How to add environment variables to your Svelte JS app
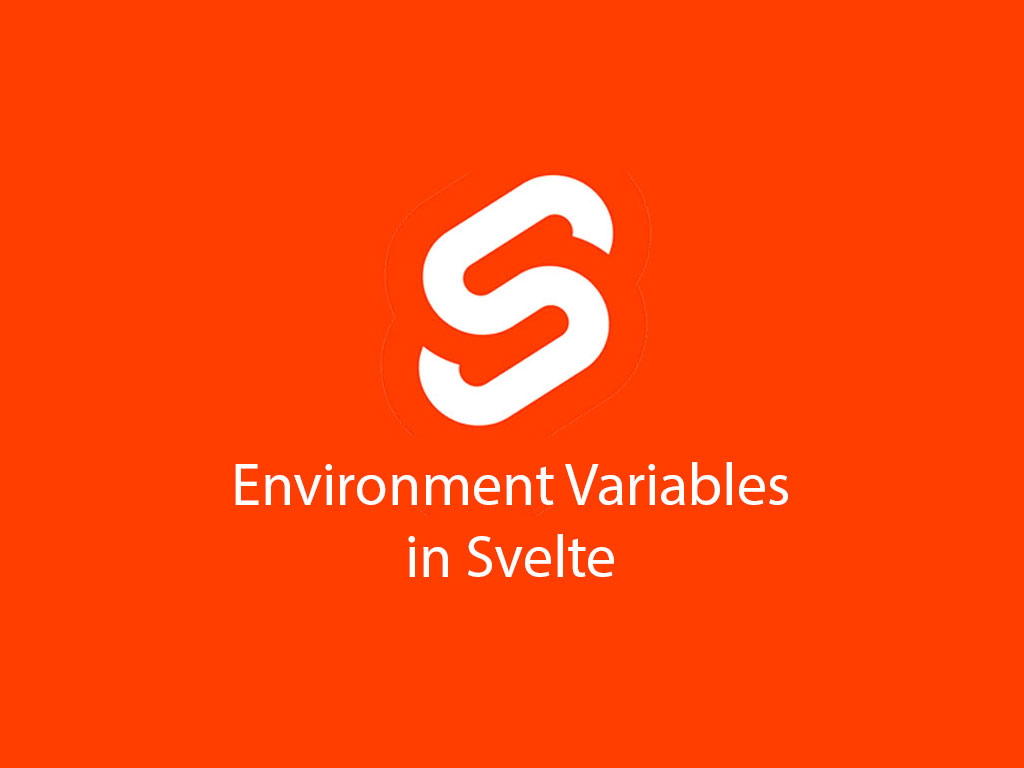
99% of the time, applications are going to need some unique configuration values that are very environment specific. How do we do this with a Svelte application?
You can use a plugin called @rollup/plugin-replace to pass environment variables down to your Svelte application. Let’s go over how to do this!
Use @rollup/plugin-replace to add environment variables
This plugin is more of a emulation of dotenv
. This plugin finds the defined properties, and replaces it with the value that it was given during compilation time.
Let’s go step by step how to use this module.
Step 1: Install plugin-replace module
npm install --save-dev @rollup/plugin-replace
Step 2: Update rollup.config.js
Go into the rollup.config.js file, and import the plugin replace module.
import replace from '@rollup/plugin-replace';
const production = !process.env.ROLLUP_WATCH;
export default {
plugins: [
replace({
FOO: 'bar',
// 2 level deep object should be stringify
process: JSON.stringify({
env: {
isProd: production,
}
}),
}),
],
};
Add the replace()
inside the plugin property.
The function replace()
accepts an object. Any deeper than 1 level objects need to be JSON.stringify()
.
Step 3: Access env variable in Svelte component
You can access the environment variable as if it was a global variable inside your Svelte component.
<script>
const mssg = process.env.isProd ? 'This is production mode' : FOO;
</script>
<h1>{mssg}</h1>
In the example above I’m creating a variable that uses the environment variables to determine what string output it should print to the user.
Oh wow, you’ve made it this far! If you enjoyed this article perhaps like or retweet the thread on Twitter:
I like to tweet about Svelte and post helpful code snippets. Follow me there if you would like some too!