How to pass values to onClick React function
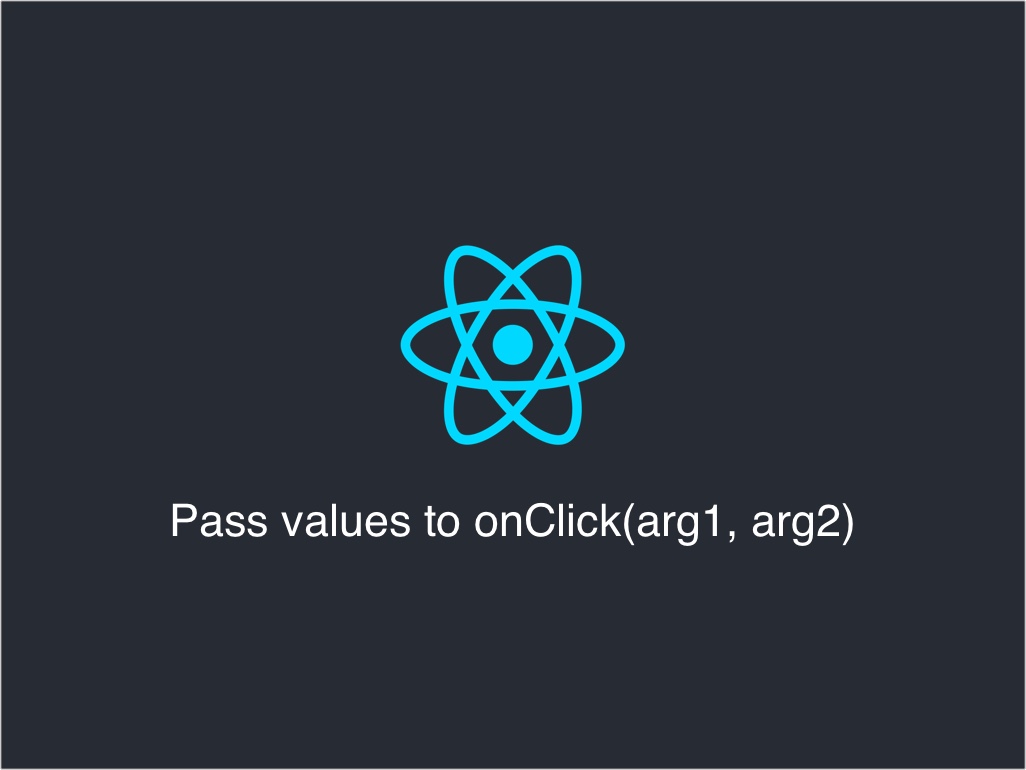
Sometimes passing an additional parameter values or arguments is necessary for you onClick
handler.
Solutions
There’s a couple approaches we can take a look at.
Method #1: Return a function that returns a function
You can create a function that returns a function.
class FooBar extends React.Component {
handleClick = value => () => {
console.log(value);
};
render() {
return <button onClick={this.handleClick('Bar')}>Speak</button>;
}
}
In the example above, I’m defining a new property called, handleClick()
.
handleClick
is a function, that accepts 1 argument called value.
And when you invoke it once, it will return another function which can be used for the onClick
React listener.
In the example above you can see that I’m attaching the handleClick()
method, and passing a string value so it may be printed in the browser console.
<button onClick={this.handleClick('Bar')}>Speak</button>
This can also be written as such:
class FooBar extends React.Component {
handleClick(value) {
return function() {
console.log(value)
}
};
render() {
return <button onClick={this.handleClick('Bar')}>Speak</button>;
}
}
If you’re writing a functional component, than it may look like this:
const handleClick = value => () => console.log(value)
const FooBar = () => <button onClick={handleClick('Bar')}>Speak</button>;
Which I believe looks a bit cleaner.
This method of passing values to an onClick
handler is by far the most common, and easiest to follow approach.
Method #2: Add custom data attributes
This method is 100% valid and an interesting one.
What you have to do is add a custom data-attribute
to your HTML element.
const handleClick = event => console.log(event.target.dataset) // {mssg: "Hello!"}
const FooBar = () => <button data-mssg="Hello!" onClick={handleClick}>Speak</button>;
The onClick
listener returns the event
object. You can go through the object and check out the dataset property to retrieve your custom data attribute.
Method #3: Set an onClick event in a sub component
You can also create a React component that accepts 2 properties.
The first property is props.value
which it may any primitive value or object.
const Button = props => {
const handleClick = () => {
if (props.onClick) {
props.onClick(props.value);
}
}
return <button onClick={handleClick}>Speak</button>;
}
The second property is called props.onClick
. This custom prop will return the value that gets given to the component.
Inside the Button
component, I’m creating a variable called handleClick
. This variable will check if the custom props.onClick
is given, and if it is will invoke it and pass the props.value
value.
Now Let’s see how to use this in the parent component.
const handleClick = value => console.log(value);
const FooBar = () => <Button value="WOOSAH" onClick={handleClick} />;
In the parent component, FooBar
, I’m using the Button
component and giving it a value of WOOSAH, and passing the handler function for the onClick
event.
In the console we should see the output
WOOSAH
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!