How to rename files in folder with Node.js
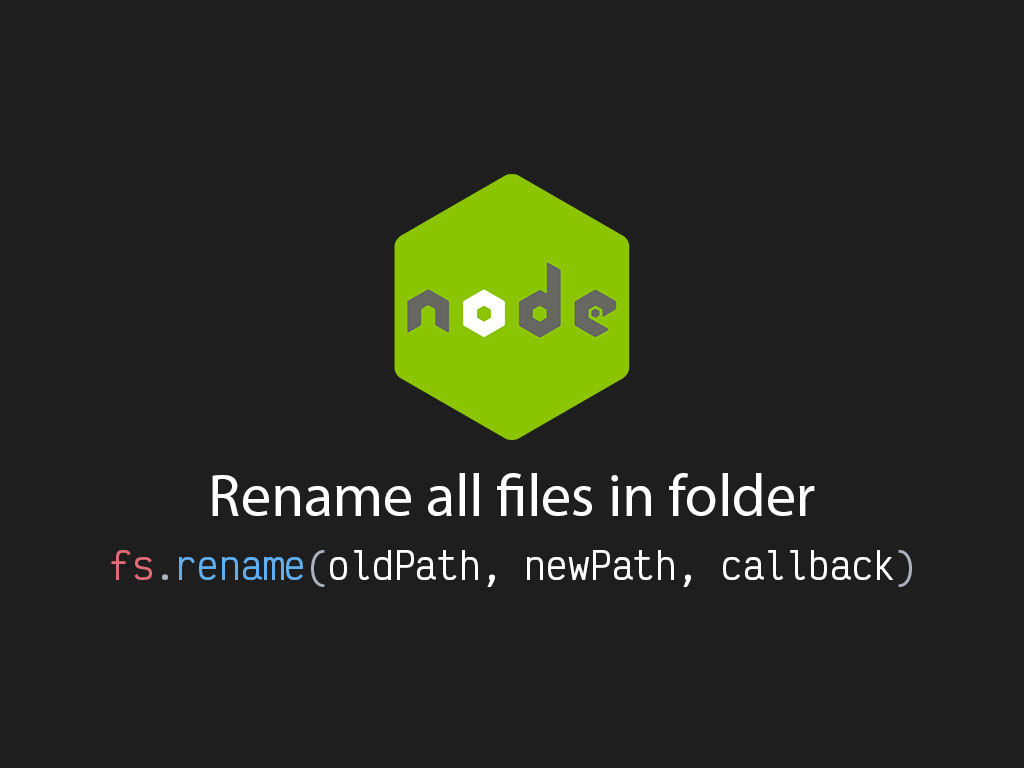
In this article I’m going to show you how to rename all the files in a folder using Node.js.
This article came to be, because I was given a folder of PNG images from a designer with inconsistent file name structure.
For example:
images
- image_Test1.png
- backgroundImage-hero.png
- bacgrkound-Image-Hero-2.png
- Another-image-file.png
Here’s how I did it.
Solution: Use fs.readdirSync and fs.rename to rename files
const { readdirSync, rename } = require('fs');
const { resolve } = require('path');
// Get path to image directory
const imageDirPath = resolve(__dirname, '[imgs_folder_name]');
// Get an array of the files inside the folder
const files = readdirSync(imageDirPath);
// Loop through each file that was retrieved
files.forEach(file => rename(
imageDirPath + `/${file}`,
imageDirPath + `/${file.toLowerCase()}`,
err => console.log(err)
));
Let’s do a code breakdown.
You’ll need to import 2 utility tools from the fs
module from Node.
const { readdirSync, rename } = require('fs');
fs.readdirSync()
– Reads the content of a folder synchronously and returns the name of all the files inside the folder in an array format.
// Get path to image directory
const files = readdirSync(path_to_directory);
fs.rename()
– Will rename the file from the old path to the new path that it’s given. If the file exists in the new path, it will override the content.
rename(old_path, new_path, callback)
Once the file names are retrieved, and we’ve store the the path to the directory in a variable. I’m going to use an Array.forEach()
to loop through each file name so I can rename it as I please.
// Loop through each file that was retrieved
files.forEach(file => {
const oldPath = imageDirPath + `/${file}`;
// lowercasing the filename
const newPath = imageDirPath + `/${file.toLowerCase()}`;
// Rename file
rename(
oldPath,
newPath,
err => console.log(err)
);
});
Pretty easy stuff. Happy coding!
I like to tweet about Node.js and post helpful code snippets. Follow me there if you would like some too!