4 methods to force a re-render in React
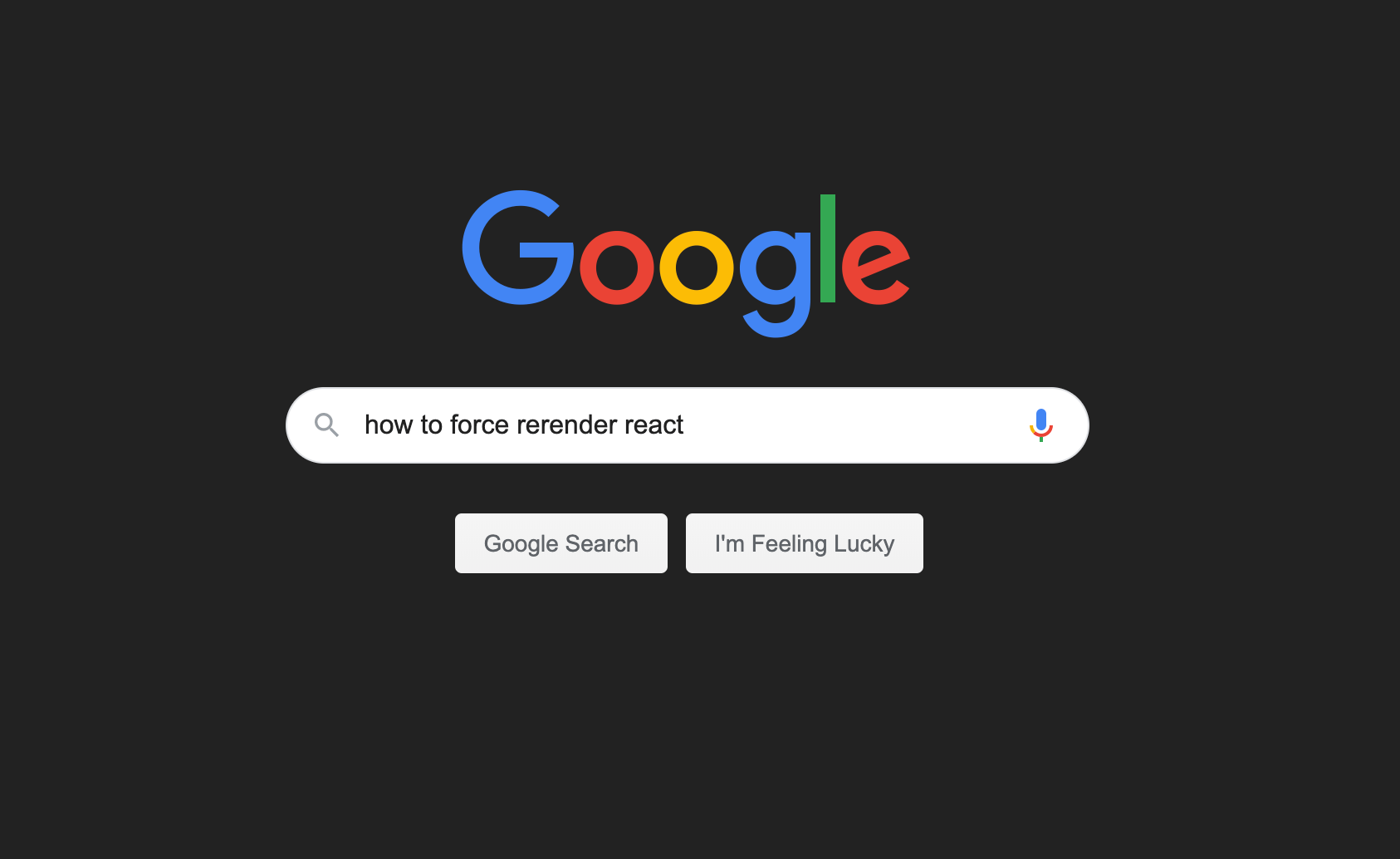
Are you looking to for a way to force a re-render on a React Component?
Here are a few methods to re-render a React component.
First, if you’re looking to become a strong and elite React developer within just 11 modules, you might want to look into Wes Bos, Advanced React course for just $97.00 (30% off). Wouldn’t it be nice to learn how to create end-to-end applications in React to get a higher paying job? Wes Bos, Advanced React course will make you an elite React developer and will teach you the skillset for you to have the confidence to apply for React positions.
Click here to become a strong and elite React developer: Advanced React course.
Disclaimer: The three React course links are affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the course I fully recommend when it comes to becoming a React expert.
1. Re-render component when state changes
Any time a React component state has changed, React has to run the render()
method.
class App extends React.Component {
componentDidMount() {
this.setState({});
}
render() {
console.log('render() method')
return <h1>Hi!</h1>;
}
}
In the example above I’m update the state when the component mounts.
You can also re-render a component on an event, such as a click event.
class App extends React.Component {
state = {
mssg: ""
};
handleClick = () => {
this.setState({ mssg: "Hi there!" });
};
render() {
console.log("render() method");
return (
<>
<button onClick={this.handleClick}>Say something</button>
<div>{this.state.mssg}</div>
</>
);
}
}
Both outputs will look like this:
render() method
render() method
2. Re-render component when props change
class Child extends React.Component {
render() {
console.log('Child component: render()');
return {this.props.message};
}
}
class App extends React.Component {
state = {
mssg: ""
};
handleClick = () => {
this.setState({ mssg: "Hi there!" });
};
render() {
return (
<>
<button onClick={this.handleClick}>Say something</button>
<Child message={this.state.mssg} />
</>
);
}
}
In the example above, <Child />
component does not have state, but it does have a custom prop that it accepts, message
.
When the button gets clicked on it will update the <Child />
component, and cause it to run the render()
lifecycle again.
Child component: render()
Child component: render()
3. Re-render with key prop
I showed an example how to cause a re-render and run the componentDidMount()
lifecycle, here.
By changing the value of the key
prop, it will make React unmount the component and re-mount it again, and go through the render()
lifecycle.
4. Force a re-render
This is a method that is highly discouraged. Always use props & state changes to cause a new render.
But nonetheless, here is how you can do it!
class App extends React.Component {
handleClick = () => {
// force a re-render
this.forceUpdate();
};
render() {
console.log('App component: render()')
return (
<>
<button onClick={this.handleClick}>Say something</button>
</>
);
}
}
Conclusion
If you need to re-render a React component, always update the components state and props.
Try to avoid causing re-render with key
prop, because it will add a bit more complexity. But There are odd use cases where this is needed.
Never use forceUpdate()
to cause a re-render.
First, if you’re looking to become a strong and elite React developer within just 11 modules, you might want to look into Wes Bos, Advanced React course for just $97.00 (30% off). Wouldn’t it be nice to learn how to create end-to-end applications in React to get a higher paying job? Wes Bos, Advanced React course will make you an elite React developer and will teach you the skillset for you to have the confidence to apply for React positions.
Click here to become a strong and elite React developer: Advanced React course.
Disclaimer: The three React course links are affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the course I fully recommend when it comes to becoming a React expert.
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!