How to avoid multiple re-renders in React (3 lines of code)
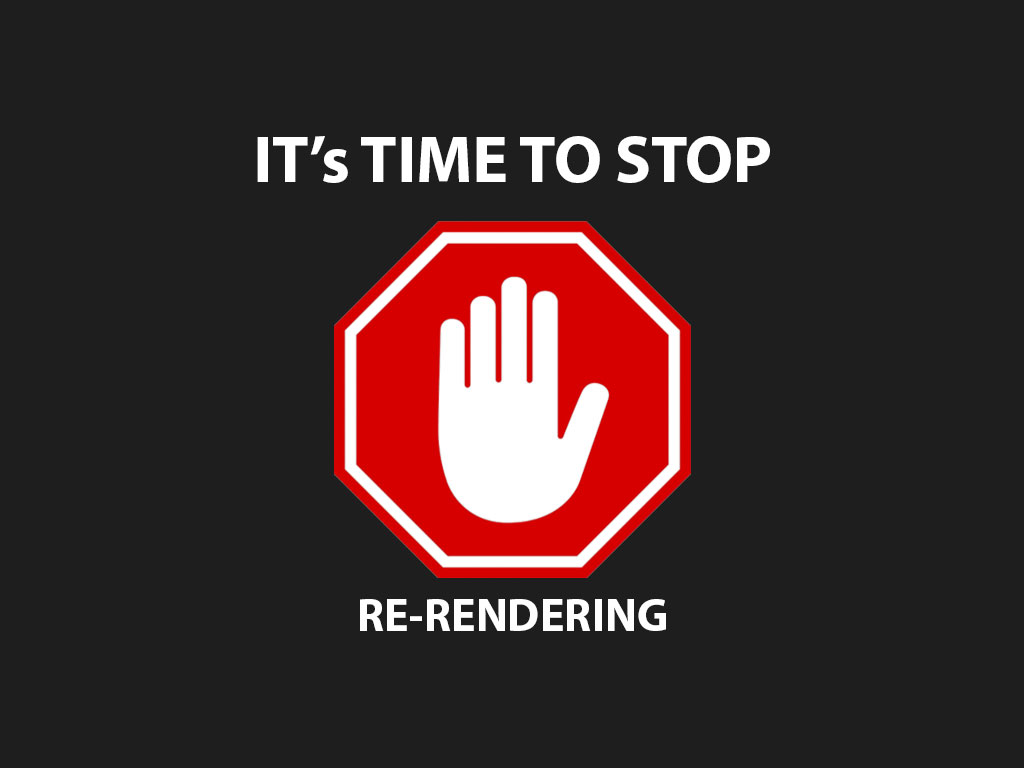
Are you noticing too many re-renders from a component and its sub components?
If that’s the case, this can be bad news for you.
Take a look at the image below.
componentDidMount() {
this.setState({ ruinPerformance: true });
}
render() {
return (
<SubComp>
<SubComp>
<SubComp>
<SubComp>
Hello world :)
</SubComp>
</SubComp>
</SubComp>
<SiblingComp />
</SubComp>
);
}
If React state changes in the parent component, every single sub component would re-render by default.
What if the SiblingComp
or the last nested SubComp
doesn’t need to get re-render?
Is there a way to avoid re-rendering?
To avoid re-rendering per component with the you will use the shouldComponentUpdate() lifecycle
.
First, if you’re looking to become a strong and elite React developer within just 11 modules, you might want to look into Wes Bos, Advanced React course for just $97.00 (30% off). Wouldn’t it be nice to learn how to create end-to-end applications in React to get a higher paying job? Wes Bos, Advanced React course will make you an elite React developer and will teach you the skillset for you to have the confidence to apply for React positions.
Click here to become a strong and elite React developer: Advanced React course.
Disclaimer: The three React course links are affiliate links where I may receive a small commission for at no cost to you if you choose to purchase a plan from a link on this page. However, these are merely the course I fully recommend when it comes to becoming a React expert.
Let’s go over some basics.
What does the React render function do?
React render
is one of the many component lifecycles that a React component goes through.
The render
method is required whenever you’re creating a new React component.
React render
requires you to return a value. This may either be null, undefined or JSX markup.
During this lifecycle you should always keep it pure, and avoid modifying state.
Modify state inside the React componentDidMount
lifecycle.
This is because the render function may be triggered multiple times.
Which may cause your app to go through an infinite loop, then crashing.
So this beg to question,
When does React decide to re-render a component?
The first render gets triggered after the componentWillMount
lifecycle.
It then gets triggered after the React componentWillUpdate
lifecycle.
After a React component does mount, it will be listening for any React props or state that has changed.
Once it detects something has changed, it will, by default, re-render the entire React component and it’s child components.
Is it bad to re-render multiple React components?
Yes, and no.
In theory, having every component re-render can be expensive, but React is pretty fast, and it’s really good at noticing what’s different and what’s not.
This is more of an optimization question.
The best way to measure the performance of these re-renders if to use React’s performance NPM module tool.
To learn more about it go here.
So you’re next question may be, how do I avoid multiple re-renders in React?
When to use React shouldComponentUpdate?
React shouldComponentUpdate
is a performance optimization method, and it tells React to avoid re-rendering a component, even if state or prop values may have changed.
Only use this method if when a component will stay static or pure.
The React shouldComponentUpdate
method requires you to return a boolean value.
Return true if it needs to re-render or false to avoid being re-render.
How to use React shouldComponentUpdate?
Here’s a basic example of how to use React shouldComponentUpdate
.
First I’ll start by creating 2 basic React components.
class Greeting extends React.Component {
render() {
console.log('Greeting - Render lifecycle');
return <h1>Hi there, my name is Ruben!</h1>;
}
}
class App extends React.Component {
render() {
console.log('App - Render lifecycle');
return <Greeting />;
}
}
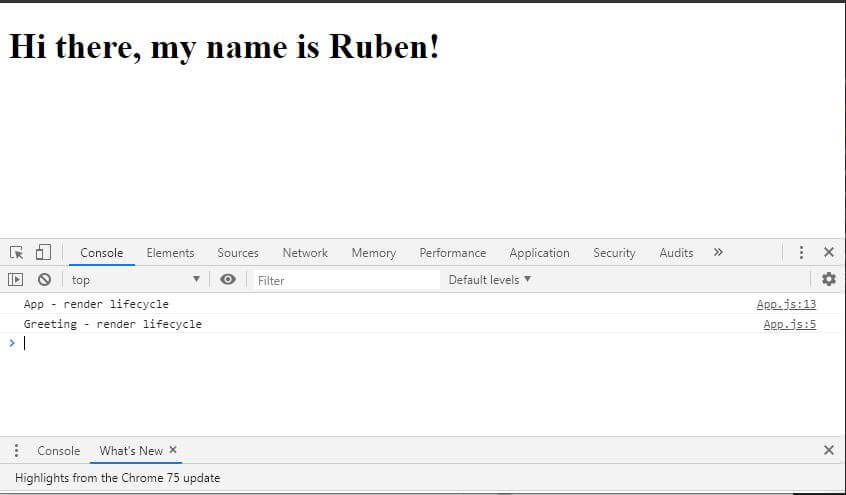
In the images above, I’ve created 2 React components.
One is a greeting component, and the other is the app component.
Each React component is console logging a message during the render
lifecycle.
Next, I will add React state, and update the state value in the componentDidMount
React lifecycle.
class App extends React.Component {
state = {
greeted: false,
};
componentDidMount() {
this.setState({ greeted: true });
}
render() {
console.log('App - Render lifecycle');
return <Greeting />;
}
}
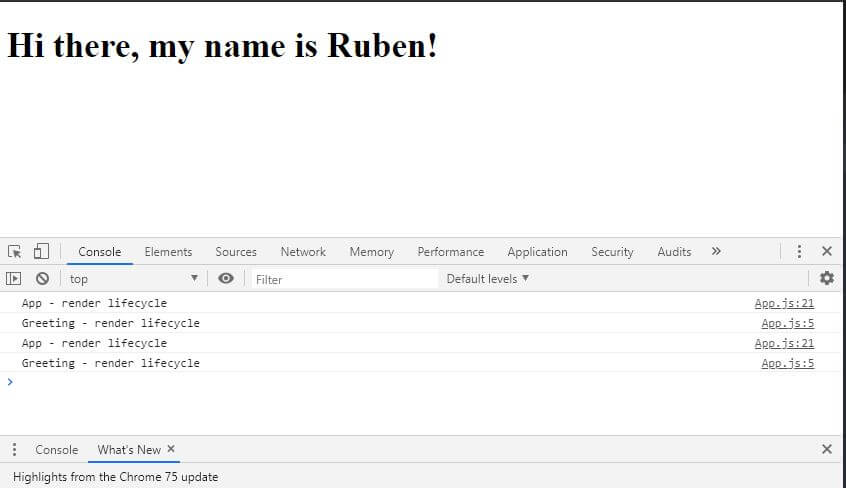
You can see in the console tab, that the render lifecycle got triggered more than once on both the app and greeting component.
This is because the React app component got re-rendered after the state values were modified, and it also re-rendered its child components.
We can assume that the greeting component is static, and that it’s not ever going to change.
I’m going to prevent it from being re-render now.
The first step is to add the shouldComponentUpdate
lifeycle to the greeting component and make it return false
.
class Greeting extends React.Component {
shouldComponentUpdate() {
console.log('Greeting - shouldComponentUpdate lifecycle');
return false;
}
render() {
console.log('Greeting - Render lifecycle');
return <h1>Hi there, my name is Ruben!</h1>;
}
}
In the code image above, I’m console logging a message when the component gets to the shouldComponentUpdate
lifecycle.
This will tell React to ignore this component as it’s updating its virtual DOM.
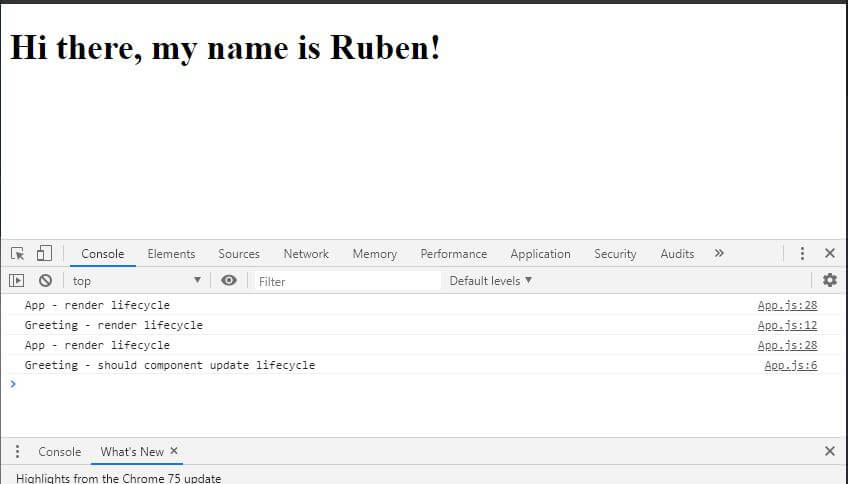
On the console tab, You can see the App and Greeting component went through a round of the render
lifeycle.
Then the App
component went through the render
lifeycle again after the state values were changed.
But the Greeting component did not console log the render lifecycle message.
Hooraay! That was easy.
shouldComponentUpdate
also provides arguments that contain future values for props and/or state.
shouldComponentUpdate(nextProps, nextState);
So you can perform any conditional logic with current and future values, and determine whether you need the component to update or not.
Conclusion
Only use React shouldComponentUpdate
when your app hits a performance bottleneck.
Or when it’s extremely obvious that a component you’re building will be static at all time.
If you’re interested in learning how to optimize your React components with a much simpler approach, check out the next article: The difference between React component vs pure component
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!