The difference between React component vs pure component
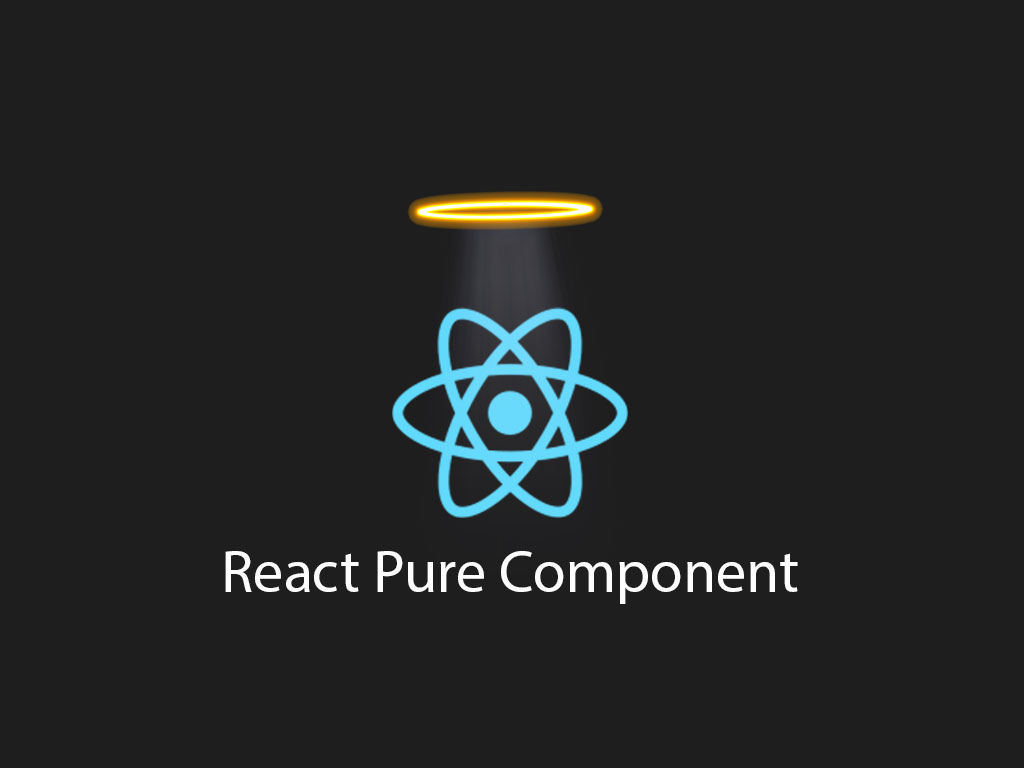
In a recent article, I talked about how to avoid multiple re-rendering in React by using shouldComponentUpdate.
Using React shouldComponentUpdate() lifecycle method is a valid option, but there is a better way.
That’s by using the React Pure Component class instead of a regular React Component class.
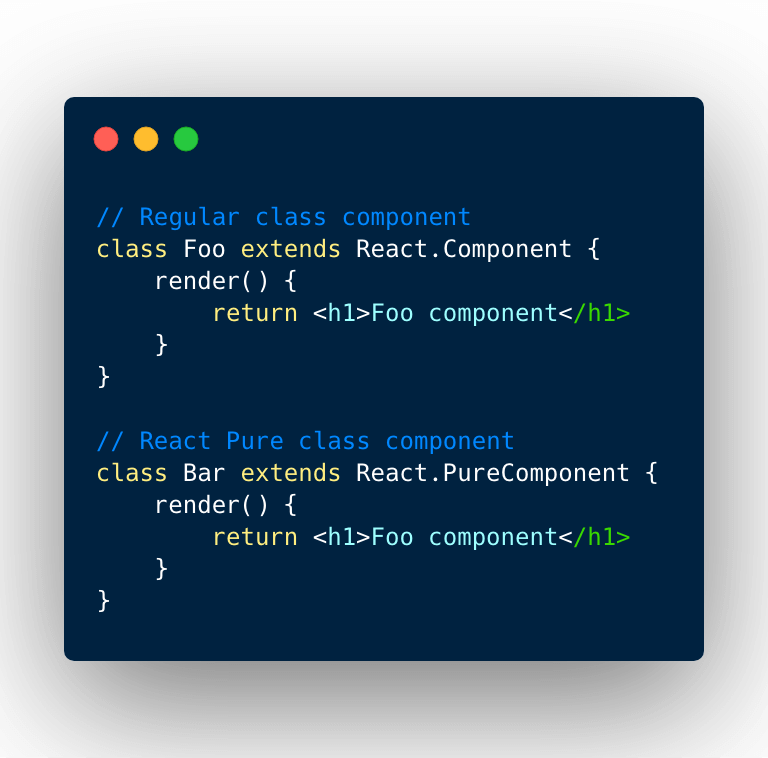
What is the difference between React Pure Component vs React Component?
The biggest difference between React pure component vs a regular React component is that a React component doesn’t implement shouldComponentUpdate() by default.
On the other hand, React pure component does implement shouldComponentUpdate() by default, and by performing a shallow comparison on React state and props values.
How to use React Pure Component
In a recent article, I showed an example of how to create a pure like component using the regular React component class with the shouldComponentUpdate lifecycle method.
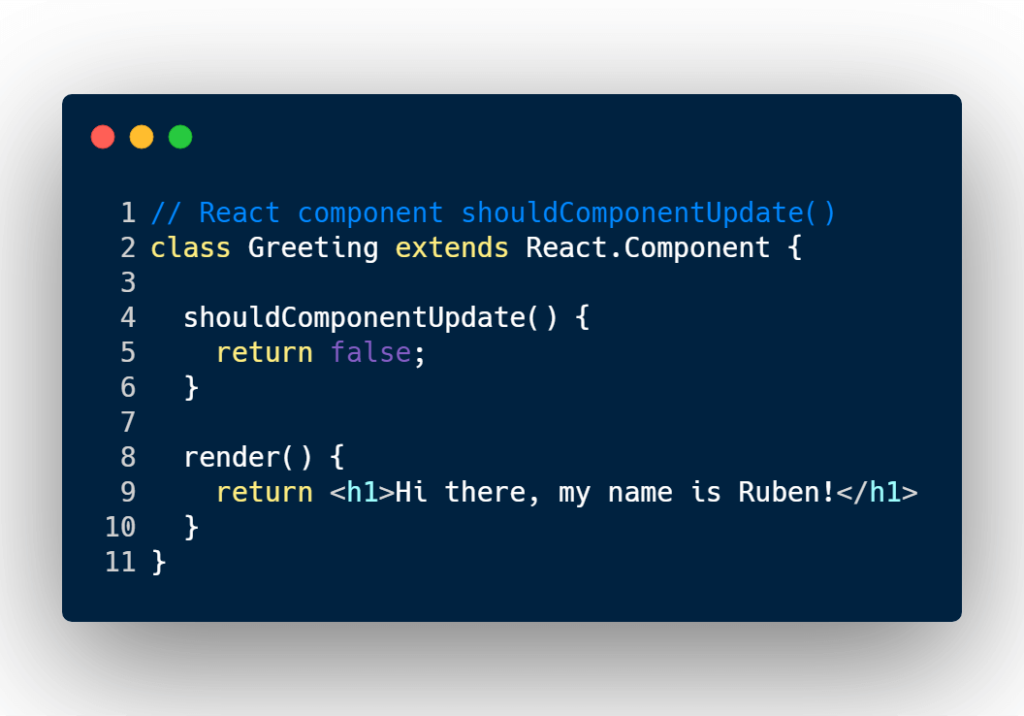
We’re gonna reduce this code to 5 lines by using React Pure Component.
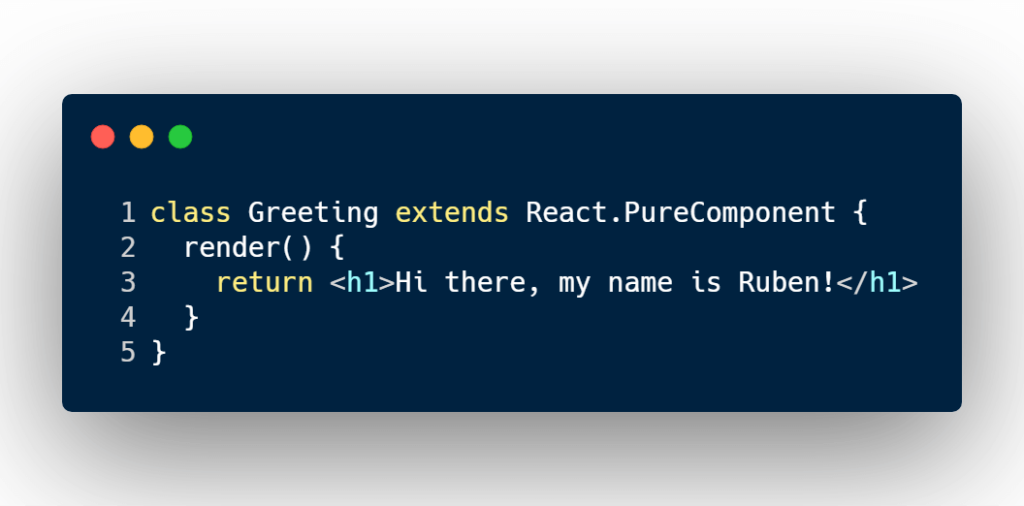
It’s that simple to use.
When to use React Pure Component
Only use React pure component when the React component won’t be mutated by state or props.
Also, if you plan to use React children components inside your pure component, make sure those children components are pure as well.
That’s because any children components inside a pure component will not get re-render.
To see the full example go here: GitHub link
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!