How to add routing to Svelte SPA with Routify
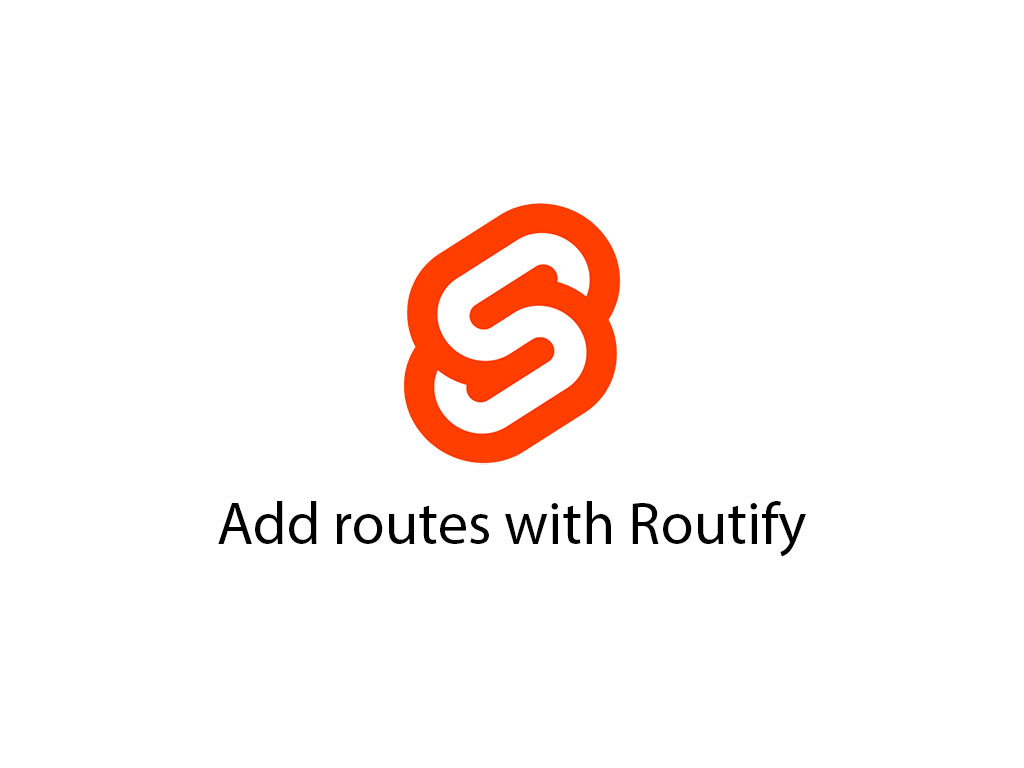
Are you building a SPA (single page application) with Svelte?
If so, you’re going to need a routing system. In this article I’ll be using a Svelte tool called Routify.
What is Routify?
Routify is a Svelte package that lets you create routes, based on a filesystem-based routing.
For example, if you create index.svelte, this would be equivalent to /
.
If you create about.svelte, this would be equivalent to /about
.
Why use Routify?
There’s usually 2 methods to building routes, a configuration style or a file structure type.
Personally, I’ve worked with large routing configurations, and that ain’t no fun.
Filesystem-based routing is simple, and it’s easier to debug. Tools such as Sapper, and Next.JS use this type of method.
And it’s proven to be reliable, and easy for development.
Adding Routify routing to your Svelte application
Step 1: Install Routify
This is a simple step. You can install the module via NPM.
npm i --save-dev @sveltech/routify@next npm-run-all
You will also be install another module called npm-run-all
.
npm-run-all
is a neat module to run multiple npm scripts in parallel.
Step 2: Update package.json file
Now that you’ve installed the 2 NPM modules above, you must update your package.json file and add the following NPM scripts.
"scripts": {
"dev": "run-p watch:routify watch:rollup",
"watch:rollup": "rollup -c -w",
"watch:routify": "routify"
}
When you run your dev environment, make sure to use npm run dev
.
Step 3: Update your App.svelte file
The next step is to import the Router
Svelte component and the routes configuration.
Those can be found within the @sveltech/routify
module.
<script>
import { Router } from '@sveltech/routify';
import { routes } from '@sveltech/routify/tmp/routes';
</script>
<Router {routes} />
That’s it!
The heavy lifting is done now. You can get started, and create your pages.
Step 4: Create your pages
Make sure to create a directory called pages
in the same directory level where the App.svelte file is.
- Svelte project
- src
- pages
- index.svelte
- about.svelte
- App.svelte
Like mentioned above, whatever you name your file, that will be the URL path name.
How to add dynamic routing
Sometimes your app will need some dynamic data in the URL, and you’ll want to make it a pretty URL as well.
You want to go from /post?id=31
to /post/31
.
To achieve this level of dynamic routing, and pretty URL’s you’ll need to name your page Svelte files as such – [post].svelte
The brackets ([]
) let Routify know that this will accept dynamic parameters.
Accessing the dynamic parameters
Accessing the route parameters is really simple also.
Import the params
helper tool, to get access to the current route parameters.
<!-- src/pages/post/[postId].svelte -->
<script>
import { params } from '@sveltech/routify';
export let postId;
console.log($params)
</script>
If you now visit the path /post/31
, for example. You should see the following print on your console.
{ postId: "31" }
How to navigate to another page
Navigating is another crucial key component to routing. This is how it’s done with Routify.
Start by importing the url()
helper function in your Svelte component.
And use the function inside the href
attribute of link tag element.
<script>
import { url } from '@sveltech/routify'
</script>
<!-- relative -->
<a href={$url('../')}>Home page</a>
<!-- absolute -->
<a href={$url('/')}>Home page</a>
<!-- params -->
<a href={$url('/post/:id', {id: '31'})}>Post 31</a>
You can do relative, and absolute paths.
You may also pass unique parameter values if you pass a second argument to the url()
function.
I like to tweet about Svelte and post helpful code snippets. Follow me there if you would like some too!