How Svelte onMount works
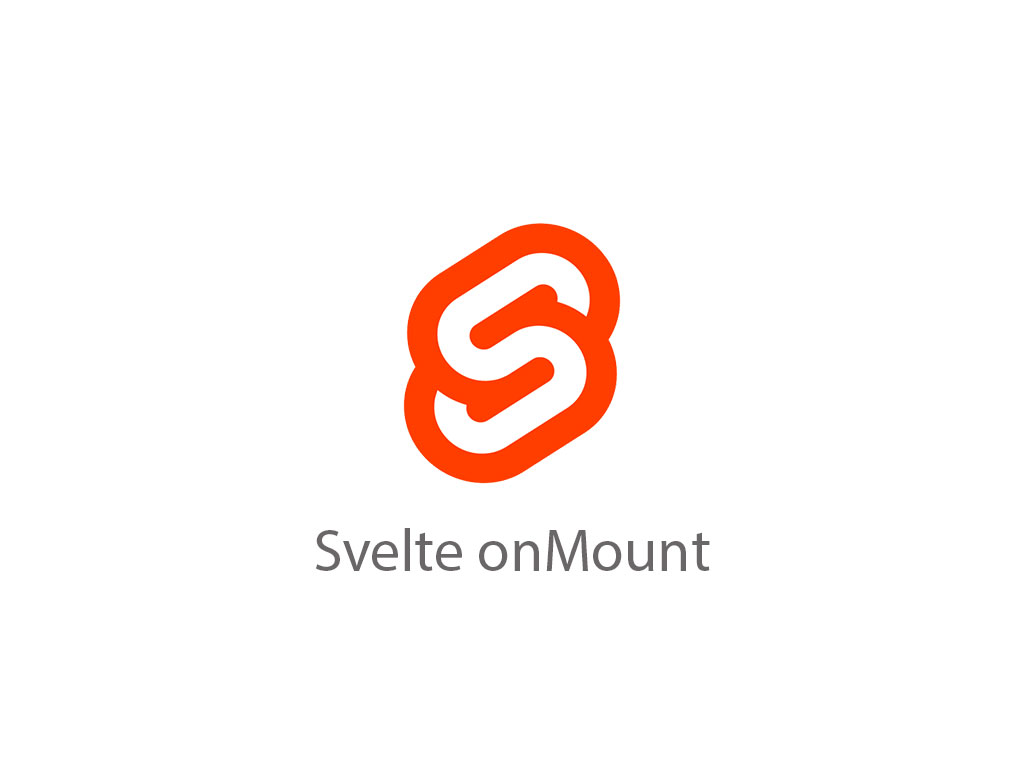
If you’re coming from a React or even an Angular world , you’re use to fetching initial data or updating React state on the componentDidMount
lifecycle.
So, what does this look like in Svelte?
Svelte has the mechanism as React. Every Svelte component goes through a phase of lifecycles, and onMount
is one of those lifecycles.
What is Svelte onMount
This lifecycle comes right after the initial render of a Svelte component.
<script>
import { onMount } from 'svelte';
onMount(() => {
// ... Run something after component has mounted
console.log('App component has mounted');
});
console.log('render');
</script>
Within a <script>
tag you must import the onMount
function from the svelte package.
onMount(callback)
onMount
accepts a callback function. This callback function gets executed once the component has mounted on to the application.
The output should look something like this.
render
App component has mounted
When to use Svelte onMount?
That’s actually a great question.
Data fetching
Just like React componentDidMount
, this is a great place to data fetch lazily.
<script>
import { onMount } from 'svelte';
// Array of photos
let photos = [];
onMount(async () => {
const res = await fetch(`https://jsonplaceholder.typicode.com/photos?_limit=20`);
// Update state variable, photos
photos = [...await res.json()];
});
</script>
The callback function for onMount
supports async/await
.
This comes in handy when you’re doing SSR applications with Svelte.
Do we need to use Svelte onMount hook
If you ever pay close attention to your Svelte component. It never re-renders the entire component like React does.
<script>
let number = 0;
const handleClick = () => number += 1;
console.log('render');
</script>
<main>
<h1>{number}</h1>
<button on:click="{handleClick}">Increment</button>
</main>
<style>
main {
text-align: center;
padding: 1em;
max-width: 750px;
margin: 0 auto;
}
h1 {
color: purple;
font-family: 'Comic Sans MS', cursive;
font-size: 2em;
display: block;
text-align: center;
}
</style>
Updating the number
variable will not cause the Svelte component to re-run like how it would happen within a React render()
method.
So, console.log('render')
will only trigger once.
So this begs to question.
Do we even need to use the onMount()
?
According to the creator, Rich Harris, no but you should for good habit practice.
And it seems to be good practice if Svelte ever decides to bring in a React Suspense API style into Svelte.
I like to tweet about Svelte and post helpful code snippets. Follow me there if you would like some too!