Add TypeScript type to Next.js getInitialProps
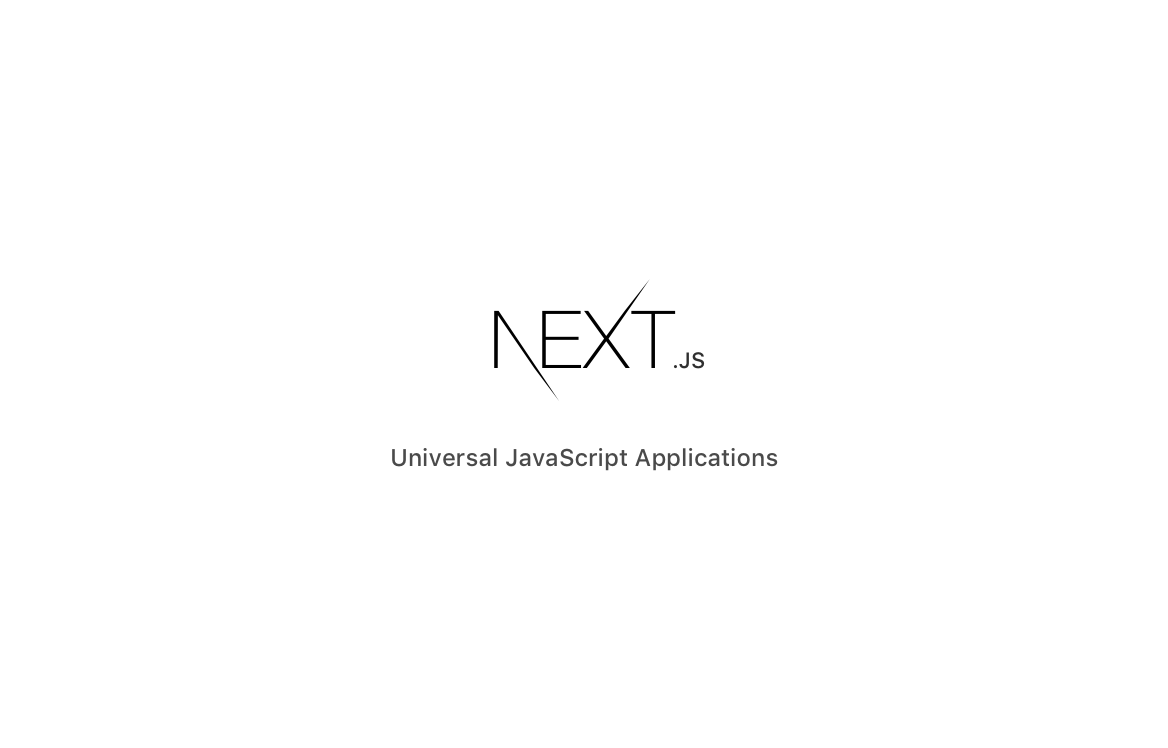
Have you ever seen a similar message like this:
[ts] Property 'getInitialProps' does not exist on type.
This happens because you haven’t given the right type definition to your Next.js page component.
Let’s take a look at a couple examples, how to get rid of this ugly error.
Solution #1: Define your getInitialProps type
interface StatelessPage<P = {}> extends React.SFC<P> {
getInitialProps?: (ctx: any) => Promise<P>
}
And you would use it as such:
const HomePage: StatelessPage<AddMoreInterfaces> = () => {
// ... page markup
};
HomePage.getInitialProps = async () => {
// ... stuff
}
Solution #2: Use NextPageContext
In the example above, I’ve defined ctx
as any
.
But in the TypeScript world, using any
is a sin. So here’s a definition that you replace any for.
import { NextPageContext } from 'next';
interface StatelessPage<P = {}> extends React.SFC<P> {
getInitialProps?: (ctx: NextPageContext) => Promise<P>
}
Pretty easy!
Solution #3: Best solution
Just use the NextPage
interface that Next.js provides.
import { NextPage } from 'next';
const HomePage: NextPage<AddMoreInterfaces> = () => {
// ... page markup
};
HomePage.getInitialProps = async () => {
// ... stuff
}
Happy coding!
I like to tweet about Next.js and post helpful code snippets. Follow me there if you would like some too!