How to use React-i18next withTranslation in TypeScript
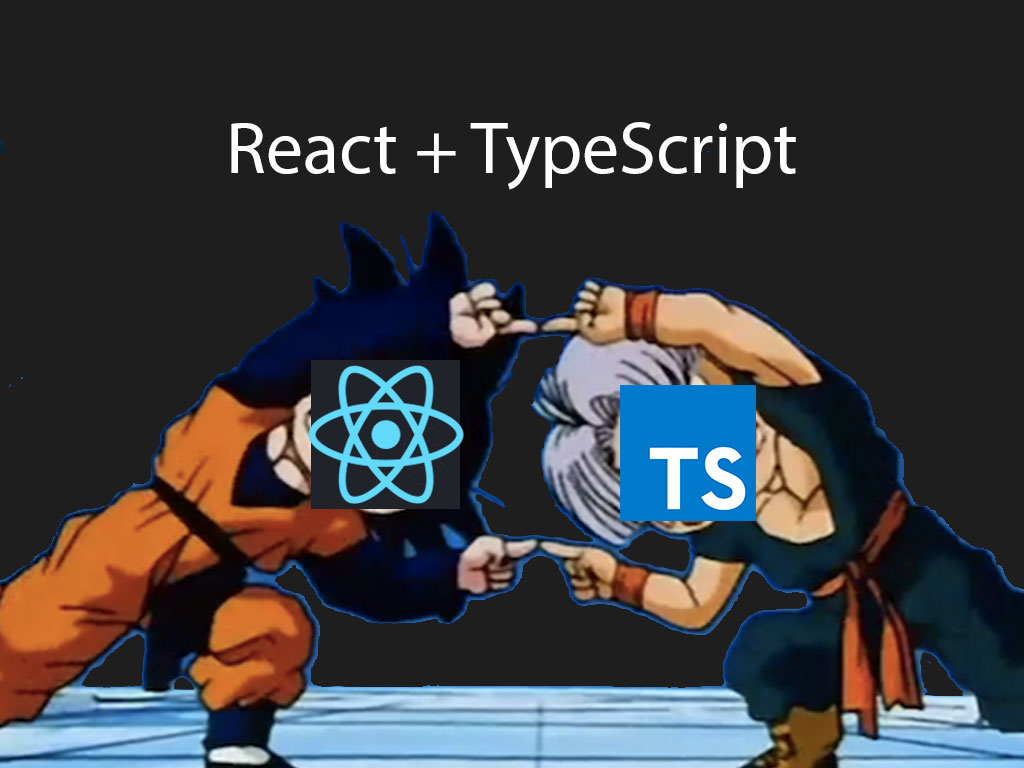
Are you getting an error from TypeScript when you withTranslation()
HOC (higher-order component) for your React component?
import * as React from 'react';
import { withTranslation } from 'react-i18next';
const App: React.FC = props => <h1>{props.t('greetMessage')}</h1>;
export default withTranslation()(App);
Here’s what an error message may look like:
TS2459: Type 'Readonly<> & Readonly<{ children?: ReactNode; }>' has no property 't' and no string index signature.
So how do you we overcome this problem?
Solution
To solve this TypeScript error, it’s quite simple.
When you import react-i18next
, also import the WithTranslation
interface.
import { withTranslation, WithTranslation } from 'react-i18next';
Then you’re going to want to extend the React.FC
type as such:
const App: React.FC<WithTranslation> = (props) => <h1>{props.t('greetMessage')}</h1>;
Here’s an example if you’re using a React class component.
import * as React from 'react';
import { withTranslation, WithTranslation } from 'react-i18next';
class App extends React.Component<WithTranslation> {
render() {
return <h1>{this.props.t('greetMessage')}</h1>;
}
}
export default withTranslation()(App);
Happy coding!
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!