Greet
component I created above
import React from 'react';
const GreetMessage = () => (
<h1>Hello John, Ruben here!</h1>
);
export default GreetMessage;
Once you’ve created your class or functional React component, you must use the export
keyword or you can use export default
to export that React component.
In Svelte, by default it’s exported as soon you create your Svelte file!
So you can have an empty file and it’s already exported. You don’t have to type any special keywords.
Importing a component
Svelte uses the import
keyword just like React does.
Let’s create a simple Greet
component in Svelte. This component will be used as a child component.
// Greet.svelte
<h1>Hello John, Ruben here!</h1>
<style>
h1 {
color: #ff3e00;
text-transform: uppercase;
font-size: 4em;
font-weight: 100;
}
</style>
Svelte makes writing components as native as possible. It keeps the plain syntax we’ve been use to writing for decades. Plain HTML, and plain styling.
Here’s the main parent component in Svelte using the Greet
component that I created above as a child component.
// App.svelte
<script>
import Greet from './Greet.svelte';
</script>
<main>
<greet></greet>
</main>
<style>
main {
text-align: center;
padding: 1em;
max-width: 240px;
margin: 0 auto;
}
@media (min-width: 640px) {
main {
max-width: none;
}
}
</style>
Inside a <script>
tag, I said to import the Greet
Svelte component.
In Svelte, you must add the file name with the extension, otherwise it will fail the compilation.
In React you can get away with import Greet from './Greet'
. But not in Svelte.
That’s okay, it’s not biggie.
Passing props to child Svelte component
This is an extremely simple step as well.
First I need to modify my Greet
component to accept a prop. This will feel similar if you’re using TypeScript or prop-types with your React project.
I’m going to let my Svelte Greet component accept a property called name
.
<script>
export let name;
</script>
<h1>Hello {name}, Ruben here!</h1>
// ... styles
First I need to do is in a <script>
tag, define an a variable and export it.
Also, I want to replace the string John with the variable, name
.
Back in the App.svelte file will just update the Greet
component.
<Greet name="John"></Greet>
That’s it!
If you were to not define or pass the name property, Svelte will not break, and it will not print the name property.
Using state in a Svelte component
Any variable inside your Svelte component <script>
tag is state property.
Let’s modify the App.svelte file.
<script>
import Greet from './Greet.svelte';
let name = "John";
setTimeout(() => {
name = "Daniel";
}, 2000);
</script>
<main>
<Greet name={name}></Greet>
</main>
I’ve added a new variable called, name
.
name
is assigned to a string that says, John.
I’ve also put a setTimeout()
for 2 seconds. After 2 seconds change name to say Daniel.
I’ve also grabbed name, and tossed to my child component. So my Svelte child component will always receive the new state data.
It honestly cannot get any easier than that.
I like to tweet about Svelte and post helpful code snippets. Follow me there if you would like some too!