How to do conditional rendering in Svelte
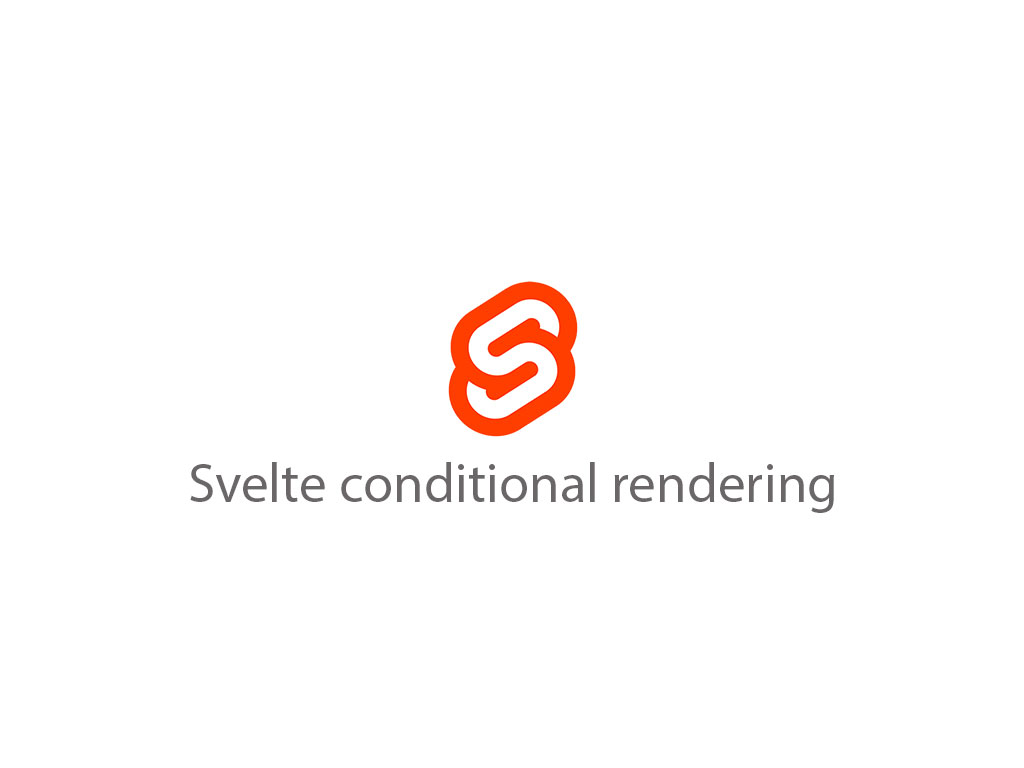
Rendering a component is extremely simple.
<!-- App.svelte -->
<main>
<h1>Hello World!</h1>
</main>
But what if you want to render a specific HTML element or Svelte component depending on a prop or state value?
if blocks conditional rendering
<script>
import { onMount } from 'svelte';
let greet = false;
onMount(() => {
setTimeout(() => {
greet = true;
}, 2000)
});
</script>
<main>
{#if greet}
<h1>Hello World!</h1>
{/if}
</main>
First I’m importing the onMount
function, and creating a variable called greet
.
I’m then changing the value of greet
2 seconds after the component mounts.
Inside the <main>
tag I’m adding my if
condition block.
<main>
{#if greet}
<h1>Hello World!</h1>
{/if}
</main>
Basic if
conditions start with a special syntax, {#if statement}
.
And it must end with {/if}
.
If-else blocks conditional rendering
<main>
{#if greet}
<h1>Hello World!</h1>
{:else}
<h1>Loading...</h1>
{/if}
</main>
As you can see in the example above, the new syntax between {#if statement}
and {/if}
is {:else}
The HTML code in between {:else}
and {/if}
is considered the else statement
In the code example above, until greet
turns into true, I will show the user the loading text.
else-if blocks conditional rendering
You can add additional conditionals with else-if statements.
<script>
import { onMount, afterUpdate } from 'svelte';
let status = 'FROZEN';
// Change status to fetch 2 seconds after mount
onMount(() => {
setTimeout(() => {
status = 'FETCHING';
}, 2000);
});
// Set status to `READY` 2 seconds after `FETCHING` status
afterUpdate(() => {
if ('FETCHING' === status) {
setTimeout(() => {
status = 'READY';
}, 2000);
}
});
</script>
<main>
{#if 'READY' === status}
<h1>Hello World!</h1>
{:else if 'FETCHING' === status}
<h1>Loading...</h1>
{:else}
<h1>Initiating</h1>
{/if}
</main>
In the example above, I’ve moved the text, “Loading…” under a else-if condition.
The condition requires the variable, status
, to equal FETCHING
.
I like to tweet about Svelte and post helpful code snippets. Follow me there if you would like some too!