How to terminate a Node.js process with process.exit()
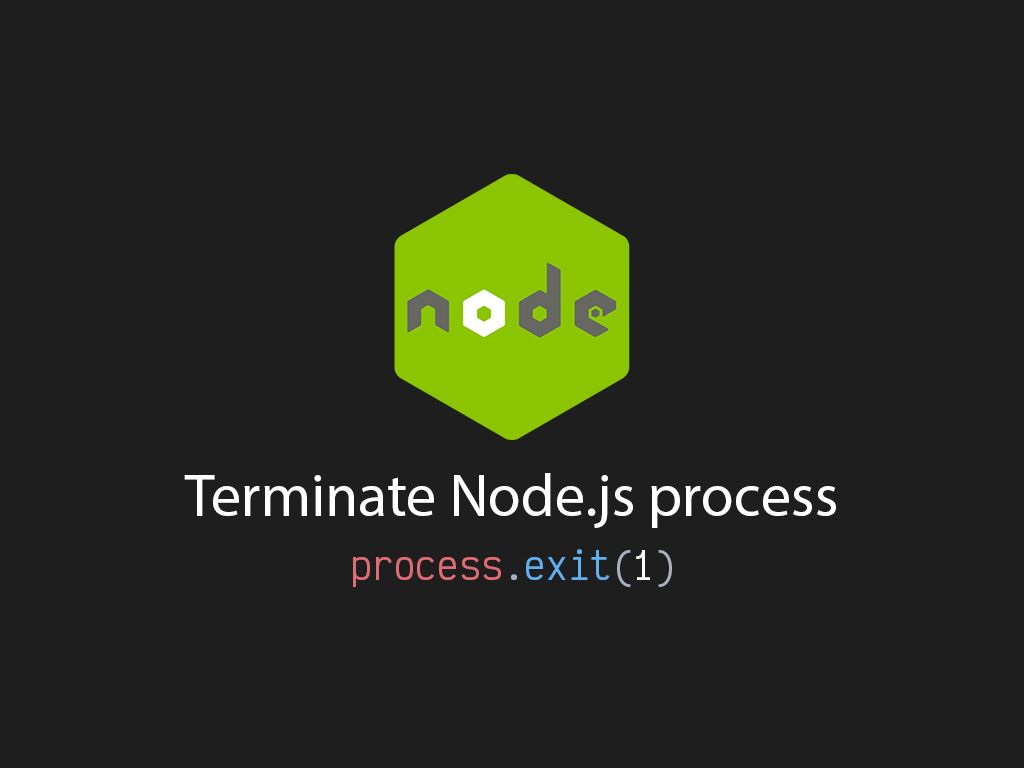
Have you ever ran a Node.js program and it’s just left hanging?
Maybe the Node process hasn’t terminated properly and you’re left clueless? By this point you probably have to manually cancel the process in the terminal.
In this article I’m going to show how to terminate Node.js process with 2 different methods, and explain the exit code statuses when terminating a Node.js process.
process.exit() will force a Node.js process termination
You may have seen this often in Node.js projects.
process.exit();
process.exit()
is the most common command to terminate a Node.js process.
process.exit()
will try to quickly terminate the process even if asynchronous calls have not completed.
fetchCatData('fluffikins'); // I'm still waiting for the content!
process.exit(1); // Don't care, we're shutting down
process.exit() status code list
In the examples above, you may have seen me add 1
as an argument.
process.exit()
accepts arguments which are just status codes or in this case exit codes.
Here’s a list of exit codes and what they do.
Exit code 0
Exit code 0 lets Node.js know to terminate when no more async operations are happening.
process.exit();
That’s the default exit code process.exit() uses when nothing is passed into the function. You may also pass 0.
process.exit(0);
Exit code 1
Exit code 1 is for when unhandled fatal exceptions occur that was not handled by the domain.
process.exit(1);
process.exit()
is one of the methods for Node.js to terminate a process. There is another, and safer approach to terminate a Node.js process.
Exit code 2
This exit code is reserved Bash for misuse.
process.exit(2);
Exit code 3
Node.js exit code 3 is for a status code when internal code cannot be parsed. This code is for development only usage.
process.exit(3);
Exit code 4
Node.js exit code 4 happens rarely and only for development. This exit code happens when your JavaScript source code fails to return a function value.
process.exit(4);
Exit code 5
Exit code 5 is for fatal errors. This happens when V8 engine cannot recover.
process.exit(5);
Use process.exitCode to safely terminate a Node.js process
process.exitCode
allows the Node.js process to terminate naturally and avoids the Node process to do additional work around event loop scheduling.
fetchCatData('fluffikins'); // I'm still waiting for the content!
process.exitCode = 1; // Great, I'll wait till you're done.
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about Node.js and post helpful code snippets. Follow me there if you would like some too!