Why React setState/useState does not update immediately
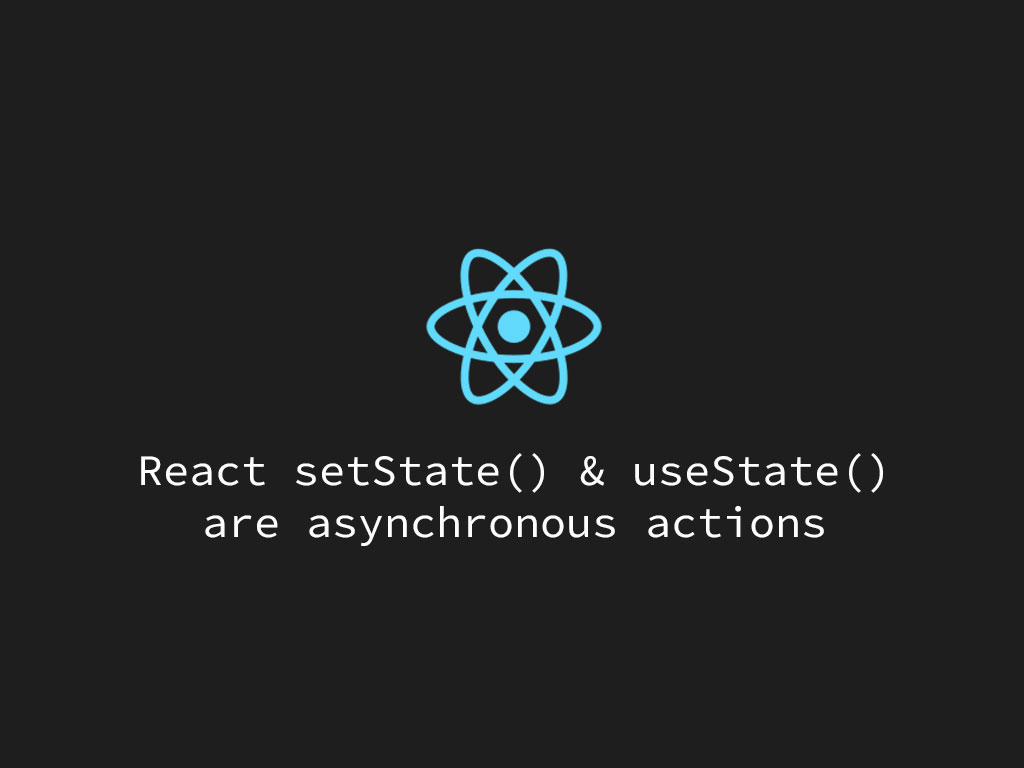
Does it feel like when you call this.setState
or React.useState
, the changes feel like it’s a step behind?
Let’s dive into why this.setState
and React.useState
do not update immediately.
The answer: They’re just queues
React this.setState
, and useState
does not make changes directly to the state object.
React this.setState
, and React.useState
create queues for React core to update the state object of a React component.
So the process to update React state is asynchronous for performance reasons. That’s why changes don’t feel immediate.
So how do you perform an action after state has changed?
First, if you’re looking to become a strong and elite React developer within just 11 modules, you might want to look into Wes Bos, Advanced React course for just $97.00 (30% off). Wouldn’t it be nice to learn how to create end-to-end applications in React to get a higher paying job? Wes Bos, Advanced React course will make you an elite React developer and will teach you the skillset for you to have the confidence to apply for React positions.
Click here to become a strong and elite React developer: Advanced React course.
Disclaimer: The three React course links are affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the course I fully recommend when it comes to becoming a React expert.
React setState callback function after state changes
If you’re using a class component, you will have to usethis.setState()
to update the state of a React component.
this.setState(state, callback);
The second parameter this.setState()
accepts is the callback function, and that’s where you’ll want to add your side effects.
This callback function will get triggered when React state has finished updating.
this.setState(newStateObject, () => {
// ... do some other actions
});
The example above uses the arrow function, but you can do it with a traditional function syntax.
this.setState(newStateObject, function() {
// ... do some other actions
});
But what if you’re not using this.setState
, and you’re using React.useState
?
How do you perform an action after React.useState
hook has triggered?
Use React useEffect after state has changed
React.useState
doesn’t have accept callback function that gets called after React state has actually been modified.
To perform side effects after state has change, you must use the React.useEffect hook
.
React.useEffect(callback, deps);
React.useEffect
accepts a callback function to perform your side effects or actions, and it accepts a list of dependencies in the form of an array.
That hook function will only activate if the values in the list change.
Let’s take a look at an example
let s;
const Foo = () => {
const [counter, setCounter] = React.useState(0);
// Emmulate componentDidMount lifecycle
React.useEffect(() => {
s = setInterval(() => {
setCounter(state => (state +1));
}, 1000);
}, []);
// This is for counter state variable
React.useEffect(() => {
if (counter > 9) {
clearInterval(s);
}
}, [counter]);
return <span>{counter}</span>;
};
The first React.useEffect hook
has an empty array. Since there are no dependencies, it only gets triggered once.
But in the second React.useEffect hook
, I added my state variable as a dependency.
Any time my state variable, counter
, changes in value, then the second React.useEffect
function will re-activate. So it behaves like the React componentDidUpdate lifecycle
.
First, if you’re looking to become a strong and elite React developer within just 11 modules, you might want to look into Wes Bos, Advanced React course for just $97.00 (30% off). Wouldn’t it be nice to learn how to create end-to-end applications in React to get a higher paying job? Wes Bos, Advanced React course will make you an elite React developer and will teach you the skillset for you to have the confidence to apply for React positions.
Click here to become a strong and elite React developer: Advanced React course.
Disclaimer: The three React course links are affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the course I fully recommend when it comes to becoming a React expert.
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!