Conditionally adding props to React Components
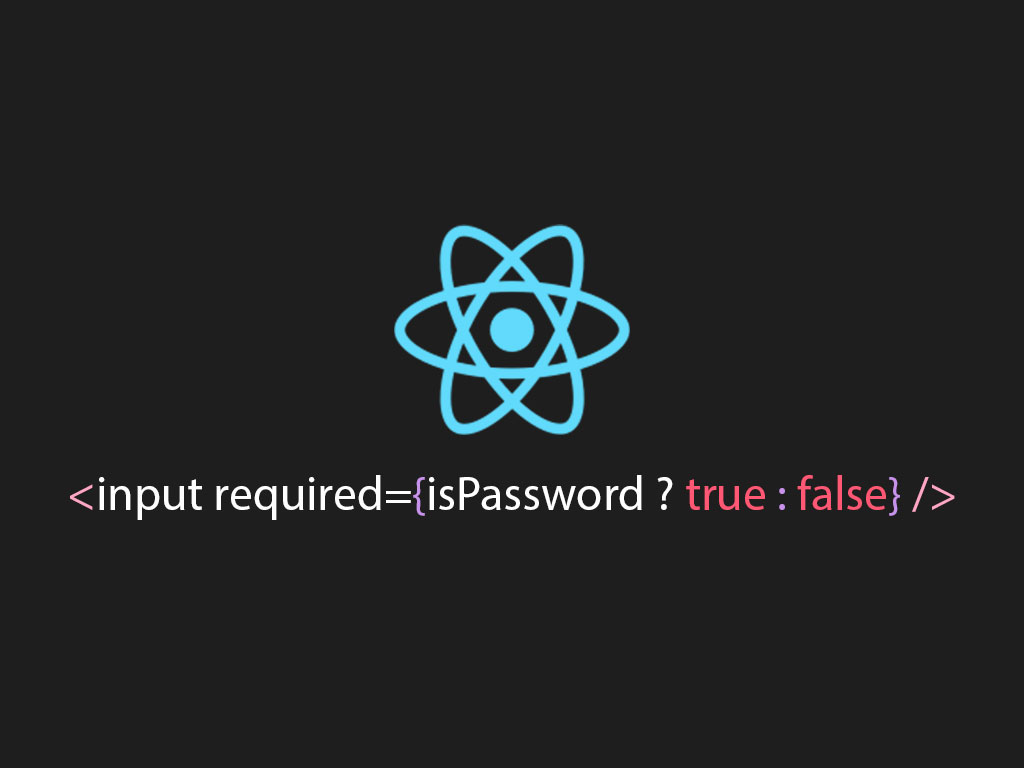
Can you only add attributes to a React component
if a certain conditions are met?
Great news you can!
Let’s go over a couple techniques.
Solution #1: Inline conditionals in attribute props
You can perform inline conditional statements inside a property attribute.
Here’s an example:
function App() {
const [mood] = React.useState("happy");
const greet = () => alert("Hi there! :)");
return (
<button onClick={greet} disabled={"happy" === mood ? false : true}>
Say hi
</button>
);
}
Solution #2: if statements
Adding inline conditionals inside attributes may be okay if it’s only 1 or 2 attributes. But if a React component has too many, it may get to clunky, and confusing to read.
So in this example I moved the conditional above the return statement.
function App() {
let disabled = true;
const [mood] = React.useState("happy");
const greet = () => alert("Hi there! :)");
if ("happy" === mood) {
disabled = undefined;
}
return (
<button onClick={greet} disabled={disabled}>
Say hi
</button>
);
}
Also note how I’m passing undefined if the mood equals “happy”.
React is smart enough to NOT pass a prop value if the value is falsy.
Falsy values are null, undefined, and false.
Solution #3: Spread props to child component
In the second example I moved the conditional logic above, and created variable for disabled
.
Creating too many variables for each prop may be tedious. For example:
function App() {
let required = false;
let type = "password";
let disabled = true;
if ("password" === type) {
required = true;
disabled = false;
}
return <input type={type} disabled={disabled} required={required} />;
}
Even typing this was hurting me!
You can simplify this code by using the object spread syntax. Here’s how:
function App() {
let fieldProps = {
type: 'password',
disabled: true,
};
if ("password" === fieldProps.type) {
fieldProps.required = true;
fieldProps.disabled = false;
}
return <input {...fieldProps} />;
}
This may save you a few keystrokes.
If you’re interested in how to conditionally render React components go to this article, “4 React conditional rendering methods with props and state“.
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!