How to destructure a React component props object
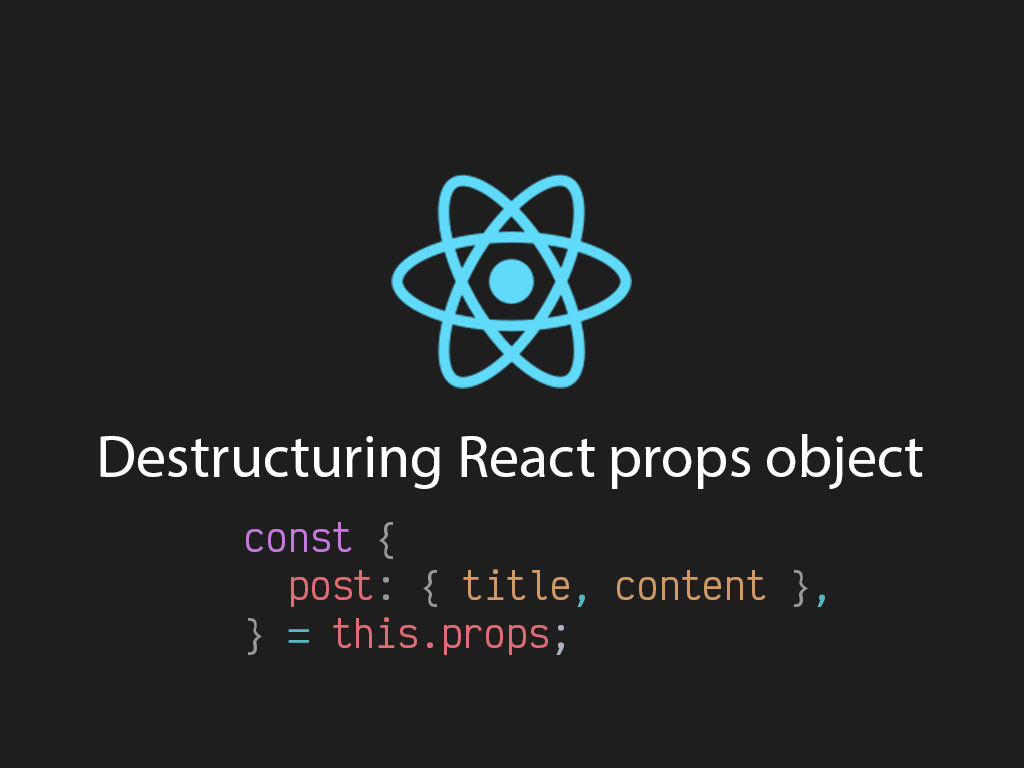
Going through the chain of React props to get a value can be mundane and really hard to read afterwards.
For example
const PostPage = props => (
<>
<Head>
<title>{props.post.title.rendered}</title>
<meta name="description" content={props.post.excerpt.rendered} />
<link rel='canonical' href={`https://linguinecode.com/post/${props.post.slug}`} />
</Head>
<main>
<h1>{props.post.title}</h1>
<div>
{props.post.content.rendered}
</div>
</main>
</>
);
Would you like to know how to clean this up a bit, and make yourself look like a hero?
In this article I’ll show you a few methods on how to destructure a React component props object, so you can fix that ugliness above.
Destructure React props object
You can destructure the React props object within the render method inside a React class component.
class PostPage extends React.Component {
render() {
const {
post: { title, content, excerpt, slug }
} = this.props;
return (
<>
<Head>
<title>{title.rendered}</title>
<meta name="description" content={excerpt.rendered} />
<link
rel="canonical"
href={`https://linguinecode.com/post/${slug}`}
/>
</Head>
<main>
<h1>{title.rendered}</h1>
<div>{content.rendered}</div>
</main>
</>
);
}
}
You can also destructure the React props object within a React functional component.
const PostPage = (props) => {
const {
post: { title, content, excerpt, slug }
} = this.props;
return (
<>
<Head>
<title>{title.rendered}</title>
<meta name="description" content={excerpt.rendered} />
<link
rel="canonical"
href={`https://linguinecode.com/post/${slug}`}
/>
</Head>
<main>
<h1>{title.rendered}</h1>
<div>{content.rendered}</div>
</main>
</>
);
};
Destructure React functional component argument
You can also destructure the props object from the arguments signature method if you’re using a React functional component.
const PostPage = ({
post: { title, content, excerpt, slug }
}) => (
<>
<Head>
<title>{title.rendered}</title>
<meta name="description" content={excerpt.rendered} />
<link
rel="canonical"
href={`https://linguinecode.com/post/${slug}`}
/>
</Head>
<main>
<h1>{title.rendered}</h1>
<div>{content.rendered}</div>
</main>
</>
);
Desctructure React props object with TypeScript
But what if you’re using TypeScript?
When you desctructure the props object, do you use the type any
?
No, you don’t use the type any
. The type any
is seemed as a sin in the TypeScript world.
Let me show you how to destructure the React props object in TypeScript.
The first step is to create an interface or type in TypeScript.
Here’s an interface definition for the React component that I’ve been using in this article.
interface PostPageProps {
post: {
title: { rendered: string };
excerpt: { rendered: string };
content: { rendered: string };
slug: string;
};
}
Make sure that you’ve defined your interface or type correctly, because if you miss a property, you might see a similar error as such:
Property does not exist on value type
Now that I’ve defined this interface, I want to embed it to my React component so I can destructure the React props object without any TypeScript errors.
Here’s how to do it in a React class component:
class PostPage extends React.Component<PostPageProps> {
render() {
const {
post: { title, content, excerpt, slug }
} = this.props;
return (
<>
<Head>
<title>{title.rendered}</title>
<meta name="description" content={excerpt.rendered} />
<link
rel="canonical"
href={`https://linguinecode.com/post/${slug}`}
/>
</Head>
<main>
<h1>{title.rendered}</h1>
<div>{content.rendered}</div>
</main>
</>
);
}
}
And here’s how to destructure a React functional component in TypeScript:
const PostPage: React.SFC<PostPageProps> = ({
post: { title, content, excerpt, slug }
}) => (
<>
<Head>
<title>{title.rendered}</title>
<meta name="description" content={excerpt.rendered} />
<link rel="canonical" href={`https://linguinecode.com/post/${slug}`} />
</Head>
<main>
<h1>{title.rendered}</h1>
<div>{content.rendered}</div>
</main>
</>
);
In the example right above, I’m assigning a type to the variable PostPage
.
const PostPage: React.SFC<PostPageProps> = (props) => ();
I’m saying that it’s going to be a React stateless function component, and that it will also use the PostPageProps
interface that was defined earlier.
That’s one method of assigning a type to a React functional component.
Another method is to assign the PostPageProps
interface to the argument variable itself.
const PostPage = ({
post: { title, content, excerpt, slug }
}: PostPageProps) => {...};
Whichever method is fine. These 2 methods will still allow you to desctructure the React props object without any issues.
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!