How to make WordPress headless and fetch posts with JavaScript
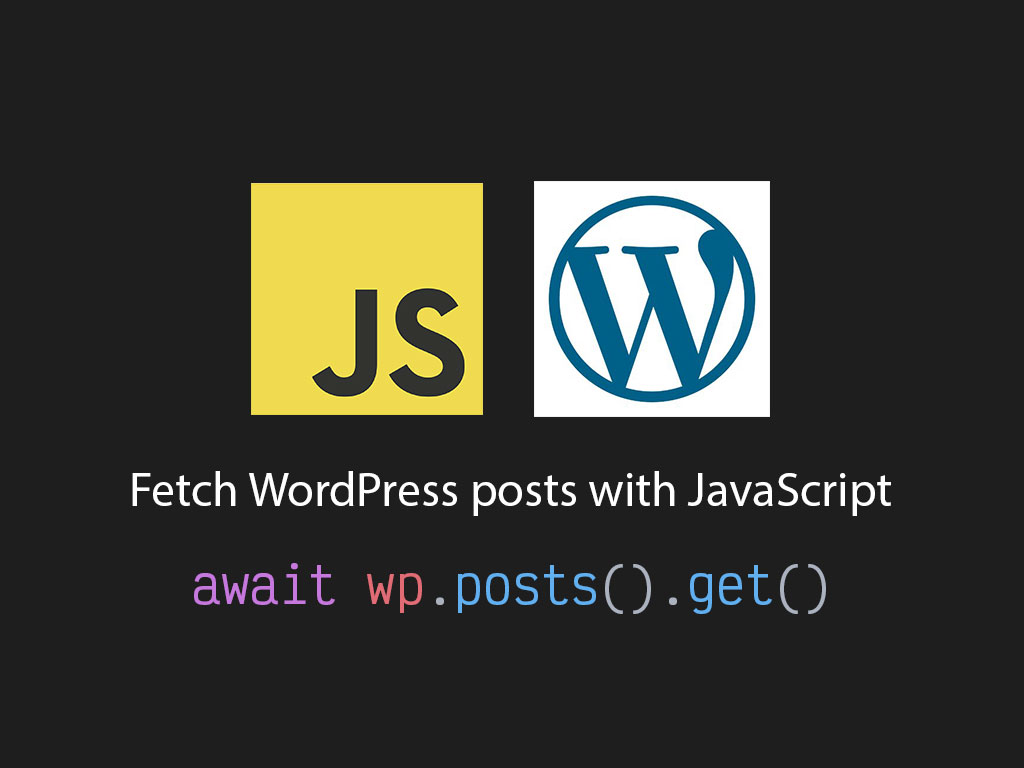
Are you trying to make a headless WordPress site and using JavaScript to fetch the data?
If so, this the article you’ve been looking for then!
First, if you’re looking to launch a WordPress site for your blog or business, you might want to look into launching your blog with Bluehost for just $3.95/mo (49.43% off). They make it really easy to select an affordable plan, and create or transfer a domain.
Get started with Bluehost.
Disclaimer: The two Bluehost links above are affiliate links which provide a small commission to me at no cost to you. These links track your purchase and credit it to this website. Affiliate links are a primary way that I make money from this blog and Bluehost is the best web hosting option for new bloggers.
If you’re looking for other options, check out this article, “Cheap hosting for WordPress blogs“.
Let’s dive into making a WordPress site headless.
WordPress is already headless
Out of the box, WordPress already provides a robust rest API.
You can visit the URL below to test that your your WordPress site rest API is available.
https://<replace-with-domain>/wp-json/
In this guide, I’m going to fetch the latest posts on a WordPress site. Here’s the endpoint that I will be requesting to return all the posts.
https://<replace-with-domain>/wp-json/wp/v2/posts
There’s 1 WordPress plugin I highly suggest you install. And that is called, Better REST API Featured Images.
Better REST API Featured Images will extend the Rest API posts endpoint and add the featured image urls.
This is helpful when you want to display your feature image on your JavaScript application or any other headless client app you’re building.
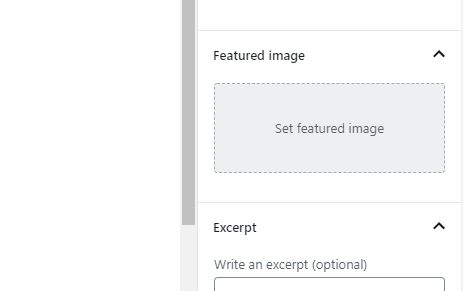
Here’s what the response from the plugin looks like.
"better_featured_image": {
"id": 3118,
"alt_text": "",
"caption": "",
"description": "",
"media_type": "image",
"media_details": {
"width": 1024,
"height": 768,
"file": "2020/06/js-wp-fetch-posts.jpg",
"sizes": {
"medium": {
"file": "js-wp-fetch-posts-300x225.jpg",
"width": 300,
"height": 225,
"mime-type": "image/jpeg",
"source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01121222/js-wp-fetch-posts-300x225.jpg"
},
"thumbnail": {
"file": "js-wp-fetch-posts-150x150.jpg",
"width": 150,
"height": 150,
"mime-type": "image/jpeg",
"source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01121222/js-wp-fetch-posts-150x150.jpg"
},
"medium_large": {
"file": "js-wp-fetch-posts-768x576.jpg",
"width": 768,
"height": 576,
"mime-type": "image/jpeg",
"source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01121222/js-wp-fetch-posts-768x576.jpg"
}
},
"image_meta": {
"aperture": "0",
"credit": "",
"camera": "",
"caption": "",
"created_timestamp": "0",
"copyright": "",
"focal_length": "0",
"iso": "0",
"shutter_speed": "0",
"title": "",
"orientation": "0",
"keywords": []
}
},
"post": 3084,
"source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01121222/js-wp-fetch-posts.jpg"
}
If the post doesn’t have a featured image. The value will be null.
"better_featured_image": null
Now that all of that is setup, it’s time to get all the posts from your WordPress rest API.
Fetch WordPress posts with JavaScript
One way to fetch the posts with JavaScript is to make a request directly to the posts API endpoint.
async function fetchPosts() {
try {
const response = await fetch('https://<replace-with-domain>/wp-json/wp/v2/posts');
console.log(response);
} catch(e) {
console.log(e)
console.log(e);
}
}
But that’s not a very efficient way to deal with the WordPress API.
Instead, install an NPM module called wpapi.
npm i -S wpapi
Here’s the JavaScript code to fetch the latest posts from your headless WordPress site with wpapi.
import WPAPI from 'wpapi';
// Create WPAPI instance and add endpoint to /wp-json
const wp = new WPAPI({
endpoint: 'http://<replace-with-domain>/wp-json',
});
async function fetchPosts() {
try {
// Fetch posts
const posts = await wp.posts().get();
return posts;
} catch (e) {
// print error
console.log(e);
return [];
}
}
The function above will either return an array of posts or an empty array if it fails.
It will print the error message out, in case you run into an error.
By default WordPress will return your latest 10 posts. If you want to fetch more than 10, then you may do so by using the perPage()
chaining tool.
const posts = await wp.posts().perPage(20).get();
Here’s a sample WordPress response object:
[
{
"id": 3084,
"date": "2020-06-01T12:26:38",
"date_gmt": "2020-06-01T12:26:38",
"modified": "2020-06-01T12:32:02",
"modified_gmt": "2020-06-01T12:32:02",
"slug": "how-to-make-wordpress-headless-and-fetch-posts-with-javascript",
"status": "publish",
"type": "post",
"title": {
"rendered": "How to make WordPress headless and fetch posts with JavaScript"
},
"content": {
"rendered": "\n<p>Are you trying to make a headless WordPress site and using JavaScript to fetch the data?</p>\n\n\n\n<p>If so, this the article you’ve been looking for then!</p>\n\n\n\n<p>First, if you’re looking to launch a WordPress site for your blog or business, you might want to look into launching your blog with <a rel=\"noreferrer noopener\" href=\"/api/recommend/bluehost\" target=\"_blank\">Bluehost</a> for just $3.95/month. They make it really easy to select an affordable plan, and create or transfer a domain.</p>\n\n\n\n<p><strong>Get started with</strong> <a href=\"/api/recommend/bluehost\" target=\"_blank\" rel=\"noreferrer noopener\">Bluehost</a>.</p>\n\n\n\n<p><strong>Disclaimer:</strong> The two Bluehost links above are affiliate links which provide a small commission to me at no cost to you. These links track your purchase and credit it to this website. Affiliate links are a primary way that I make money from this blog and Bluehost is the best web hosting option for new bloggers.</p>\n\n\n\n<p>If you’re looking for other options, check out this article, “<a href=\"https://linguinecode.com/post/cheap-wordpress-hosting\">Cheap hosting for WordPress blogs</a>“.</p>\n\n\n\n<p>Let’s dive into making a WordPress site headless.</p>\n\n\n\n<h2>WordPress is already headless</h2>\n\n\n\n<p>Out of the box, WordPress already provides a robust rest API.</p>\n\n\n\n<p>You can visit the URL below to test that your your WordPress site rest API is available.</p>\n\n\n\n<pre><code rel=\"WordPress JSON endpoint\" class=\"language-text\">\nhttps://<replace-with-domain>/wp-json/\n</code></pre>\n\n\n\n<p>In this guide, I’m going to fetch the latest posts on a WordPress site. Here’s the endpoint that I will be requesting to return all the posts.</p>\n\n\n\n<p><code class=\"language-text\">https://<replace-with-domain>/wp-json/wp/v2/posts</code></p>\n\n\n\n<p>There’s 1 WordPress plugin I highly suggest you install. And that is called, <a rel=\"noreferrer noopener\" href=\"https://wordpress.org/plugins/better-rest-api-featured-images/\" target=\"_blank\">Better REST API Featured Images</a>.</p>\n\n\n\n<p>Better REST API Featured Images will extend the Rest API posts endpoint and add the featured image urls.</p>\n\n\n\n<p>This is helpful when you want to display your feature image on your JavaScript application or any other headless client app you’re building.</p>\n\n\n\n<figure class=\"wp-block-image size-large\"><img src=\"https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01111135/feature-img-field.jpg\" alt=\"\" class=\"wp-image-3092\" srcset=\"https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01111135/feature-img-field.jpg 463w, https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/06/01111135/feature-img-field-300x189.jpg 300w\" sizes=\"(max-width: 463px) 100vw, 463px\" /></figure>\n\n\n\n<p>Here’s what the response from the plugin looks like.</p>\n\n\n\n<pre><code rel=\"Better REST API Featured Images response\" class=\"language-json\">\n"better_featured_image": {\n "id": 3083,\n "alt_text": "",\n "caption": "",\n "description": "",\n "media_type": "image",\n "media_details": {\n "width": 1024,\n "height": 768,\n "file": "2020/05/react-grid-layout-component.jpg",\n "sizes": {\n "medium": {\n "file": "react-grid-layout-component-300x225.jpg",\n "width": 300,\n "height": 225,\n "mime-type": "image/jpeg",\n "source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/05/30120740/react-grid-layout-component-300x225.jpg"\n },\n "thumbnail": {\n "file": "react-grid-layout-component-150x150.jpg",\n "width": 150,\n "height": 150,\n "mime-type": "image/jpeg",\n "source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/05/30120740/react-grid-layout-component-150x150.jpg"\n },\n "medium_large": {\n "file": "react-grid-layout-component-768x576.jpg",\n "width": 768,\n "height": 576,\n "mime-type": "image/jpeg",\n "source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/05/30120740/react-grid-layout-component-768x576.jpg"\n }\n },\n "image_meta": {\n "aperture": "0",\n "credit": "",\n "camera": "",\n "caption": "",\n "created_timestamp": "0",\n "copyright": "",\n "focal_length": "0",\n "iso": "0",\n "shutter_speed": "0",\n "title": "",\n "orientation": "0",\n "keywords": []\n }\n },\n "post": 3063,\n "source_url": "https://daqxzxzy8xq3u.cloudfront.net/wp-content/uploads/2020/05/30120740/react-grid-layout-component.jpg"\n}\n</code></pre>\n\n\n\n<p>If the post doesn’t have a featured image. The value will be <a href=\"https://linguinecode.com/guides/javascript/beginners/types-intro#null\">null</a>.</p>\n\n\n\n<pre><code rel=\"Better REST API Featured Images empty response\" class=\"language-json\">\n"better_featured_image": null\n</code></pre>\n\n\n\n<p>Now that all of that is setup, it’s time to get all the posts from your WordPress rest API.</p>\n\n\n\n<h2>Fetch WordPress posts with JavaScript</h2>\n\n\n\n<p>One way to fetch the posts with JavaScript is to make a request directly to the posts API endpoint.</p>\n\n\n\n<pre><code rel=\"Fetch WP posts with JavaScript\" class=\"language-javascript\">\nasync function fetchPosts() {\n try {\n const response = await fetch('https://<replace-with-domain>/wp-json/wp/v2/posts');\n\n console.log(response);\n } catch(e) {\n console.log(e)\n console.log(e);\n }\n}\n</code></pre>\n\n\n\n<p>But that’s not a very efficient way to deal with the WordPress API.</p>\n\n\n\n<p>Instead, install an NPM module called <a rel=\"noreferrer noopener\" href=\"https://www.npmjs.com/package/wpapi\" target=\"_blank\">wpapi</a>.</p>\n\n\n\n<pre><code rel=\"Terminal\" class=\"language-text\">\nnpm i -S wpapi\n</code></pre>\n\n\n\n<p>Here’s the JavaScript code to fetch the latest posts from your headless WordPress site with wpapi.</p>\n\n\n\n<pre><code rel=\"Fetch WP posts\" class=\"language-javascript\">\nimport WPAPI from 'wpapi';\n\n// Create WPAPI instance and add endpoint to /wp-json\nconst wp = new WPAPI({\n endpoint: 'http://<replace-with-domain>/wp-json',\n});\n\nasync function fetchPosts() {\n try {\n // Fetch posts\n const posts = await wp.posts().get();\n return posts;\n } catch (e) {\n // print error\n console.log(e);\n return [];\n }\n}\n</code></pre>\n\n\n\n<p>The function above will either return an array of posts or an empty array if it fails.</p>\n\n\n\n<p>It will print the error message out, in case you run into an error.</p>\n\n\n\n<p>By default WordPress will return your latest 10 posts. If you want to fetch more than 10, then you may do so by using the <code class=\"language-text\">perPage()</code> chaining tool.</p>\n\n\n\n<pre><code rel=\"Set amount of posts per page\" class=\"language-javascript\">\nconst posts = await wp.posts().perPage(20).get();\n</code></pre>\n\n\n\n<p>And that’s how you fetch posts from WordPress with JavaScript!</p>\n",
},
"excerpt": {
"rendered": "<p>Use the module wpapi to return all posts or a specific number from WordPress rest API v2.</p>\n",
},
"author": 1,
"featured_media": 3118,
"comment_status": "open",
"ping_status": "open",
"sticky": false,
"template": "",
"format": "standard",
"meta": [],
"categories": [
2
],
"tags": [],
"better_featured_image": {
// ... better featured imags response
}
},
// ... many more posts
]
And that’s how you fetch posts from WordPress with JavaScript!
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about JavaScript and post helpful code snippets. Follow me there if you would like some too!