How to render a function with HTML in Svelte
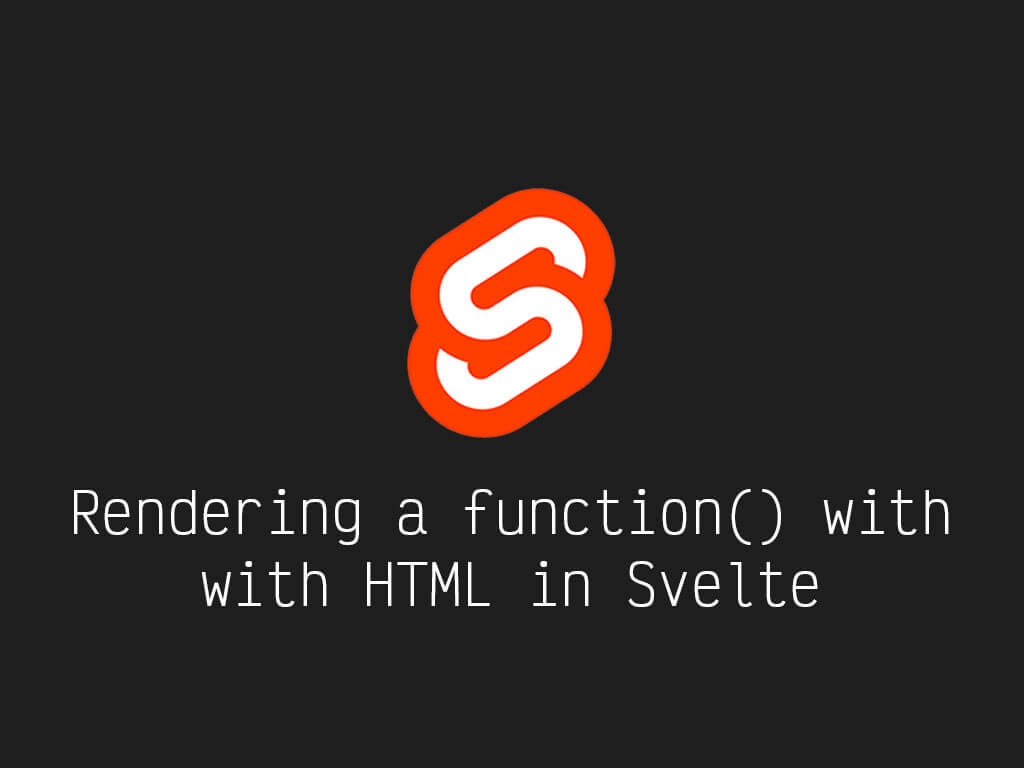
How do you call a custom function with HTML code, and render it in Svelte?
For example:
<script>
const renderGreet = mssg => `<h1>${mssg}</h1>`;
</script>
{renderGreet("Hello World!")}
Note that in my renderGreet()
function I’m wrapping my html code with backticks (``
).
If you don’t do that your Svelte application will break. This isn’t JSX.
Another thing to note is that strings in Svelte are inserted as just plain text. Special characters like brackets (<>
) have no special meaning.
The code example above would render as such
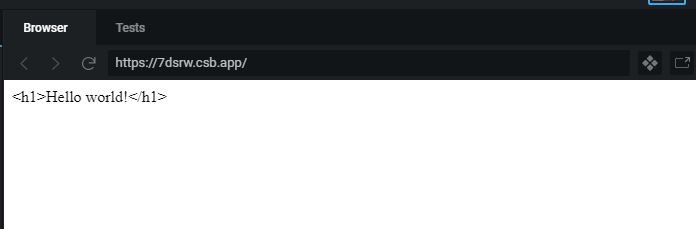
So how do we solve for this?
Solution: Use @html tags expressions
{@html expression}
expressions allows Svelte to make those special characters have meaning.
<script>
const renderGreet = mssg => `<h1>${mssg}</h1>`;
</script>
{@html renderGreet("Hello World!")}
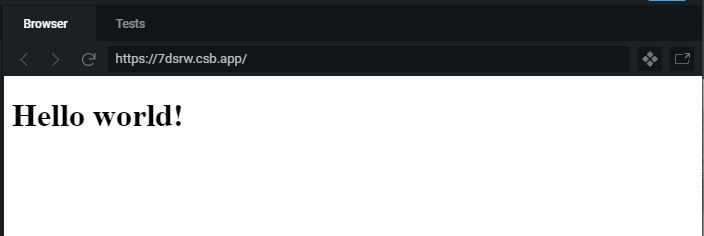
When you’re using this this expression type, you have to make sure
It’s valid HTML
{@html ''}Hello World{@html ''}
Doing something like this may render “Hello World,” but it will not generate the HTML as you would expect.
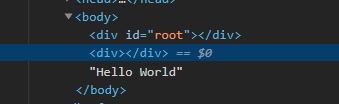
Sanitize before injecting
If you’re making an API to an untrusted source that returns HTML content, make sure to sanitize.
That way you don’t expose your users to XSS (cross-site scripting) vulnerabilities.
Conclusion
I would only use this approach when I have no control of the HTML.
This is usually the case when you’re fetch data dynamically, and that API is returning HTML back.
But if you do have control of the HTML markup, I would extract that content into it’s own Svelte component file, and use <svelte:component /> directive
.
I like to tweet about Svelte and post helpful code snippets. Follow me there if you would like some too!