Get current element with onMouseOver or onMouseEnter in React
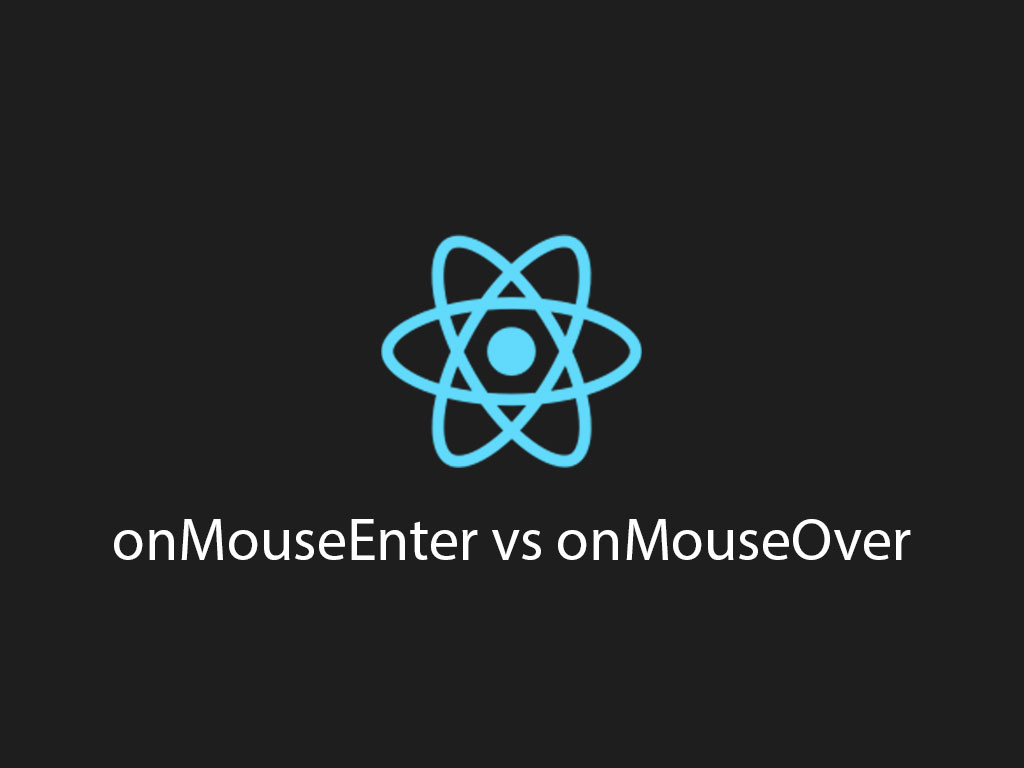
Are you trying to figure out how to get the current element when a user hovers over it?
Great! I want to go over a couple methods that you can approach to solve this problem.
Method #1: React useRef
I recommend using React useRef
if you’re targeting single elements.
Here’s an example of what that would look like:
const App = () => {
const boxRef = React.useRef(null);
const handleMouseEnter = () => {
console.log(boxRef);
};
return (
<div
style={{ width: 200, height: 200, backgroundColor: 'red' }}
ref={boxRef}
onMouseEnter={handleMouseEnter}>
Hover over me
</div>
);
};
If you’re interested in learning how to use React useRef
with TypeScript, check out this article, “How to use React useRef with TypeScript“.
But what if you have an array of elements? Do you create a React useRef hook
for each element the array?
Nope. Another appropriate method is to use the event
object that comes with the event listener.
Method #2: Event object
In this example I’m going to use the React onMouseOver
event.
This method may also apply to React onMouseEnter
as well.
const cats = [
{name: "Mr. Whiskers", color: 'black'},
{name: "Sassy", color: 'white'},
{name: "Smokey", color: 'gray'},
];
const App = () => {
const handleMouseOver = (event) => {
console.log(JSON.parse(event.target.dataset.info));
};
return (
<>
{cats.map((cat, i) => (
<div
key={i}
data-info={JSON.stringify(cat)}
style={{ width: 200, height: 200, backgroundColor: 'red', margin: 8, display: 'inline-block' }}
onMouseOver={handleMouseOver}>
{cat.name}
</div>
))}
</>
);
};
In the code example above, I’m going over an array of cat objects.
Each cat object has a name, and the color of the cat.
In the JSX section, I’m iterating over each object in the array, and embedding the cat information into a data attribute called data-info
.
The objective of this code example is to grab the current element that was hovered on, and console.log()
the data attribute that was added to the div
.
To achieve this, I attached an event handler called, handleMouseOver()
, onto the onMouseOver event
for each div
directive.
The event function handler provides us an object called event
.
I will use the event object and drill through the object tree to log the data attribute data-info
.
const handleMouseOver = (event) => {
console.log(JSON.parse(event.target.dataset.info));
};
The output looks like this:
Object {name: "Mr. Whiskers", color: "black"}
Object {name: "Sassy", color: "white"}
Object {name: "Smokey", color: "gray"}
I like to tweet about React and post helpful code snippets. Follow me there if you would like some too!