How to redirect on the server side with Next.JS
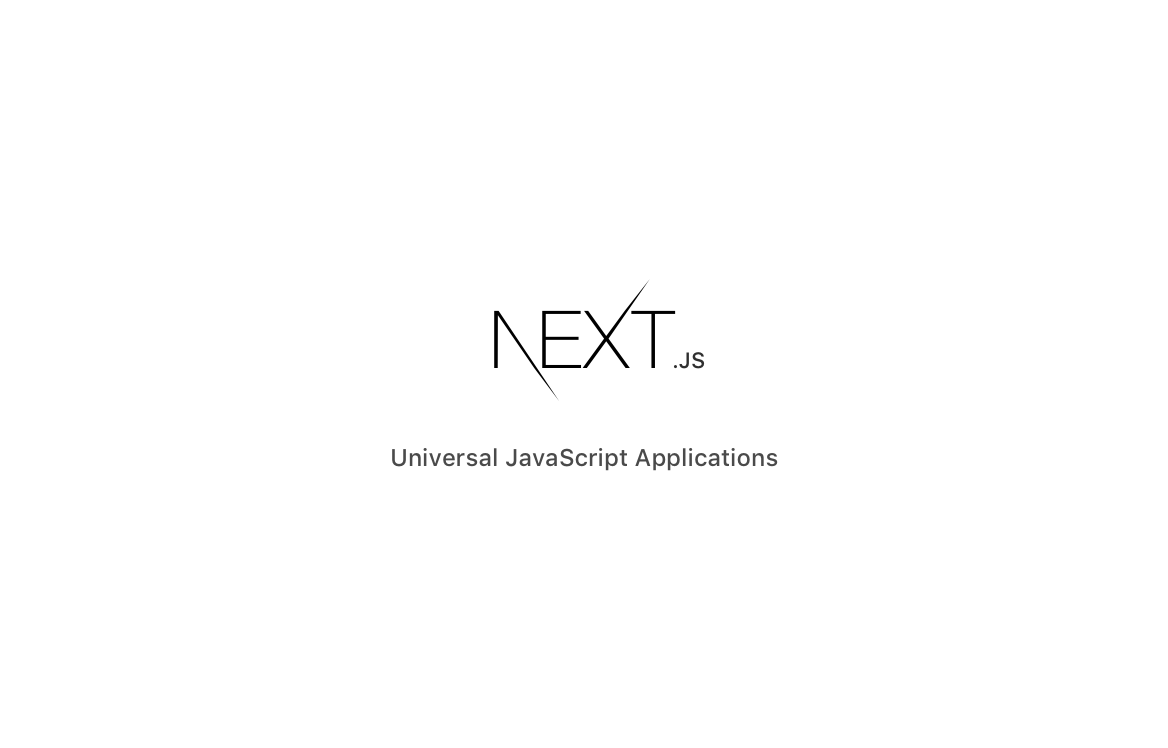
Maybe your blog domain has changed, and you need to redirect the user to the new URL.
Or maybe, the user is not authenticated and it needs to get redirected before any React work gets executed.
How do you accomplish this redirect on the server-side with Next.JS?
Solution #1: getInitialProps()
In a recent article, I discussed the benefits of getInitialProps(). You can read up on it here, “How to access getInitialProps with a class or function component in NextJS“.
To do a quick recap, getInitialProps() is a function/method that lets you write server code before the page component in Next.JS gets rendered.
In that function/method you can redirect a user to an internal or external URL via the server side.
Let’s take a quick look at a code snippet to see how this is done programatically.
Home.getInitialProps = ({ res }) => {
if (res) {
res.writeHead(301, {
Location: 'new/url/destination/here'
});
res.end();
}
return {};
};
In the example above, I’m accessing getInitialProps() with the functional component type.
I’m grabbing the res
object that Next.JS provides for us so I may redirect the user somewhere else.
res
is only available on the server side.
res
comes with a method called, res.writeHead()
.
When using res.writeHead()
, I’m telling my server-side of the application to send some new header information.
The first piece of information is that the status code will be a 301 (permanently moved). This status code can be any of the HTTP status codes that best suits your use case.
The second piece of information I’m modifying is the Location value. This is where you’re going to place the URL you want to redirect a user to.
To finish it off, I’m ending with a res.end()
to let the application know to execute the changes.
Redirect a user via Zeit Now 2 configuration
If you’re using Zeit hosting platform, Now, you can also do this through their now.json configuration.
{
"routes": [
{
"src": "/kitties",
"status": 301,
"headers": {
"Location": "https://linguinecode.com/"
}
}
]
}
In the now configuration file, you’ll need to add a routes
property.
In the example above I’m letting Zeit know to check for a specific pathname in my site, /kitties.
If it matches, it will perform a 301 redirect to my homepage.
I like to tweet about NextJS and post helpful code snippets. Follow me there if you would like some too!