The best and proven React Webpack plugins for 2021
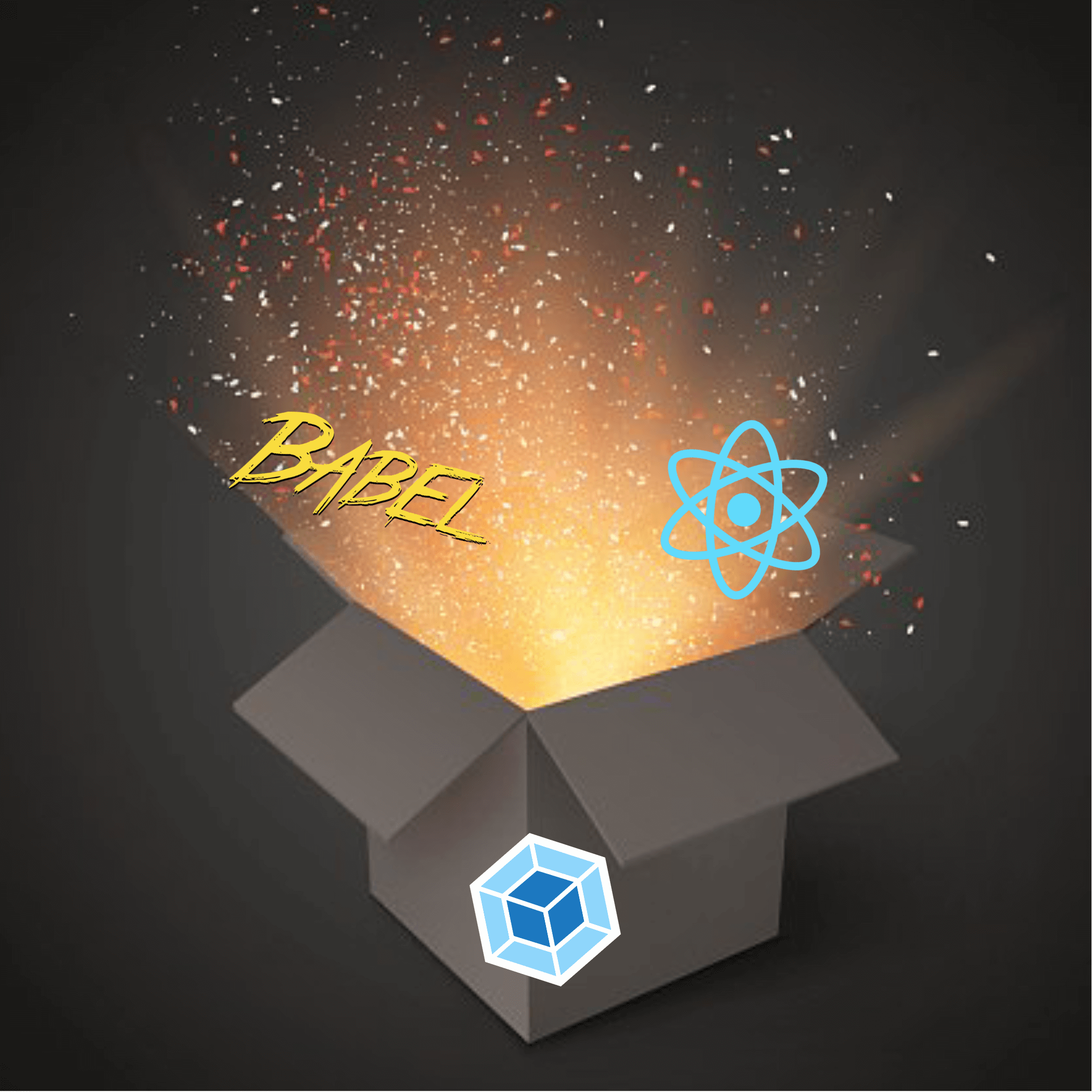
Are you looking to build a React application with Webpack? Then you’re probably looking for the must-have Webpack plugins for your React application.
Here’s a quick list of the plugins that this article covers:
- Terser Webpack plugin
- Optimize CSS assets Webpack Plugin
- HTML Webpack plugin
- Webpack Define plugin
- Mini CSS Extract plugin
- Babel loader
- Babel preset React app
Let’s go over each one these plugins.
Webpack optimization
Webpack comes with a configuration property called optimization.
Inside optimization another property named minimize, and minimizer.
If minimize equals true, then everything inside minimizer will trigger.
optimization: {
minimize: true,
minimizer: [
// ...plugins
]
},
The next couple of Webpack plugins that I will cover, they should be inside the minimizer property.
Terser webpack plugin
The Terser plugin is a great tool to minimize the application JavaScript bundle file for production.
Another plus to this plugin is that it supports ES6+.
Here is a basic configuration for Terser.
new TerserPlugin({
terserOptions: {
parse: {
// We want terser to parse ecma 8 code. However, we don't want it
// to apply minification steps that turns valid ecma 5 code
// into invalid ecma 5 code. This is why the `compress` and `output`
ecma: 8,
},
compress: {
ecma: 5,
warning: false,
inline: 2,
},
mangle: {
// Find work around for Safari 10+
safari10: true,
},
output: {
ecma: 5,
comments: false,
ascii__only: true,
}
},
// Use multi-process parallel running to improve the build speed
parallel: true,
// Enable file caching
cache: true,
})
Source: Terser plugin
Optimize CSS assets Webpack plugin
The Optimize CSS assets Webpack plugin is another one for production build.
It helps optimize and minimize your React CSS code.
new OptimizeCSSAssetsPlugin({
cssProcessorOptions: {
map: {
inline: false,
annotation: true,
}
}
})
Source: Optimize css assets plugin
Webpack plugins
HTML Webpack plugin
The HTML Webpack plugin is a handy plugin for both development and production build.
It tells Webpack to generate an HTML file and inject a script tag with the JavaScript code.
All you need to do is supply the HTML template to use.
new HtmlWebpackPlugin(
Object.assign(
{},
{
inject: true,
template: 'path/to/index.html',
},
// Only for production
process.env.NODE_ENV === "production" ? {
minify: {
removeComments: true,
collapseWhitespace: true,
removeRedundantAttributes: true,
useShortDoctype: true,
removeEmptyAttributes: true,
removeStyleLinkTypeAttributes: true,
keepClosingSlash: true,
minifyJS: true,
minifyCSS: true,
minifyURLs: true
}
} : undefined
)
),
You may also add minification rules such as removing comments, minifying the CSS, and JavaScript.
Source: HTML Webpack plugin
Webpack Define plugin
This plugin comes with the Webpack node module, and it’s a handy little tool when developing your React application.
The DefinePlugin allows you to create environment variables and makes it accessible to your JavaScript code.
new webpack.DefinePlugin({
NODE_ENV: process.env.NODE_ENV || 'development',
PUBLIC_URL: 'path/to/public/dir'
})
Source: Webpack DefinePlugin
Mini CSS Extract plugin
This plugin is another great production tool.
It allows your to extract the CSS in your React app into separate files per JavaScript file.
Some other features that are enjoyable versus the Extract Text Webpack plugin is:
- Async loading
- No duplicate compilation
- Easier to use
new MiniCSSExtractPlugin({
filename: 'static/css/[name].[contenthash:8].css',
chunkFilename: 'static/css/[name].[contenthash:8].chunk.css',
}),
Webpack modules
Babel loader
This plugin allows you to write the latest generation of JavaScript and converts it to a compiled ES5 version.
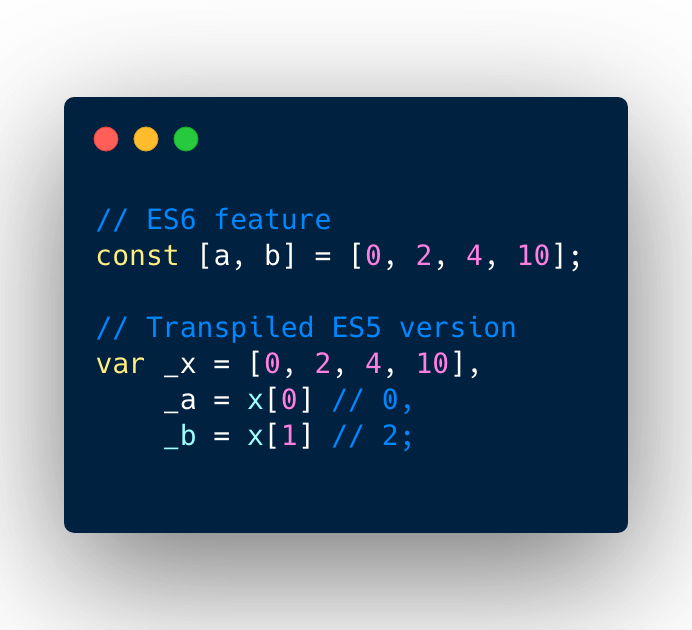
Now to configure babel-loader for .js, .jsx, and as well for Typescript (if you’re using it).
{
test: /\.(js|jsx|ts|tsx)$/,
exclude: /node_modules/,
include: 'path/to/src/file',
loader: 'babel-loader',
}
Source: Babel loader
Babel preset react app
This module is maintained by the Create React App team and it has a great set of presets for your React App.
All we need to do is add more to the babel loader configuration.
{
test: /\.(js|jsx|ts|tsx)$/,
exclude: /node_modules/,
include: 'path/to/src/file',
loader: 'babel-loader',
options: {
presets: [
// Preset includes JSX, TypeScript, and some ESnext features
require.resolve('babel-preset-react-app'),
]
}
}
Source: Babel preset react app
I like to tweet about Webpack and post helpful code snippets. Follow me there if you would like some too!