How To Import Local Files In Golang In 4 Easy Steps
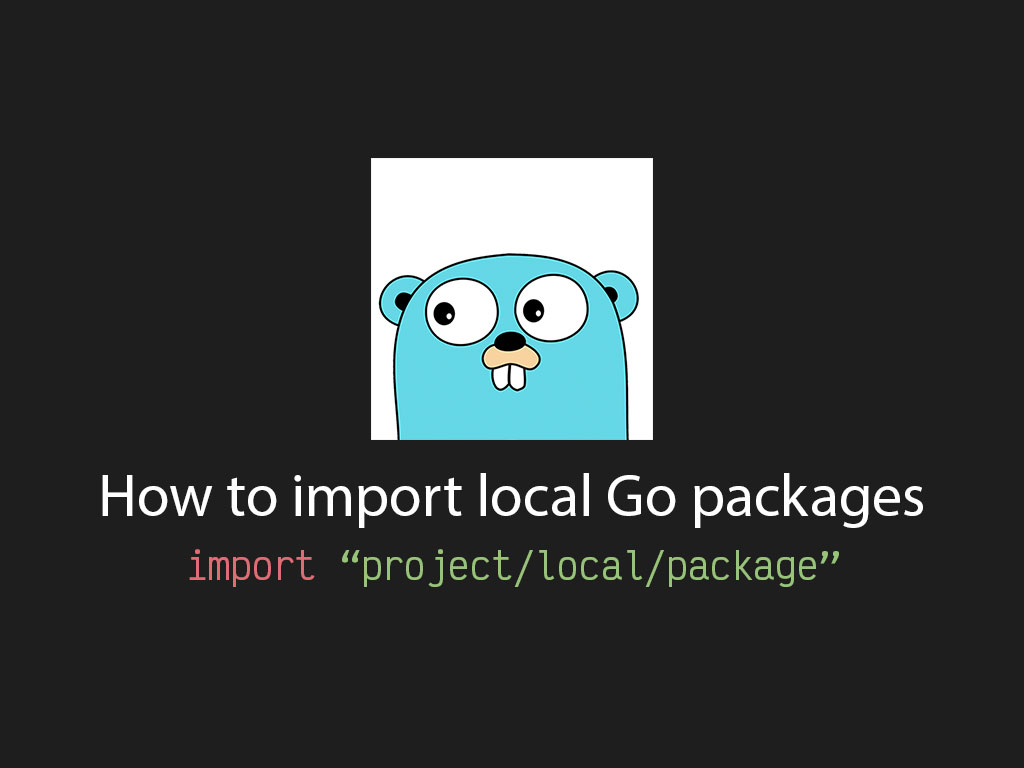
Coming from a PHP and JavaScript world myself, importing local files is really easy.
But jumping into Golang, I struggled for hours trying to figure out how to import a local file and finding good resources that explain how it works was another pain point.
This article will cover everything you need to know about how to import a local Go files into your project.
Before I go through the step-by-step process, you need to understand what a Go package means. If you already know feel free to jump to step 1. Click here to jump.
What is a Go package?
A Go package also known as a “workspace”, is just a directory in your project.
-src
--foobar.com/
---main.go
---woosah/
----init.go
---bar/
----a.go
Let’s say the project directory is called foobar.com. Inside my project I have 2 more directories called woosah and bar.
In Golang, a directory is known as a package. Every file inside that directory is automatically assigned to that directory aka a Go package.
The root of a project is always known as the main package
. So the file main.go in the example above is assigned to the main package
.
package main
In the example, init.go is assigned to the woosah Go package
.
package woosah
And the file a.go, is assigned to the bar Go package
.
package woosah
Every exported code inside a Go package is automatically accessible when importing.
That means you don’t have to import single files. You just import the package and you have access to the all the exported code within that package.
To recap, a directory inside a project is known as a Go package.
In the example above I defined the package name in the Go file the same as the directory name. It’s common practice to do that, but you don’t have to.
You can have a directory called utils, but the files inside can use a package name called silly_goose
.
In a directory you cannot have multiple package names in Go. Create a new directory for different packages.
From here on out, I will be referring a directory as a Go package.
If you haven’t installed Go and setup your $GOPATH
, please read this guide before proceeding. “How to install Golang in 5 easy steps“.
Step 1: Initialize a new module in your project
The first step is to initialize a new module in your Go project. In your terminal go the root of your project and enter the following command:
go mod init <module_name>
When you initialize a new module in your project directory it will generate a new file called go.mod.
module <module_name>
go 1.15
What is a go.mod file?
A go.mod file is like the package.json file for your Go application.
The go.mod file contains information like the module name of your project, the version of Go you’re using, and a list of third-party Go modules.
How to name your Go module
When you’re initializing your Go module, it’s best practice to give your module name the domain of your site.
For example, if my Go project is going to be serving linguinecode.com than module name should be <linguinecode.com>
go mod init linguinecode.com
Another example is if my project is just to showcase in my Github portfolio, and the Github project name is called go-test-project then my command should look like this:
go mod init github.com/rleija/go-test-project
The Go module name is important because it will get used when trying to access your local Go packages.
Step 2: Create a Go package in your project
The next step is to create a new Go package in your project.
Will follow a similar structure from the example above.
-src
--github.com/rleija/go-test-project
---main.go
---woosah/
----init.go
Create a directory called woosah in your Go project.
mkdir ./woosah
Step 3: Create a file in your new Go package
Now that you’ve created your Go package, create a Go file inside your Go package. I’ll call mine init.go
In your new file, the first line of code must let Go know to what the Go package namespace will be. I will use the the directory name as the Go package, woosah.
package woosah
Now let’s add some code that we can export from the new Go file.
To export variables and functions in Golang you have to make sure the first letter of the identifier name for a variable or function is capitalized.
package woosah
// Private visibility
var test1 = "private variable"
// Public visibility
var Test2 = "public variable"
// Private visiblity
func greet() string {
return "hello"
}
// Public visibility
func Greet2() string {
return "hello world"
}
In the example above the variable test1
and the function greet()
are private because the first letter of the identifier name isn’t capitalized.
If you try to use them Go will print out an error message
// cannot refer to unexported name woosah.test1
fmt.Println(woosah.test1)
// public variable
fmt.Println(woosah.Test2)
The variable Test2
and the function Greet2()
are considered exported because the first letter in the identifier name are capitalized.
Now that you understand how to export variables and functions from a Go file, it’s time to import them from another Go file and use that exported code.
Step 4: Import your local Go package aka local file
In my main.go file found in the root of the project, I’m going to import the woosah package
and print to the terminal the greet message.
package main
import (
"fmt"
"github.com/rleija/go-test-project/woosah"
)
func main() {
fmt.Println(woosah.Greet2())
}
When you’re using import
, you want to start with your project module name and point to the directory where you Go package lives.
To recap about exported code, any file created under the Go package will be accessible at all time.
This means that if you create 10 different files inside the woosah package
name, all exported code in each of those files will be accessible when you import your package.
I like to tweet about Golang and post helpful code snippets. Follow me there if you would like some too!