How to enable CORS on your WordPress REST API
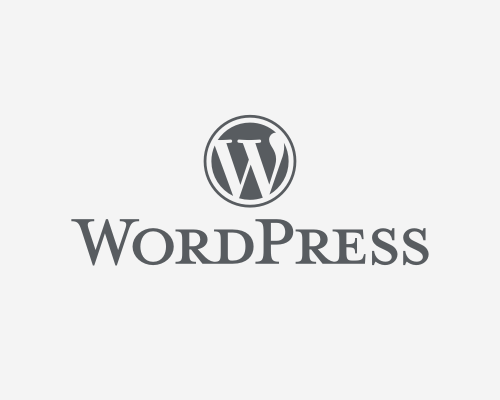
Are you trying to customize the Access-Control-Allow-Headers
property for your WordPress API?
I wrote an article about, “How to fetch WordPress data with JavaScript“. This is usually done because you want to create a headless WordPress site.
Now that you got WordPress rest API up and running, you might not want to let anyone ping your site but your own site only.
First, before you enable CORS on your WordPress site you need to host your WordPress site. If you’re looking to launch a WordPress site for your blog or business, you might want to look into launching your blog with Bluehost for just $3.95/mo (49.43% off). They make it really easy to select an affordable plan, and create or transfer a domain.
Get started with Bluehost.
Disclaimer: The two Bluehost links above are affiliate links which provide a small commission to me at no cost to you. These links track your purchase and credit it to this website. Affiliate links are a primary way that I make money from this blog and Bluehost is the best web hosting option for new bloggers.
Let’s dive into enabling configuring your CORS settings.
Setup your header CORS function
In your functions.php file add the following code.
<?php
function initCors( $value ) {
$origin_url = '*';
// Check if production environment or not
if (ENVIRONMENT === 'production') {
$origin_url = 'https://linguinecode.com';
}
header( 'Access-Control-Allow-Origin: ' . $origin_url );
header( 'Access-Control-Allow-Methods: GET' );
header( 'Access-Control-Allow-Credentials: true' );
return $value;
}
In the example above, I’ve set the variable $origin_url
to equal the asterisk (*
), which means all.
But in the following if conditional
, I’m checking if the environment is in production mode, change the $origin_url
value to my main site URL.
This is helpful when you’re testing locally, or maybe testing an environment that is not production.
This is also assuming that $origin_value
is from a different server or site, that is making the request to your WordPress site.
If you want to only allow same origin, you will have to change the value of Access-Control-Allow-Origin
to
header( 'Access-Control-Allow-Origin: ' . esc_url_raw( site_url() ) );
Enable your init CORS function
The next step is to attach the function that was created above to a WordPress filter called rest_pre_serve_request
.
But before you do that, you must remove the current one.
And we’re going to add this under the WordPress action called rest_api_init
.
<?php
// ... initCors function
add_action( 'rest_api_init', function() {
remove_filter( 'rest_pre_serve_request', 'rest_send_cors_headers' );
add_filter( 'rest_pre_serve_request', initCors);
}, 15 );
Allow multiple origins
You may add multiple origin support. All you need to do is create an array of allowed origins, and check if the origin coming in is allowed.
function initCors( $value ) {
$origin = get_http_origin();
$allowed_origins = [ 'site1.example.com', 'site2.example.com', 'localhost:3000' ];
if ( $origin && in_array( $origin, $allowed_origins ) ) {
header( 'Access-Control-Allow-Origin: ' . esc_url_raw( $origin ) );
header( 'Access-Control-Allow-Methods: GET' );
header( 'Access-Control-Allow-Credentials: true' );
}
return $value;
}
I like to tweet about WordPress and post helpful code snippets. Follow me there if you would like some too!